L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_hal_firewall.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_firewall.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief FIREWALL HAL module driver. 00008 * This file provides firmware functions to manage the Firewall 00009 * Peripheral initialization and enabling. 00010 * 00011 * 00012 @verbatim 00013 =============================================================================== 00014 ##### How to use this driver ##### 00015 =============================================================================== 00016 [..] 00017 The FIREWALL HAL driver can be used as follows: 00018 00019 (#) Declare a FIREWALL_InitTypeDef initialization structure. 00020 00021 (#) Resort to HAL_FIREWALL_Config() API to initialize the Firewall 00022 00023 (#) Enable the FIREWALL in calling HAL_FIREWALL_EnableFirewall() API 00024 00025 (#) To ensure that any code executed outside the protected segment closes the 00026 FIREWALL, the user must set the flag FIREWALL_PRE_ARM_SET in calling 00027 __HAL_FIREWALL_PREARM_ENABLE() macro if called within a protected code segment 00028 or 00029 HAL_FIREWALL_EnablePreArmFlag() API if called outside of protected code segment 00030 after HAL_FIREWALL_Config() call. 00031 00032 00033 @endverbatim 00034 ****************************************************************************** 00035 * @attention 00036 * 00037 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00038 * 00039 * Redistribution and use in source and binary forms, with or without modification, 00040 * are permitted provided that the following conditions are met: 00041 * 1. Redistributions of source code must retain the above copyright notice, 00042 * this list of conditions and the following disclaimer. 00043 * 2. Redistributions in binary form must reproduce the above copyright notice, 00044 * this list of conditions and the following disclaimer in the documentation 00045 * and/or other materials provided with the distribution. 00046 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00047 * may be used to endorse or promote products derived from this software 00048 * without specific prior written permission. 00049 * 00050 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00051 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00052 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00053 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00054 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00055 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00056 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00057 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00058 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00059 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00060 * 00061 ****************************************************************************** 00062 */ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm32l4xx_hal.h" 00066 00067 /** @addtogroup STM32L4xx_HAL_Driver 00068 * @{ 00069 */ 00070 00071 /** @defgroup FIREWALL FIREWALL 00072 * @brief HAL FIREWALL module driver 00073 * @{ 00074 */ 00075 #ifdef HAL_FIREWALL_MODULE_ENABLED 00076 00077 /* Private typedef -----------------------------------------------------------*/ 00078 /* Private define ------------------------------------------------------------*/ 00079 /* Private macro -------------------------------------------------------------*/ 00080 /* Private variables ---------------------------------------------------------*/ 00081 /* Private function prototypes -----------------------------------------------*/ 00082 /* Private functions ---------------------------------------------------------*/ 00083 00084 00085 /** @defgroup FIREWALL_Exported_Functions FIREWALL Exported Functions 00086 * @{ 00087 */ 00088 00089 /** @defgroup FIREWALL_Exported_Functions_Group1 Initialization Functions 00090 * @brief Initialization and Configuration Functions 00091 * 00092 @verbatim 00093 =============================================================================== 00094 ##### Initialization and Configuration functions ##### 00095 =============================================================================== 00096 [..] 00097 This subsection provides the functions allowing to initialize the Firewall. 00098 Initialization is done by HAL_FIREWALL_Config(): 00099 00100 (+) Enable the Firewall clock thru __HAL_RCC_FIREWALL_CLK_ENABLE() macro. 00101 00102 (+) Set the protected code segment address start and length. 00103 00104 (+) Set the protected non-volatile and/or volatile data segments 00105 address starts and lengths if applicable. 00106 00107 (+) Set the volatile data segment execution and sharing status. 00108 00109 (+) Length must be set to 0 for an unprotected segment. 00110 00111 @endverbatim 00112 * @{ 00113 */ 00114 00115 /** 00116 * @brief Initialize the Firewall according to the FIREWALL_InitTypeDef structure parameters. 00117 * @param fw_init: Firewall initialization structure 00118 * @note The API returns HAL_ERROR if the Firewall is already enabled. 00119 * @retval HAL status 00120 */ 00121 HAL_StatusTypeDef HAL_FIREWALL_Config(FIREWALL_InitTypeDef * fw_init) 00122 { 00123 /* Check the Firewall initialization structure allocation */ 00124 if(fw_init == NULL) 00125 { 00126 return HAL_ERROR; 00127 } 00128 00129 /* Enable Firewall clock */ 00130 __HAL_RCC_FIREWALL_CLK_ENABLE(); 00131 00132 /* Make sure that Firewall is not enabled already */ 00133 if (__HAL_FIREWALL_IS_ENABLED() != RESET) 00134 { 00135 return HAL_ERROR; 00136 } 00137 00138 /* Check Firewall configuration addresses and lengths when segment is protected */ 00139 /* Code segment */ 00140 if (fw_init->CodeSegmentLength != 0) 00141 { 00142 assert_param(IS_FIREWALL_CODE_SEGMENT_ADDRESS(fw_init->CodeSegmentStartAddress)); 00143 assert_param(IS_FIREWALL_CODE_SEGMENT_LENGTH(fw_init->CodeSegmentStartAddress, fw_init->CodeSegmentLength)); 00144 } 00145 /* Non volatile data segment */ 00146 if (fw_init->NonVDataSegmentLength != 0) 00147 { 00148 assert_param(IS_FIREWALL_NONVOLATILEDATA_SEGMENT_ADDRESS(fw_init->NonVDataSegmentStartAddress)); 00149 assert_param(IS_FIREWALL_NONVOLATILEDATA_SEGMENT_LENGTH(fw_init->NonVDataSegmentStartAddress, fw_init->NonVDataSegmentLength)); 00150 } 00151 /* Volatile data segment */ 00152 if (fw_init->VDataSegmentLength != 0) 00153 { 00154 assert_param(IS_FIREWALL_VOLATILEDATA_SEGMENT_ADDRESS(fw_init->VDataSegmentStartAddress)); 00155 assert_param(IS_FIREWALL_VOLATILEDATA_SEGMENT_LENGTH(fw_init->VDataSegmentStartAddress, fw_init->VDataSegmentLength)); 00156 } 00157 00158 /* Check Firewall Configuration Register parameters */ 00159 assert_param(IS_FIREWALL_VOLATILEDATA_EXECUTE(fw_init->VolatileDataExecution)); 00160 assert_param(IS_FIREWALL_VOLATILEDATA_SHARE(fw_init->VolatileDataShared)); 00161 00162 00163 /* Configuration */ 00164 00165 /* Protected code segment start address configuration */ 00166 WRITE_REG(FIREWALL->CSSA, (FW_CSSA_ADD & fw_init->CodeSegmentStartAddress)); 00167 /* Protected code segment length configuration */ 00168 WRITE_REG(FIREWALL->CSL, (FW_CSL_LENG & fw_init->CodeSegmentLength)); 00169 00170 /* Protected non volatile data segment start address configuration */ 00171 WRITE_REG(FIREWALL->NVDSSA, (FW_NVDSSA_ADD & fw_init->NonVDataSegmentStartAddress)); 00172 /* Protected non volatile data segment length configuration */ 00173 WRITE_REG(FIREWALL->NVDSL, (FW_NVDSL_LENG & fw_init->NonVDataSegmentLength)); 00174 00175 /* Protected volatile data segment start address configuration */ 00176 WRITE_REG(FIREWALL->VDSSA, (FW_VDSSA_ADD & fw_init->VDataSegmentStartAddress)); 00177 /* Protected volatile data segment length configuration */ 00178 WRITE_REG(FIREWALL->VDSL, (FW_VDSL_LENG & fw_init->VDataSegmentLength)); 00179 00180 /* Set Firewall Configuration Register VDE and VDS bits 00181 (volatile data execution and shared configuration) */ 00182 MODIFY_REG(FIREWALL->CR, FW_CR_VDS|FW_CR_VDE, fw_init->VolatileDataExecution|fw_init->VolatileDataShared); 00183 00184 return HAL_OK; 00185 } 00186 00187 /** 00188 * @brief Retrieve the Firewall configuration. 00189 * @param fw_config: Firewall configuration, type is same as initialization structure 00190 * @note This API can't be executed inside a code area protected by the Firewall 00191 * when the Firewall is enabled 00192 * @note If NVDSL register is different from 0, that is, if the non volatile data segment 00193 * is defined, this API can't be executed when the Firewall is enabled. 00194 * @note User should resort to __HAL_FIREWALL_GET_PREARM() macro to retrieve FPA bit status 00195 * @retval None 00196 */ 00197 void HAL_FIREWALL_GetConfig(FIREWALL_InitTypeDef * fw_config) 00198 { 00199 00200 /* Enable Firewall clock, in case no Firewall configuration has been carried 00201 out up to this point */ 00202 __HAL_RCC_FIREWALL_CLK_ENABLE(); 00203 00204 /* Retrieve code segment protection setting */ 00205 fw_config->CodeSegmentStartAddress = (READ_REG(FIREWALL->CSSA) & FW_CSSA_ADD); 00206 fw_config->CodeSegmentLength = (READ_REG(FIREWALL->CSL) & FW_CSL_LENG); 00207 00208 /* Retrieve non volatile data segment protection setting */ 00209 fw_config->NonVDataSegmentStartAddress = (READ_REG(FIREWALL->NVDSSA) & FW_NVDSSA_ADD); 00210 fw_config->NonVDataSegmentLength = (READ_REG(FIREWALL->NVDSL) & FW_NVDSL_LENG); 00211 00212 /* Retrieve volatile data segment protection setting */ 00213 fw_config->VDataSegmentStartAddress = (READ_REG(FIREWALL->VDSSA) & FW_VDSSA_ADD); 00214 fw_config->VDataSegmentLength = (READ_REG(FIREWALL->VDSL) & FW_VDSL_LENG); 00215 00216 /* Retrieve volatile data execution setting */ 00217 fw_config->VolatileDataExecution = (READ_REG(FIREWALL->CR) & FW_CR_VDE); 00218 00219 /* Retrieve volatile data shared setting */ 00220 fw_config->VolatileDataShared = (READ_REG(FIREWALL->CR) & FW_CR_VDS); 00221 00222 return; 00223 } 00224 00225 00226 00227 /** 00228 * @brief Enable FIREWALL. 00229 * @note Firewall is enabled in clearing FWDIS bit of SYSCFG CFGR1 register. 00230 * Once enabled, the Firewall cannot be disabled by software. Only a 00231 * system reset can set again FWDIS bit. 00232 * @retval None 00233 */ 00234 void HAL_FIREWALL_EnableFirewall(void) 00235 { 00236 /* Clears FWDIS bit of SYSCFG CFGR1 register */ 00237 CLEAR_BIT(SYSCFG->CFGR1, SYSCFG_CFGR1_FWDIS); 00238 00239 } 00240 00241 /** 00242 * @brief Enable FIREWALL pre arm. 00243 * @note When FPA bit is set, any code executed outside the protected segment 00244 * will close the Firewall. 00245 * @note This API provides the same service as __HAL_FIREWALL_PREARM_ENABLE() macro 00246 * but can't be executed inside a code area protected by the Firewall. 00247 * @note When the Firewall is disabled, user can resort to HAL_FIREWALL_EnablePreArmFlag() API any time. 00248 * @note When the Firewall is enabled and NVDSL register is equal to 0 (that is, 00249 * when the non volatile data segment is not defined), 00250 * ** this API can be executed when the Firewall is closed 00251 * ** when the Firewall is opened, user should resort to 00252 * __HAL_FIREWALL_PREARM_ENABLE() macro instead 00253 * @note When the Firewall is enabled and NVDSL register is different from 0 00254 * (that is, when the non volatile data segment is defined) 00255 * ** FW_CR register can be accessed only when the Firewall is opened: 00256 * user should resort to __HAL_FIREWALL_PREARM_ENABLE() macro instead. 00257 * @retval None 00258 */ 00259 void HAL_FIREWALL_EnablePreArmFlag(void) 00260 { 00261 /* Set FPA bit */ 00262 SET_BIT(FIREWALL->CR, FW_CR_FPA); 00263 } 00264 00265 00266 /** 00267 * @brief Disable FIREWALL pre arm. 00268 * @note When FPA bit is reset, any code executed outside the protected segment 00269 * when the Firewall is opened will generate a system reset. 00270 * @note This API provides the same service as __HAL_FIREWALL_PREARM_DISABLE() macro 00271 * but can't be executed inside a code area protected by the Firewall. 00272 * @note When the Firewall is disabled, user can resort to HAL_FIREWALL_EnablePreArmFlag() API any time. 00273 * @note When the Firewall is enabled and NVDSL register is equal to 0 (that is, 00274 * when the non volatile data segment is not defined), 00275 * ** this API can be executed when the Firewall is closed 00276 * ** when the Firewall is opened, user should resort to 00277 * __HAL_FIREWALL_PREARM_DISABLE() macro instead 00278 * @note When the Firewall is enabled and NVDSL register is different from 0 00279 * (that is, when the non volatile data segment is defined) 00280 * ** FW_CR register can be accessed only when the Firewall is opened: 00281 * user should resort to __HAL_FIREWALL_PREARM_DISABLE() macro instead. 00282 00283 * @retval None 00284 */ 00285 void HAL_FIREWALL_DisablePreArmFlag(void) 00286 { 00287 /* Clear FPA bit */ 00288 CLEAR_BIT(FIREWALL->CR, FW_CR_FPA); 00289 } 00290 00291 /** 00292 * @} 00293 */ 00294 00295 /** 00296 * @} 00297 */ 00298 00299 #endif /* HAL_FIREWALL_MODULE_ENABLED */ 00300 /** 00301 * @} 00302 */ 00303 00304 /** 00305 * @} 00306 */ 00307 00308 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00309
Generated on Tue Jul 12 2022 10:58:09 by
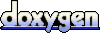