L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_hal_firewall.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_firewall.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of FIREWALL HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_FIREWALL_H 00040 #define __STM32L4xx_HAL_FIREWALL_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup FIREWALL FIREWALL 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup FIREWALL_Exported_Types FIREWALL Exported Types 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief FIREWALL Initialization Structure definition 00064 */ 00065 typedef struct 00066 { 00067 uint32_t CodeSegmentStartAddress; /*!< Protected code segment start address. This value is 24-bit long, the 8 LSB bits are 00068 reserved and forced to 0 in order to allow a 256-byte granularity. */ 00069 00070 uint32_t CodeSegmentLength; /*!< Protected code segment length in bytes. This value is 22-bit long, the 8 LSB bits are 00071 reserved and forced to 0 for the length to be a multiple of 256 bytes. */ 00072 00073 uint32_t NonVDataSegmentStartAddress; /*!< Protected non-volatile data segment start address. This value is 24-bit long, the 8 LSB 00074 bits are reserved and forced to 0 in order to allow a 256-byte granularity. */ 00075 00076 uint32_t NonVDataSegmentLength; /*!< Protected non-volatile data segment length in bytes. This value is 22-bit long, the 8 LSB 00077 bits are reserved and forced to 0 for the length to be a multiple of 256 bytes. */ 00078 00079 uint32_t VDataSegmentStartAddress; /*!< Protected volatile data segment start address. This value is 17-bit long, the 6 LSB bits 00080 are reserved and forced to 0 in order to allow a 64-byte granularity. */ 00081 00082 uint32_t VDataSegmentLength; /*!< Protected volatile data segment length in bytes. This value is 17-bit long, the 6 LSB 00083 bits are reserved and forced to 0 for the length to be a multiple of 64 bytes. */ 00084 00085 uint32_t VolatileDataExecution; /*!< Set VDE bit specifying whether or not the volatile data segment can be executed. 00086 When VDS = 1 (set by parameter VolatileDataShared), VDE bit has no meaning. 00087 This parameter can be a value of @ref FIREWALL_VolatileData_Executable */ 00088 00089 uint32_t VolatileDataShared; /*!< Set VDS bit in specifying whether or not the volatile data segment can be shared with a 00090 non-protected application code. 00091 This parameter can be a value of @ref FIREWALL_VolatileData_Shared */ 00092 00093 }FIREWALL_InitTypeDef; 00094 00095 00096 /** 00097 * @} 00098 */ 00099 00100 00101 /* Exported constants --------------------------------------------------------*/ 00102 /** @defgroup FIREWALL_Exported_Constants FIREWALL Exported Constants 00103 * @{ 00104 */ 00105 00106 /** @defgroup FIREWALL_VolatileData_Executable FIREWALL volatile data segment execution status 00107 * @{ 00108 */ 00109 #define FIREWALL_VOLATILEDATA_NOT_EXECUTABLE ((uint32_t)0x0000) 00110 #define FIREWALL_VOLATILEDATA_EXECUTABLE ((uint32_t)FW_CR_VDE) 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup FIREWALL_VolatileData_Shared FIREWALL volatile data segment share status 00116 * @{ 00117 */ 00118 #define FIREWALL_VOLATILEDATA_NOT_SHARED ((uint32_t)0x0000) 00119 #define FIREWALL_VOLATILEDATA_SHARED ((uint32_t)FW_CR_VDS) 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup FIREWALL_Pre_Arm FIREWALL pre arm status 00125 * @{ 00126 */ 00127 #define FIREWALL_PRE_ARM_RESET ((uint32_t)0x0000) 00128 #define FIREWALL_PRE_ARM_SET ((uint32_t)FW_CR_FPA) 00129 00130 /** 00131 * @} 00132 */ 00133 00134 /** 00135 * @} 00136 */ 00137 00138 /* Private macros --------------------------------------------------------*/ 00139 /** @defgroup FIREWALL_Private_Macros FIREWALL Private Macros 00140 * @{ 00141 */ 00142 #define IS_FIREWALL_CODE_SEGMENT_ADDRESS(ADDRESS) (((ADDRESS) >= FLASH_BASE) && ((ADDRESS) < (FLASH_BASE + FLASH_SIZE))) 00143 #define IS_FIREWALL_CODE_SEGMENT_LENGTH(ADDRESS, LENGTH) (((ADDRESS) + (LENGTH)) <= (FLASH_BASE + FLASH_SIZE)) 00144 00145 #define IS_FIREWALL_NONVOLATILEDATA_SEGMENT_ADDRESS(ADDRESS) (((ADDRESS) >= FLASH_BASE) && ((ADDRESS) < (FLASH_BASE + FLASH_SIZE))) 00146 #define IS_FIREWALL_NONVOLATILEDATA_SEGMENT_LENGTH(ADDRESS, LENGTH) (((ADDRESS) + (LENGTH)) <= (FLASH_BASE + FLASH_SIZE)) 00147 00148 #define IS_FIREWALL_VOLATILEDATA_SEGMENT_ADDRESS(ADDRESS) (((ADDRESS) >= SRAM1_BASE) && ((ADDRESS) < (SRAM1_BASE + SRAM1_SIZE_MAX))) 00149 #define IS_FIREWALL_VOLATILEDATA_SEGMENT_LENGTH(ADDRESS, LENGTH) (((ADDRESS) + (LENGTH)) <= (SRAM1_BASE + SRAM1_SIZE_MAX)) 00150 00151 00152 #define IS_FIREWALL_VOLATILEDATA_SHARE(SHARE) (((SHARE) == FIREWALL_VOLATILEDATA_NOT_SHARED) || \ 00153 ((SHARE) == FIREWALL_VOLATILEDATA_SHARED)) 00154 00155 #define IS_FIREWALL_VOLATILEDATA_EXECUTE(EXECUTE) (((EXECUTE) == FIREWALL_VOLATILEDATA_NOT_EXECUTABLE) || \ 00156 ((EXECUTE) == FIREWALL_VOLATILEDATA_EXECUTABLE)) 00157 /** 00158 * @} 00159 */ 00160 00161 00162 /* Exported macros -----------------------------------------------------------*/ 00163 /** @defgroup FIREWALL_Exported_Macros FIREWALL Exported Macros 00164 * @{ 00165 */ 00166 00167 /** @brief Check whether the FIREWALL is enabled or not. 00168 * @retval FIREWALL enabling status (TRUE or FALSE). 00169 */ 00170 #define __HAL_FIREWALL_IS_ENABLED() HAL_IS_BIT_CLR(SYSCFG->CFGR1, SYSCFG_CFGR1_FWDIS) 00171 00172 00173 /** @brief Enable FIREWALL pre arm. 00174 * @note When FPA bit is set, any code executed outside the protected segment 00175 * closes the Firewall, otherwise it generates a system reset. 00176 * @note This macro provides the same service as HAL_FIREWALL_EnablePreArmFlag() API 00177 * but can be executed inside a code area protected by the Firewall. 00178 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00179 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00180 * 0, that is, when the non volatile data segment is defined), the macro can be 00181 * executed only when the Firewall is opened. 00182 */ 00183 #define __HAL_FIREWALL_PREARM_ENABLE() \ 00184 do { \ 00185 __IO uint32_t tmpreg; \ 00186 SET_BIT(FIREWALL->CR, FW_CR_FPA) ; \ 00187 /* Read bit back to ensure it is taken into account by IP */ \ 00188 /* (introduce proper delay inside macro execution) */ \ 00189 tmpreg = READ_BIT(FIREWALL->CR, FW_CR_FPA) ; \ 00190 UNUSED(tmpreg); \ 00191 } while(0) 00192 00193 00194 00195 /** @brief Disable FIREWALL pre arm. 00196 * @note When FPA bit is set, any code executed outside the protected segment 00197 * closes the Firewall, otherwise, it generates a system reset. 00198 * @note This macro provides the same service as HAL_FIREWALL_DisablePreArmFlag() API 00199 * but can be executed inside a code area protected by the Firewall. 00200 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00201 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00202 * 0, that is, when the non volatile data segment is defined), the macro can be 00203 * executed only when the Firewall is opened. 00204 */ 00205 #define __HAL_FIREWALL_PREARM_DISABLE() \ 00206 do { \ 00207 __IO uint32_t tmpreg; \ 00208 CLEAR_BIT(FIREWALL->CR, FW_CR_FPA) ; \ 00209 /* Read bit back to ensure it is taken into account by IP */ \ 00210 /* (introduce proper delay inside macro execution) */ \ 00211 tmpreg = READ_BIT(FIREWALL->CR, FW_CR_FPA) ; \ 00212 UNUSED(tmpreg); \ 00213 } while(0) 00214 00215 /** @brief Enable volatile data sharing in setting VDS bit. 00216 * @note When VDS bit is set, the volatile data segment is shared with non-protected 00217 * application code. It can be accessed whatever the Firewall state (opened or closed). 00218 * @note This macro can be executed inside a code area protected by the Firewall. 00219 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00220 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00221 * 0, that is, when the non volatile data segment is defined), the macro can be 00222 * executed only when the Firewall is opened. 00223 */ 00224 #define __HAL_FIREWALL_VOLATILEDATA_SHARED_ENABLE() \ 00225 do { \ 00226 __IO uint32_t tmpreg; \ 00227 SET_BIT(FIREWALL->CR, FW_CR_VDS) ; \ 00228 /* Read bit back to ensure it is taken into account by IP */ \ 00229 /* (introduce proper delay inside macro execution) */ \ 00230 tmpreg = READ_BIT(FIREWALL->CR, FW_CR_VDS) ; \ 00231 UNUSED(tmpreg); \ 00232 } while(0) 00233 00234 /** @brief Disable volatile data sharing in resetting VDS bit. 00235 * @note When VDS bit is reset, the volatile data segment is not shared and cannot be 00236 * hit by a non protected executable code when the Firewall is closed. If it is 00237 * accessed in such a condition, a system reset is generated by the Firewall. 00238 * @note This macro can be executed inside a code area protected by the Firewall. 00239 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00240 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00241 * 0, that is, when the non volatile data segment is defined), the macro can be 00242 * executed only when the Firewall is opened. 00243 */ 00244 #define __HAL_FIREWALL_VOLATILEDATA_SHARED_DISABLE() \ 00245 do { \ 00246 __IO uint32_t tmpreg; \ 00247 CLEAR_BIT(FIREWALL->CR, FW_CR_VDS) ; \ 00248 /* Read bit back to ensure it is taken into account by IP */ \ 00249 /* (introduce proper delay inside macro execution) */ \ 00250 tmpreg = READ_BIT(FIREWALL->CR, FW_CR_VDS) ; \ 00251 UNUSED(tmpreg); \ 00252 } while(0) 00253 00254 /** @brief Enable volatile data execution in setting VDE bit. 00255 * @note VDE bit is ignored when VDS is set. IF VDS = 1, the Volatile data segment can be 00256 * executed whatever the VDE bit value. 00257 * @note When VDE bit is set (with VDS = 0), the volatile data segment is executable. When 00258 * the Firewall call is closed, a "call gate" entry procedure is required to open 00259 * first the Firewall. 00260 * @note This macro can be executed inside a code area protected by the Firewall. 00261 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00262 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00263 * 0, that is, when the non volatile data segment is defined), the macro can be 00264 * executed only when the Firewall is opened. 00265 */ 00266 #define __HAL_FIREWALL_VOLATILEDATA_EXECUTION_ENABLE() \ 00267 do { \ 00268 __IO uint32_t tmpreg; \ 00269 SET_BIT(FIREWALL->CR, FW_CR_VDE) ; \ 00270 /* Read bit back to ensure it is taken into account by IP */ \ 00271 /* (introduce proper delay inside macro execution) */ \ 00272 tmpreg = READ_BIT(FIREWALL->CR, FW_CR_VDE) ; \ 00273 UNUSED(tmpreg); \ 00274 } while(0) 00275 00276 /** @brief Disable volatile data execution in resetting VDE bit. 00277 * @note VDE bit is ignored when VDS is set. IF VDS = 1, the Volatile data segment can be 00278 * executed whatever the VDE bit value. 00279 * @note When VDE bit is reset (with VDS = 0), the volatile data segment cannot be executed. 00280 * @note This macro can be executed inside a code area protected by the Firewall. 00281 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00282 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00283 * 0, that is, when the non volatile data segment is defined), the macro can be 00284 * executed only when the Firewall is opened. 00285 */ 00286 #define __HAL_FIREWALL_VOLATILEDATA_EXECUTION_DISABLE() \ 00287 do { \ 00288 __IO uint32_t tmpreg; \ 00289 CLEAR_BIT(FIREWALL->CR, FW_CR_VDE) ; \ 00290 /* Read bit back to ensure it is taken into account by IP */ \ 00291 /* (introduce proper delay inside macro execution) */ \ 00292 tmpreg = READ_BIT(FIREWALL->CR, FW_CR_VDE) ; \ 00293 UNUSED(tmpreg); \ 00294 } while(0) 00295 00296 00297 /** @brief Check whether or not the volatile data segment is shared. 00298 * @note This macro can be executed inside a code area protected by the Firewall. 00299 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00300 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00301 * 0, that is, when the non volatile data segment is defined), the macro can be 00302 * executed only when the Firewall is opened. 00303 * @retval VDS bit setting status (TRUE or FALSE). 00304 */ 00305 #define __HAL_FIREWALL_GET_VOLATILEDATA_SHARED() ((FIREWALL->CR & FW_CR_VDS) == FW_CR_VDS) 00306 00307 /** @brief Check whether or not the volatile data segment is declared executable. 00308 * @note This macro can be executed inside a code area protected by the Firewall. 00309 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00310 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00311 * 0, that is, when the non volatile data segment is defined), the macro can be 00312 * executed only when the Firewall is opened. 00313 * @retval VDE bit setting status (TRUE or FALSE). 00314 */ 00315 #define __HAL_FIREWALL_GET_VOLATILEDATA_EXECUTION() ((FIREWALL->CR & FW_CR_VDE) == FW_CR_VDE) 00316 00317 /** @brief Check whether or not the Firewall pre arm bit is set. 00318 * @note This macro can be executed inside a code area protected by the Firewall. 00319 * @note This macro can be executed whatever the Firewall state (opened or closed) when 00320 * NVDSL register is equal to 0. Otherwise (when NVDSL register is different from 00321 * 0, that is, when the non volatile data segment is defined), the macro can be 00322 * executed only when the Firewall is opened. 00323 * @retval FPA bit setting status (TRUE or FALSE). 00324 */ 00325 #define __HAL_FIREWALL_GET_PREARM() ((FIREWALL->CR & FW_CR_FPA) == FW_CR_FPA) 00326 00327 00328 /** 00329 * @} 00330 */ 00331 00332 /* Exported functions --------------------------------------------------------*/ 00333 00334 /** @addtogroup FIREWALL_Exported_Functions FIREWALL Exported Functions 00335 * @{ 00336 */ 00337 00338 /** @addtogroup FIREWALL_Exported_Functions_Group1 Initialization Functions 00339 * @brief Initialization and Configuration Functions 00340 * @{ 00341 */ 00342 00343 /* Initialization functions ********************************/ 00344 HAL_StatusTypeDef HAL_FIREWALL_Config(FIREWALL_InitTypeDef * fw_init); 00345 void HAL_FIREWALL_GetConfig(FIREWALL_InitTypeDef * fw_config); 00346 void HAL_FIREWALL_EnableFirewall(void); 00347 void HAL_FIREWALL_EnablePreArmFlag(void); 00348 void HAL_FIREWALL_DisablePreArmFlag(void); 00349 00350 /** 00351 * @} 00352 */ 00353 00354 /** 00355 * @} 00356 */ 00357 00358 /** 00359 * @} 00360 */ 00361 00362 /** 00363 * @} 00364 */ 00365 00366 #ifdef __cplusplus 00367 } 00368 #endif 00369 00370 #endif /* __STM32L4xx_HAL_FIREWALL_H */ 00371 00372 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00373
Generated on Tue Jul 12 2022 10:58:09 by
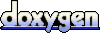