L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_hal_cryp.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_cryp.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of CRYP HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_CRYP_H 00040 #define __STM32L4xx_HAL_CRYP_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 #if defined(STM32L485xx) || defined(STM32L486xx) 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32l4xx_hal_def.h" 00050 00051 /** @addtogroup STM32L4xx_HAL_Driver 00052 * @{ 00053 */ 00054 00055 /** @addtogroup CRYP 00056 * @{ 00057 */ 00058 00059 /* Exported types ------------------------------------------------------------*/ 00060 /** @defgroup CRYP_Exported_Types CRYP Exported Types 00061 * @{ 00062 */ 00063 00064 /** 00065 * @brief CRYP Configuration Structure definition 00066 */ 00067 typedef struct 00068 { 00069 uint32_t DataType; /*!< 32-bit data, 16-bit data, 8-bit data or 1-bit string. 00070 This parameter can be a value of @ref CRYP_Data_Type */ 00071 00072 uint32_t KeySize; /*!< 128 or 256-bit key length. 00073 This parameter can be a value of @ref CRYP_Key_Size */ 00074 00075 uint32_t OperatingMode; /*!< AES operating mode. 00076 This parameter can be a value of @ref CRYP_AES_OperatingMode */ 00077 00078 uint32_t ChainingMode; /*!< AES chaining mode. 00079 This parameter can be a value of @ref CRYP_AES_ChainingMode */ 00080 00081 uint32_t KeyWriteFlag; /*!< Allows to bypass or not key write-up before decryption. 00082 This parameter can be a value of @ref CRYP_Key_Write */ 00083 00084 uint32_t GCMCMACPhase; /*!< Indicates the processing phase of the Galois Counter Mode (GCM), 00085 Galois Message Authentication Code (GMAC) or Cipher Message 00086 Authentication Code (CMAC) mode. 00087 This parameter can be a value of @ref CRYP_GCM_CMAC_Phase */ 00088 00089 uint8_t* pKey; /*!< Encryption/Decryption Key */ 00090 00091 uint8_t* pInitVect; /*!< Initialization Vector used for CTR, CBC, GCM/GMAC and CMAC modes */ 00092 00093 uint8_t* Header; /*!< Header used in GCM/GMAC and CMAC modes */ 00094 00095 uint64_t HeaderSize; /*!< Header size in bytes */ 00096 00097 }CRYP_InitTypeDef; 00098 00099 /** 00100 * @brief HAL CRYP State structures definition 00101 */ 00102 typedef enum 00103 { 00104 HAL_CRYP_STATE_RESET = 0x00, /*!< CRYP not yet initialized or disabled */ 00105 HAL_CRYP_STATE_READY = 0x01, /*!< CRYP initialized and ready for use */ 00106 HAL_CRYP_STATE_BUSY = 0x02, /*!< CRYP internal processing is ongoing */ 00107 HAL_CRYP_STATE_TIMEOUT = 0x03, /*!< CRYP timeout state */ 00108 HAL_CRYP_STATE_ERROR = 0x04, /*!< CRYP error state */ 00109 HAL_CRYP_STATE_SUSPENDED = 0x05 /*!< CRYP suspended */ 00110 }HAL_CRYP_STATETypeDef; 00111 00112 /** 00113 * @brief HAL CRYP phase structures definition 00114 */ 00115 typedef enum 00116 { 00117 HAL_CRYP_PHASE_READY = 0x01, /*!< CRYP peripheral is ready for initialization. */ 00118 HAL_CRYP_PHASE_PROCESS = 0x02, /*!< CRYP peripheral is in processing phase */ 00119 HAL_CRYP_PHASE_START = 0x03, /*!< CRYP peripheral has been initialized but GCM/GMAC/CMAC 00120 initialization phase has not started */ 00121 HAL_CRYP_PHASE_INIT_OVER = 0x04, /*!< GCM/GMAC/CMAC init phase has been carried out */ 00122 HAL_CRYP_PHASE_HEADER_OVER = 0x05, /*!< GCM/GMAC/CMAC header phase has been carried out */ 00123 HAL_CRYP_PHASE_PAYLOAD_OVER = 0x06, /*!< GCM/GMAC/CMAC payload phase has been carried out */ 00124 HAL_CRYP_PHASE_FINAL_OVER = 0x07, /*!< GCM/GMAC/CMAC final phase has been carried out */ 00125 HAL_CRYP_PHASE_HEADER_SUSPENDED = 0x08, /*!< GCM/GMAC/CMAC header phase has been suspended */ 00126 HAL_CRYP_PHASE_PAYLOAD_SUSPENDED = 0x09, /*!< GCM/GMAC payload phase has been suspended */ 00127 HAL_CRYP_PHASE_NOT_USED = 0x0a /*!< Phase is irrelevant to the current chaining mode */ 00128 }HAL_PhaseTypeDef; 00129 00130 /** 00131 * @brief HAL CRYP mode suspend definitions 00132 */ 00133 typedef enum 00134 { 00135 HAL_CRYP_SUSPEND_NONE = 0x00, /*!< CRYP peripheral suspension not requested */ 00136 HAL_CRYP_SUSPEND = 0x01 /*!< CRYP peripheral suspension requested */ 00137 }HAL_SuspendTypeDef; 00138 00139 00140 /** 00141 * @brief HAL CRYP Error Codes definition 00142 */ 00143 #define HAL_CRYP_ERROR_NONE ((uint32_t)0x00000000) /*!< No error */ 00144 #define HAL_CRYP_WRITE_ERROR ((uint32_t)0x00000001) /*!< Write error */ 00145 #define HAL_CRYP_READ_ERROR ((uint32_t)0x00000002) /*!< Read error */ 00146 #define HAL_CRYP_DMA_ERROR ((uint32_t)0x00000004) /*!< DMA error */ 00147 00148 00149 /** 00150 * @brief CRYP handle Structure definition 00151 */ 00152 typedef struct 00153 { 00154 AES_TypeDef *Instance; /*!< Register base address */ 00155 00156 CRYP_InitTypeDef Init; /*!< CRYP initialization parameters */ 00157 00158 uint8_t *pCrypInBuffPtr; /*!< Pointer to CRYP processing (encryption, decryption,...) input buffer */ 00159 00160 uint8_t *pCrypOutBuffPtr; /*!< Pointer to CRYP processing (encryption, decryption,...) output buffer */ 00161 00162 __IO uint16_t CrypInCount; /*!< Input data size in bytes or, after suspension, the remaining 00163 number of bytes to process */ 00164 00165 __IO uint16_t CrypOutCount; /*!< Output data size in bytes */ 00166 00167 HAL_PhaseTypeDef Phase; /*!< CRYP peripheral processing phase for GCM, GMAC or CMAC modes. 00168 Indicates the last phase carried out to ease 00169 phase transitions */ 00170 00171 DMA_HandleTypeDef *hdmain; /*!< CRYP peripheral Input DMA handle parameters */ 00172 00173 DMA_HandleTypeDef *hdmaout; /*!< CRYP peripheral Output DMA handle parameters */ 00174 00175 HAL_LockTypeDef Lock; /*!< CRYP locking object */ 00176 00177 __IO HAL_CRYP_STATETypeDef State; /*!< CRYP peripheral state */ 00178 00179 __IO uint32_t ErrorCode; /*!< CRYP peripheral error code */ 00180 00181 HAL_SuspendTypeDef SuspendRequest; /*!< CRYP peripheral suspension request flag */ 00182 }CRYP_HandleTypeDef; 00183 00184 /** 00185 * @} 00186 */ 00187 00188 00189 /* Exported constants --------------------------------------------------------*/ 00190 /** @defgroup CRYP_Exported_Constants CRYP Exported Constants 00191 * @{ 00192 */ 00193 00194 /** @defgroup CRYP_Key_Size Key size selection 00195 * @{ 00196 */ 00197 #define CRYP_KEYSIZE_128B ((uint32_t)0x00000000) /*!< 128-bit long key */ 00198 #define CRYP_KEYSIZE_256B AES_CR_KEYSIZE /*!< 256-bit long key */ 00199 /** 00200 * @} 00201 */ 00202 00203 /** @defgroup CRYP_Data_Type AES Data Type selection 00204 * @{ 00205 */ 00206 #define CRYP_DATATYPE_32B ((uint32_t)0x00000000) /*!< 32-bit data type (no swapping) */ 00207 #define CRYP_DATATYPE_16B AES_CR_DATATYPE_0 /*!< 16-bit data type (half-word swapping) */ 00208 #define CRYP_DATATYPE_8B AES_CR_DATATYPE_1 /*!< 8-bit data type (byte swapping) */ 00209 #define CRYP_DATATYPE_1B AES_CR_DATATYPE /*!< 1-bit data type (bit swapping) */ 00210 /** 00211 * @} 00212 */ 00213 00214 /** @defgroup CRYP_AES_State AES Enable state 00215 * @{ 00216 */ 00217 #define CRYP_AES_DISABLE ((uint32_t)0x00000000) /*!< Disable AES */ 00218 #define CRYP_AES_ENABLE AES_CR_EN /*!< Enable AES */ 00219 /** 00220 * @} 00221 */ 00222 00223 /** @defgroup CRYP_AES_OperatingMode AES operating mode 00224 * @{ 00225 */ 00226 #define CRYP_ALGOMODE_ENCRYPT ((uint32_t)0x00000000) /*!< Encryption mode */ 00227 #define CRYP_ALGOMODE_KEYDERIVATION AES_CR_MODE_0 /*!< Key derivation mode */ 00228 #define CRYP_ALGOMODE_DECRYPT AES_CR_MODE_1 /*!< Decryption */ 00229 #define CRYP_ALGOMODE_KEYDERIVATION_DECRYPT AES_CR_MODE /*!< Key derivation and decryption */ 00230 #define CRYP_ALGOMODE_TAG_GENERATION ((uint32_t)0x00000000) /*!< GMAC or CMAC authentication tag generation */ 00231 /** 00232 * @} 00233 */ 00234 00235 /** @defgroup CRYP_AES_ChainingMode AES chaining mode 00236 * @{ 00237 */ 00238 #define CRYP_CHAINMODE_AES_ECB ((uint32_t)0x00000000) /*!< Electronic codebook chaining algorithm */ 00239 #define CRYP_CHAINMODE_AES_CBC AES_CR_CHMOD_0 /*!< Cipher block chaining algorithm */ 00240 #define CRYP_CHAINMODE_AES_CTR AES_CR_CHMOD_1 /*!< Counter mode chaining algorithm */ 00241 #define CRYP_CHAINMODE_AES_GCM_GMAC (AES_CR_CHMOD_0 | AES_CR_CHMOD_1) /*!< Galois counter mode - Galois message authentication code */ 00242 #define CRYP_CHAINMODE_AES_CMAC AES_CR_CHMOD_2 /*!< Cipher message authentication code */ 00243 /** 00244 * @} 00245 */ 00246 00247 /** @defgroup CRYP_Key_Write AES decryption key write-up flag 00248 * @{ 00249 */ 00250 #define CRYP_KEY_WRITE_ENABLE ((uint32_t)0x00000000) /*!< Enable decryption key writing */ 00251 #define CRYP_KEY_WRITE_DISABLE ((uint32_t)0x00000001) /*!< Disable decryption key writing */ 00252 /** 00253 * @} 00254 */ 00255 00256 /** @defgroup CRYP_DMAIN DMA Input phase management enable state 00257 * @{ 00258 */ 00259 #define CRYP_DMAIN_DISABLE ((uint32_t)0x00000000) /*!< Disable DMA Input phase management */ 00260 #define CRYP_DMAIN_ENABLE AES_CR_DMAINEN /*!< Enable DMA Input phase management */ 00261 /** 00262 * @} 00263 */ 00264 00265 /** @defgroup CRYP_DMAOUT DMA Output phase management enable state 00266 * @{ 00267 */ 00268 #define CRYP_DMAOUT_DISABLE ((uint32_t)0x00000000) /*!< Disable DMA Output phase management */ 00269 #define CRYP_DMAOUT_ENABLE AES_CR_DMAOUTEN /*!< Enable DMA Output phase management */ 00270 /** 00271 * @} 00272 */ 00273 00274 00275 /** @defgroup CRYP_GCM_CMAC_Phase GCM/GMAC and CMAC processing phase selection 00276 * @{ 00277 */ 00278 #define CRYP_GCM_INIT_PHASE ((uint32_t)0x00000000) /*!< GCM/GMAC init phase */ 00279 #define CRYP_GCMCMAC_HEADER_PHASE AES_CR_GCMPH_0 /*!< GCM/GMAC or CMAC header phase */ 00280 #define CRYP_GCM_PAYLOAD_PHASE AES_CR_GCMPH_1 /*!< GCM payload phaset */ 00281 #define CRYP_GCMCMAC_FINAL_PHASE AES_CR_GCMPH /*!< GCM/GMAC or CMAC final phase */ 00282 /** 00283 * @} 00284 */ 00285 00286 /** @defgroup CRYP_Flags AES status flags 00287 * @{ 00288 */ 00289 00290 #define CRYP_FLAG_BUSY AES_SR_BUSY /*!< GCM process suspension forbidden */ 00291 #define CRYP_FLAG_WRERR AES_SR_WRERR /*!< Write Error */ 00292 #define CRYP_FLAG_RDERR AES_SR_RDERR /*!< Read error */ 00293 #define CRYP_FLAG_CCF AES_SR_CCF /*!< Computation completed */ 00294 /** 00295 * @} 00296 */ 00297 00298 /** @defgroup CRYP_Clear_Flags AES clearing flags 00299 * @{ 00300 */ 00301 00302 #define CRYP_CCF_CLEAR AES_CR_CCFC /*!< Computation Complete Flag Clear */ 00303 #define CRYP_ERR_CLEAR AES_CR_ERRC /*!< Error Flag Clear */ 00304 /** 00305 * @} 00306 */ 00307 00308 /** @defgroup AES_Interrupts_Enable AES Interrupts Enable bits 00309 * @{ 00310 */ 00311 #define CRYP_IT_CCFIE AES_CR_CCFIE /*!< Computation Complete interrupt enable */ 00312 #define CRYP_IT_ERRIE AES_CR_ERRIE /*!< Error interrupt enable */ 00313 /** 00314 * @} 00315 */ 00316 00317 /** @defgroup CRYP_Interrupts_Flags AES Interrupts flags 00318 * @{ 00319 */ 00320 #define CRYP_IT_WRERR AES_SR_WRERR /*!< Write Error */ 00321 #define CRYP_IT_RDERR AES_SR_RDERR /*!< Read Error */ 00322 #define CRYP_IT_CCF AES_SR_CCF /*!< Computation completed */ 00323 /** 00324 * @} 00325 */ 00326 00327 /** 00328 * @} 00329 */ 00330 00331 /* Exported macros -----------------------------------------------------------*/ 00332 /** @defgroup CRYP_Exported_Macros CRYP Exported Macros 00333 * @{ 00334 */ 00335 00336 /** @brief Reset CRYP handle state. 00337 * @param __HANDLE__: specifies the CRYP handle. 00338 * @retval None 00339 */ 00340 #define __HAL_CRYP_RESET_HANDLE_STATE(__HANDLE__) ((__HANDLE__)->State = HAL_CRYP_STATE_RESET) 00341 00342 /** 00343 * @brief Enable the CRYP AES peripheral. 00344 * @retval None 00345 */ 00346 #define __HAL_CRYP_ENABLE() (AES->CR |= AES_CR_EN) 00347 00348 /** 00349 * @brief Disable the CRYP AES peripheral. 00350 * @retval None 00351 */ 00352 #define __HAL_CRYP_DISABLE() (AES->CR &= ~AES_CR_EN) 00353 00354 /** 00355 * @brief Set the algorithm operating mode. 00356 * @param __OPERATING_MODE__: specifies the operating mode 00357 * This parameter can be one of the following values: 00358 * @arg CRYP_ALGOMODE_ENCRYPT: encryption 00359 * @arg CRYP_ALGOMODE_KEYDERIVATION: key derivation 00360 * @arg CRYP_ALGOMODE_DECRYPT: decryption 00361 * @arg CRYP_ALGOMODE_KEYDERIVATION_DECRYPT: key derivation and decryption 00362 * @retval None 00363 */ 00364 #define __HAL_CRYP_SET_OPERATINGMODE(__OPERATING_MODE__) MODIFY_REG(AES->CR, AES_CR_MODE, (__OPERATING_MODE__)) 00365 00366 00367 /** 00368 * @brief Set the algorithm chaining mode. 00369 * @param __CHAINING_MODE__: specifies the chaining mode 00370 * This parameter can be one of the following values: 00371 * @arg CRYP_CHAINMODE_AES_ECB: Electronic CodeBook 00372 * @arg CRYP_CHAINMODE_AES_CBC: Cipher Block Chaining 00373 * @arg CRYP_CHAINMODE_AES_CTR: CounTeR mode 00374 * @arg CRYP_CHAINMODE_AES_GCM_GMAC: Galois Counter Mode or Galois Message Authentication Code 00375 * @arg CRYP_CHAINMODE_AES_CMAC: Cipher Message Authentication Code 00376 * @retval None 00377 */ 00378 #define __HAL_CRYP_SET_CHAININGMODE(__CHAINING_MODE__) MODIFY_REG(AES->CR, AES_CR_CHMOD, (__CHAINING_MODE__)) 00379 00380 00381 00382 /** @brief Check whether the specified CRYP status flag is set or not. 00383 * @param __FLAG__: specifies the flag to check. 00384 * This parameter can be one of the following values: 00385 * @arg CRYP_FLAG_BUSY: GCM process suspension forbidden 00386 * @arg CRYP_IT_WRERR: Write Error 00387 * @arg CRYP_IT_RDERR: Read Error 00388 * @arg CRYP_IT_CCF: Computation Complete 00389 * @retval The state of __FLAG__ (TRUE or FALSE). 00390 */ 00391 #define __HAL_CRYP_GET_FLAG(__FLAG__) ((AES->SR & (__FLAG__)) == (__FLAG__)) 00392 00393 00394 /** @brief Clear the CRYP pending status flag. 00395 * @param __FLAG__: specifies the flag to clear. 00396 * This parameter can be one of the following values: 00397 * @arg CRYP_ERR_CLEAR: Read (RDERR) or Write Error (WRERR) Flag Clear 00398 * @arg CRYP_CCF_CLEAR: Computation Complete Flag (CCF) Clear 00399 * @retval None 00400 */ 00401 #define __HAL_CRYP_CLEAR_FLAG(__FLAG__) SET_BIT(AES->CR, (__FLAG__)) 00402 00403 00404 00405 /** @brief Check whether the specified CRYP interrupt source is enabled or not. 00406 * @param __INTERRUPT__: CRYP interrupt source to check 00407 * This parameter can be one of the following values: 00408 * @arg CRYP_IT_ERRIE: Error interrupt (used for RDERR and WRERR) 00409 * @arg CRYP_IT_CCFIE: Computation Complete interrupt 00410 * @retval State of interruption (TRUE or FALSE). 00411 */ 00412 #define __HAL_CRYP_GET_IT_SOURCE(__INTERRUPT__) ((AES->CR & (__INTERRUPT__)) == (__INTERRUPT__)) 00413 00414 00415 /** @brief Check whether the specified CRYP interrupt is set or not. 00416 * @param __INTERRUPT__: specifies the interrupt to check. 00417 * This parameter can be one of the following values: 00418 * @arg CRYP_IT_WRERR: Write Error 00419 * @arg CRYP_IT_RDERR: Read Error 00420 * @arg CRYP_IT_CCF: Computation Complete 00421 * @retval The state of __INTERRUPT__ (TRUE or FALSE). 00422 */ 00423 #define __HAL_CRYP_GET_IT(__INTERRUPT__) ((AES->SR & (__INTERRUPT__)) == (__INTERRUPT__)) 00424 00425 00426 00427 /** @brief Clear the CRYP pending interrupt. 00428 * @param __INTERRUPT__: specifies the IT to clear. 00429 * This parameter can be one of the following values: 00430 * @arg CRYP_ERR_CLEAR: Read (RDERR) or Write Error (WRERR) Flag Clear 00431 * @arg CRYP_CCF_CLEAR: Computation Complete Flag (CCF) Clear 00432 * @retval None 00433 */ 00434 #define __HAL_CRYP_CLEAR_IT(__INTERRUPT__) SET_BIT(AES->CR, (__INTERRUPT__)) 00435 00436 00437 /** 00438 * @brief Enable the CRYP interrupt. 00439 * @param __INTERRUPT__: CRYP Interrupt. 00440 * This parameter can be one of the following values: 00441 * @arg CRYP_IT_ERRIE: Error interrupt (used for RDERR and WRERR) 00442 * @arg CRYP_IT_CCFIE: Computation Complete interrupt 00443 * @retval None 00444 */ 00445 #define __HAL_CRYP_ENABLE_IT(__INTERRUPT__) ((AES->CR) |= (__INTERRUPT__)) 00446 00447 00448 /** 00449 * @brief Disable the CRYP interrupt. 00450 * @param __INTERRUPT__: CRYP Interrupt. 00451 * This parameter can be one of the following values: 00452 * @arg CRYP_IT_ERRIE: Error interrupt (used for RDERR and WRERR) 00453 * @arg CRYP_IT_CCFIE: Computation Complete interrupt 00454 * @retval None 00455 */ 00456 #define __HAL_CRYP_DISABLE_IT(__INTERRUPT__) ((AES->CR) &= ~(__INTERRUPT__)) 00457 00458 /** 00459 * @} 00460 */ 00461 00462 /* Private macros --------------------------------------------------------*/ 00463 /** @addtogroup CRYP_Private_Macros CRYP Private Macros 00464 * @{ 00465 */ 00466 00467 /** 00468 * @brief Verify the key size length. 00469 * @param __KEYSIZE__: Ciphering/deciphering algorithm key size. 00470 * @retval SET (__KEYSIZE__ is a valid value) or RESET (__KEYSIZE__ is invalid) 00471 */ 00472 #define IS_CRYP_KEYSIZE(__KEYSIZE__) (((__KEYSIZE__) == CRYP_KEYSIZE_128B) || \ 00473 ((__KEYSIZE__) == CRYP_KEYSIZE_256B)) 00474 00475 /** 00476 * @brief Verify the input data type. 00477 * @param __DATATYPE__: Ciphering/deciphering algorithm input data type. 00478 * @retval SET (__DATATYPE__ is valid) or RESET (__DATATYPE__ is invalid) 00479 */ 00480 #define IS_CRYP_DATATYPE(__DATATYPE__) (((__DATATYPE__) == CRYP_DATATYPE_32B) || \ 00481 ((__DATATYPE__) == CRYP_DATATYPE_16B) || \ 00482 ((__DATATYPE__) == CRYP_DATATYPE_8B) || \ 00483 ((__DATATYPE__) == CRYP_DATATYPE_1B)) 00484 00485 /** 00486 * @brief Verify the CRYP AES IP running mode. 00487 * @param __MODE__: CRYP AES IP running mode. 00488 * @retval SET (__MODE__ is valid) or RESET (__MODE__ is invalid) 00489 */ 00490 #define IS_CRYP_AES(__MODE__) (((__MODE__) == CRYP_AES_DISABLE) || \ 00491 ((__MODE__) == CRYP_AES_ENABLE)) 00492 00493 /** 00494 * @brief Verify the selected CRYP algorithm. 00495 * @param __ALGOMODE__: Selected CRYP algorithm (ciphering, deciphering, key derivation or a combination of the latter). 00496 * @retval SET (__ALGOMODE__ is valid) or RESET (__ALGOMODE__ is invalid) 00497 */ 00498 #define IS_CRYP_ALGOMODE(__ALGOMODE__) (((__ALGOMODE__) == CRYP_ALGOMODE_ENCRYPT) || \ 00499 ((__ALGOMODE__) == CRYP_ALGOMODE_KEYDERIVATION) || \ 00500 ((__ALGOMODE__) == CRYP_ALGOMODE_DECRYPT) || \ 00501 ((__ALGOMODE__) == CRYP_ALGOMODE_TAG_GENERATION) || \ 00502 ((__ALGOMODE__) == CRYP_ALGOMODE_KEYDERIVATION_DECRYPT)) 00503 00504 /** 00505 * @brief Verify the selected CRYP chaining algorithm. 00506 * @param __CHAINMODE__: Selected CRYP chaining algorithm. 00507 * @retval SET (__CHAINMODE__ is valid) or RESET (__CHAINMODE__ is invalid) 00508 */ 00509 #define IS_CRYP_CHAINMODE(__CHAINMODE__) (((__CHAINMODE__) == CRYP_CHAINMODE_AES_ECB) || \ 00510 ((__CHAINMODE__) == CRYP_CHAINMODE_AES_CBC) || \ 00511 ((__CHAINMODE__) == CRYP_CHAINMODE_AES_CTR) || \ 00512 ((__CHAINMODE__) == CRYP_CHAINMODE_AES_GCM_GMAC) || \ 00513 ((__CHAINMODE__) == CRYP_CHAINMODE_AES_CMAC)) 00514 00515 /** 00516 * @brief Verify the deciphering key write option. 00517 * @param __WRITE__: deciphering key write option. 00518 * @retval SET (__WRITE__ is valid) or RESET (__WRITE__ is invalid) 00519 */ 00520 #define IS_CRYP_WRITE(__WRITE__) (((__WRITE__) == CRYP_KEY_WRITE_ENABLE) || \ 00521 ((__WRITE__) == CRYP_KEY_WRITE_DISABLE)) 00522 00523 /** 00524 * @brief Verify the CRYP input data DMA mode. 00525 * @param __MODE__: CRYP input data DMA mode. 00526 * @retval SET (__MODE__ is valid) or RESET (__MODE__ is invalid) 00527 */ 00528 #define IS_CRYP_DMAIN(__MODE__) (((__MODE__) == CRYP_DMAIN_DISABLE) || \ 00529 ((__MODE__) == CRYP_DMAIN_ENABLE)) 00530 00531 /** 00532 * @brief Verify the CRYP output data DMA mode. 00533 * @param __MODE__: CRYP output data DMA mode. 00534 * @retval SET (__MODE__ is valid) or RESET (__MODE__ is invalid) 00535 */ 00536 #define IS_CRYP_DMAOUT(__MODE__) (((__MODE__) == CRYP_DMAOUT_DISABLE) || \ 00537 ((__MODE__) == CRYP_DMAOUT_ENABLE)) 00538 00539 /** 00540 * @brief Verify the CRYP AES ciphering/deciphering/authentication algorithm phase. 00541 * @param __PHASE__: CRYP AES ciphering/deciphering/authentication algorithm phase. 00542 * @retval SET (__PHASE__ is valid) or RESET (__PHASE__ is invalid) 00543 */ 00544 #define IS_CRYP_GCMCMAC_PHASE(__PHASE__) (((__PHASE__) == CRYP_GCM_INIT_PHASE) || \ 00545 ((__PHASE__) == CRYP_GCMCMAC_HEADER_PHASE) || \ 00546 ((__PHASE__) == CRYP_GCM_PAYLOAD_PHASE) || \ 00547 ((__PHASE__) == CRYP_GCMCMAC_FINAL_PHASE)) 00548 00549 /** 00550 * @} 00551 */ 00552 00553 /* Include CRYP HAL Extended module */ 00554 #include "stm32l4xx_hal_cryp_ex.h" 00555 00556 /* Exported functions --------------------------------------------------------*/ 00557 /** @addtogroup CRYP_Exported_Functions CRYP Exported Functions 00558 * @{ 00559 */ 00560 00561 /** @addtogroup CRYP_Group1 Initialization and deinitialization functions 00562 * @{ 00563 */ 00564 00565 /* Initialization/de-initialization functions ********************************/ 00566 HAL_StatusTypeDef HAL_CRYP_Init(CRYP_HandleTypeDef *hcryp); 00567 HAL_StatusTypeDef HAL_CRYP_DeInit(CRYP_HandleTypeDef *hcryp); 00568 00569 /* MSP initialization/de-initialization functions ****************************/ 00570 void HAL_CRYP_MspInit(CRYP_HandleTypeDef *hcryp); 00571 void HAL_CRYP_MspDeInit(CRYP_HandleTypeDef *hcryp); 00572 00573 /** 00574 * @} 00575 */ 00576 00577 /** @addtogroup CRYP_Group2 AES processing functions 00578 * @{ 00579 */ 00580 00581 /* AES encryption/decryption processing functions ****************************/ 00582 00583 /* AES encryption/decryption using polling ***********************************/ 00584 HAL_StatusTypeDef HAL_CRYP_AESECB_Encrypt(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData, uint32_t Timeout); 00585 HAL_StatusTypeDef HAL_CRYP_AESECB_Decrypt(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData, uint32_t Timeout); 00586 HAL_StatusTypeDef HAL_CRYP_AESCBC_Encrypt(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData, uint32_t Timeout); 00587 HAL_StatusTypeDef HAL_CRYP_AESCBC_Decrypt(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData, uint32_t Timeout); 00588 HAL_StatusTypeDef HAL_CRYP_AESCTR_Encrypt(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData, uint32_t Timeout); 00589 HAL_StatusTypeDef HAL_CRYP_AESCTR_Decrypt(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData, uint32_t Timeout); 00590 00591 /* AES encryption/decryption using interrupt *********************************/ 00592 HAL_StatusTypeDef HAL_CRYP_AESECB_Encrypt_IT(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData); 00593 HAL_StatusTypeDef HAL_CRYP_AESCBC_Encrypt_IT(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData); 00594 HAL_StatusTypeDef HAL_CRYP_AESCTR_Encrypt_IT(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData); 00595 HAL_StatusTypeDef HAL_CRYP_AESECB_Decrypt_IT(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData); 00596 HAL_StatusTypeDef HAL_CRYP_AESCTR_Decrypt_IT(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData); 00597 HAL_StatusTypeDef HAL_CRYP_AESCBC_Decrypt_IT(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData); 00598 00599 /* AES encryption/decryption using DMA ***************************************/ 00600 HAL_StatusTypeDef HAL_CRYP_AESECB_Encrypt_DMA(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData); 00601 HAL_StatusTypeDef HAL_CRYP_AESECB_Decrypt_DMA(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData); 00602 HAL_StatusTypeDef HAL_CRYP_AESCBC_Encrypt_DMA(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData); 00603 HAL_StatusTypeDef HAL_CRYP_AESCBC_Decrypt_DMA(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData); 00604 HAL_StatusTypeDef HAL_CRYP_AESCTR_Encrypt_DMA(CRYP_HandleTypeDef *hcryp, uint8_t *pPlainData, uint16_t Size, uint8_t *pCypherData); 00605 HAL_StatusTypeDef HAL_CRYP_AESCTR_Decrypt_DMA(CRYP_HandleTypeDef *hcryp, uint8_t *pCypherData, uint16_t Size, uint8_t *pPlainData); 00606 00607 /** 00608 * @} 00609 */ 00610 00611 /** @addtogroup CRYP_Group3 Callback functions 00612 * @{ 00613 */ 00614 /* CallBack functions ********************************************************/ 00615 void HAL_CRYP_InCpltCallback(CRYP_HandleTypeDef *hcryp); 00616 void HAL_CRYP_OutCpltCallback(CRYP_HandleTypeDef *hcryp); 00617 void HAL_CRYP_ErrorCallback(CRYP_HandleTypeDef *hcryp); 00618 00619 /** 00620 * @} 00621 */ 00622 00623 /** @addtogroup CRYP_Group4 CRYP IRQ handler 00624 * @{ 00625 */ 00626 00627 /* AES interrupt handling function *******************************************/ 00628 void HAL_CRYP_IRQHandler(CRYP_HandleTypeDef *hcryp); 00629 00630 /** 00631 * @} 00632 */ 00633 00634 /** @addtogroup CRYP_Group5 Peripheral State functions 00635 * @{ 00636 */ 00637 00638 /* Peripheral State functions ************************************************/ 00639 HAL_CRYP_STATETypeDef HAL_CRYP_GetState(CRYP_HandleTypeDef *hcryp); 00640 uint32_t HAL_CRYP_GetError(CRYP_HandleTypeDef *hcryp); 00641 00642 /** 00643 * @} 00644 */ 00645 00646 /** 00647 * @} 00648 */ 00649 00650 /** 00651 * @} 00652 */ 00653 00654 /** 00655 * @} 00656 */ 00657 00658 #endif /* defined(STM32L485xx) || defined(STM32L486xx) */ 00659 00660 #ifdef __cplusplus 00661 } 00662 #endif 00663 00664 #endif /* __STM32L4xx_HAL_CRYP_H */ 00665 00666 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00667
Generated on Tue Jul 12 2022 10:58:09 by
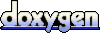