L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_hal_nand.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_nand.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of NAND HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_NAND_H 00040 #define __STM32L4xx_HAL_NAND_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_ll_fmc.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup NAND 00054 * @{ 00055 */ 00056 00057 /** @addtogroup NAND_Private_Constants 00058 * @{ 00059 */ 00060 00061 #define NAND_DEVICE FMC_BANK3 00062 #define NAND_WRITE_TIMEOUT ((uint32_t)1000) 00063 00064 #define CMD_AREA ((uint32_t)(1<<16)) /* A16 = CLE high */ 00065 #define ADDR_AREA ((uint32_t)(1<<17)) /* A17 = ALE high */ 00066 00067 #define NAND_CMD_AREA_A ((uint8_t)0x00) 00068 #define NAND_CMD_AREA_B ((uint8_t)0x01) 00069 #define NAND_CMD_AREA_C ((uint8_t)0x50) 00070 #define NAND_CMD_AREA_TRUE1 ((uint8_t)0x30) 00071 00072 #define NAND_CMD_WRITE0 ((uint8_t)0x80) 00073 #define NAND_CMD_WRITE_TRUE1 ((uint8_t)0x10) 00074 #define NAND_CMD_ERASE0 ((uint8_t)0x60) 00075 #define NAND_CMD_ERASE1 ((uint8_t)0xD0) 00076 #define NAND_CMD_READID ((uint8_t)0x90) 00077 #define NAND_CMD_STATUS ((uint8_t)0x70) 00078 #define NAND_CMD_LOCK_STATUS ((uint8_t)0x7A) 00079 #define NAND_CMD_RESET ((uint8_t)0xFF) 00080 00081 /* NAND memory status */ 00082 #define NAND_VALID_ADDRESS ((uint32_t)0x00000100) 00083 #define NAND_INVALID_ADDRESS ((uint32_t)0x00000200) 00084 #define NAND_TIMEOUT_ERROR ((uint32_t)0x00000400) 00085 #define NAND_BUSY ((uint32_t)0x00000000) 00086 #define NAND_ERROR ((uint32_t)0x00000001) 00087 #define NAND_READY ((uint32_t)0x00000040) 00088 00089 /** 00090 * @} 00091 */ 00092 00093 /** @addtogroup NAND_Private_Macros 00094 * @{ 00095 */ 00096 00097 /** 00098 * @brief NAND memory address computation. 00099 * @param __ADDRESS__: NAND memory address. 00100 * @param __HANDLE__: NAND handle. 00101 * @retval NAND Raw address value 00102 */ 00103 #define ARRAY_ADDRESS(__ADDRESS__ , __HANDLE__) (((__ADDRESS__)->Page) + \ 00104 (((__ADDRESS__)->Block + (((__ADDRESS__)->Zone) * ((__HANDLE__)->Info.ZoneSize)))* ((__HANDLE__)->Info.BlockSize * ((__HANDLE__)->Info.PageSize + (__HANDLE__)->Info.SpareAreaSize)))) 00105 00106 /** 00107 * @brief NAND memory address cycling. 00108 * @param __ADDRESS__: NAND memory address. 00109 * @retval NAND address cycling value. 00110 */ 00111 #define ADDR_1ST_CYCLE(__ADDRESS__) (uint8_t)(__ADDRESS__) /* 1st addressing cycle */ 00112 #define ADDR_2ND_CYCLE(__ADDRESS__) (uint8_t)((__ADDRESS__) >> 8) /* 2nd addressing cycle */ 00113 #define ADDR_3RD_CYCLE(__ADDRESS__) (uint8_t)((__ADDRESS__) >> 16) /* 3rd addressing cycle */ 00114 #define ADDR_4TH_CYCLE(__ADDRESS__) (uint8_t)((__ADDRESS__) >> 24) /* 4th addressing cycle */ 00115 00116 /** 00117 * @} 00118 */ 00119 00120 /* Exported typedef ----------------------------------------------------------*/ 00121 /* Exported types ------------------------------------------------------------*/ 00122 /** @defgroup NAND_Exported_Types NAND Exported Types 00123 * @{ 00124 */ 00125 00126 /** 00127 * @brief HAL NAND State structures definition 00128 */ 00129 typedef enum 00130 { 00131 HAL_NAND_STATE_RESET = 0x00, /*!< NAND not yet initialized or disabled */ 00132 HAL_NAND_STATE_READY = 0x01, /*!< NAND initialized and ready for use */ 00133 HAL_NAND_STATE_BUSY = 0x02, /*!< NAND internal process is ongoing */ 00134 HAL_NAND_STATE_ERROR = 0x03 /*!< NAND error state */ 00135 }HAL_NAND_StateTypeDef; 00136 00137 /** 00138 * @brief NAND Memory electronic signature Structure definition 00139 */ 00140 typedef struct 00141 { 00142 /*<! NAND memory electronic signature maker and device IDs */ 00143 00144 uint8_t Maker_Id; 00145 00146 uint8_t Device_Id; 00147 00148 uint8_t Third_Id; 00149 00150 uint8_t Fourth_Id; 00151 }NAND_IDTypeDef; 00152 00153 /** 00154 * @brief NAND Memory address Structure definition 00155 */ 00156 typedef struct 00157 { 00158 uint16_t Page; /*!< NAND memory Page address */ 00159 00160 uint16_t Zone; /*!< NAND memory Zone address */ 00161 00162 uint16_t Block; /*!< NAND memory Block address */ 00163 00164 }NAND_AddressTypeDef; 00165 00166 /** 00167 * @brief NAND Memory info Structure definition 00168 */ 00169 typedef struct 00170 { 00171 uint32_t PageSize; /*!< NAND memory page (without spare area) size measured in K. bytes */ 00172 00173 uint32_t SpareAreaSize; /*!< NAND memory spare area size measured in K. bytes */ 00174 00175 uint32_t BlockSize; /*!< NAND memory block size number of pages */ 00176 00177 uint32_t BlockNbr; /*!< NAND memory number of blocks */ 00178 00179 uint32_t ZoneSize; /*!< NAND memory zone size measured in number of blocks */ 00180 }NAND_InfoTypeDef; 00181 00182 /** 00183 * @brief NAND handle Structure definition 00184 */ 00185 typedef struct 00186 { 00187 FMC_NAND_TypeDef *Instance; /*!< Register base address */ 00188 00189 FMC_NAND_InitTypeDef Init; /*!< NAND device control configuration parameters */ 00190 00191 HAL_LockTypeDef Lock; /*!< NAND locking object */ 00192 00193 __IO HAL_NAND_StateTypeDef State; /*!< NAND device access state */ 00194 00195 NAND_InfoTypeDef Info; /*!< NAND characteristic information structure */ 00196 }NAND_HandleTypeDef; 00197 00198 /** 00199 * @} 00200 */ 00201 00202 /* Exported constants --------------------------------------------------------*/ 00203 /* Exported macro ------------------------------------------------------------*/ 00204 /** @defgroup NAND_Exported_Macros NAND Exported Macros 00205 * @{ 00206 */ 00207 00208 /** @brief Reset NAND handle state. 00209 * @param __HANDLE__: specifies the NAND handle. 00210 * @retval None 00211 */ 00212 #define __HAL_NAND_RESET_HANDLE_STATE(__HANDLE__) ((__HANDLE__)->State = HAL_NAND_STATE_RESET) 00213 00214 /** 00215 * @} 00216 */ 00217 00218 /* Exported functions --------------------------------------------------------*/ 00219 /** @addtogroup NAND_Exported_Functions NAND Exported Functions 00220 * @{ 00221 */ 00222 00223 /** @addtogroup NAND_Exported_Functions_Group1 Initialization and de-initialization functions 00224 * @{ 00225 */ 00226 00227 /* Initialization/de-initialization functions ********************************/ 00228 HAL_StatusTypeDef HAL_NAND_Init(NAND_HandleTypeDef *hnand, FMC_NAND_PCC_TimingTypeDef *ComSpace_Timing, FMC_NAND_PCC_TimingTypeDef *AttSpace_Timing); 00229 HAL_StatusTypeDef HAL_NAND_DeInit(NAND_HandleTypeDef *hnand); 00230 void HAL_NAND_MspInit(NAND_HandleTypeDef *hnand); 00231 void HAL_NAND_MspDeInit(NAND_HandleTypeDef *hnand); 00232 void HAL_NAND_IRQHandler(NAND_HandleTypeDef *hnand); 00233 void HAL_NAND_ITCallback(NAND_HandleTypeDef *hnand); 00234 00235 /** 00236 * @} 00237 */ 00238 00239 /** @addtogroup NAND_Exported_Functions_Group2 Input and Output functions 00240 * @{ 00241 */ 00242 00243 /* IO operation functions ****************************************************/ 00244 HAL_StatusTypeDef HAL_NAND_Read_ID(NAND_HandleTypeDef *hnand, NAND_IDTypeDef *pNAND_ID); 00245 HAL_StatusTypeDef HAL_NAND_Reset(NAND_HandleTypeDef *hnand); 00246 HAL_StatusTypeDef HAL_NAND_Read_Page(NAND_HandleTypeDef *hnand, NAND_AddressTypeDef *pAddress, uint8_t *pBuffer, uint32_t NumPageToRead); 00247 HAL_StatusTypeDef HAL_NAND_Write_Page(NAND_HandleTypeDef *hnand, NAND_AddressTypeDef *pAddress, uint8_t *pBuffer, uint32_t NumPageToWrite); 00248 HAL_StatusTypeDef HAL_NAND_Read_SpareArea(NAND_HandleTypeDef *hnand, NAND_AddressTypeDef *pAddress, uint8_t *pBuffer, uint32_t NumSpareAreaToRead); 00249 HAL_StatusTypeDef HAL_NAND_Write_SpareArea(NAND_HandleTypeDef *hnand, NAND_AddressTypeDef *pAddress, uint8_t *pBuffer, uint32_t NumSpareAreaTowrite); 00250 HAL_StatusTypeDef HAL_NAND_Erase_Block(NAND_HandleTypeDef *hnand, NAND_AddressTypeDef *pAddress); 00251 uint32_t HAL_NAND_Read_Status(NAND_HandleTypeDef *hnand); 00252 uint32_t HAL_NAND_Address_Inc(NAND_HandleTypeDef *hnand, NAND_AddressTypeDef *pAddress); 00253 00254 /** 00255 * @} 00256 */ 00257 00258 /** @addtogroup NAND_Exported_Functions_Group3 Peripheral Control functions 00259 * @{ 00260 */ 00261 00262 /* NAND Control functions ****************************************************/ 00263 HAL_StatusTypeDef HAL_NAND_ECC_Enable(NAND_HandleTypeDef *hnand); 00264 HAL_StatusTypeDef HAL_NAND_ECC_Disable(NAND_HandleTypeDef *hnand); 00265 HAL_StatusTypeDef HAL_NAND_GetECC(NAND_HandleTypeDef *hnand, uint32_t *ECCval, uint32_t Timeout); 00266 00267 /** 00268 * @} 00269 */ 00270 00271 /** @addtogroup NAND_Exported_Functions_Group4 Peripheral State functions 00272 * @{ 00273 */ 00274 00275 /* NAND State functions *******************************************************/ 00276 HAL_NAND_StateTypeDef HAL_NAND_GetState(NAND_HandleTypeDef *hnand); 00277 uint32_t HAL_NAND_Read_Status(NAND_HandleTypeDef *hnand); 00278 00279 /** 00280 * @} 00281 */ 00282 00283 /** 00284 * @} 00285 */ 00286 00287 /** 00288 * @} 00289 */ 00290 00291 /** 00292 * @} 00293 */ 00294 00295 #ifdef __cplusplus 00296 } 00297 #endif 00298 00299 #endif /* __STM32L4xx_HAL_NAND_H */ 00300 00301 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00302
Generated on Tue Jul 12 2022 10:58:10 by
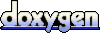