Library used to control ST Nucleo Evaluation board IHM04A1, based on L6206 motor control driver.
Dependencies: ST_INTERFACES
Dependents: HelloWorld_IHM04A1 RoboCane_Motore arm_dcmotor_can arm_linear_can_2 ... more
Fork of X_NUCLEO_IHM04A1 by
motor_def.h
00001 /** 00002 ****************************************************************************** 00003 * @file motor_def.h 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date January 25, 2016 00007 * @brief This file contains all the functions prototypes for motor drivers. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __MOTOR_H 00040 #define __MOTOR_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include <stdint.h> 00048 #include "component_def.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup Components 00055 * @{ 00056 */ 00057 00058 /** @defgroup Motor Motor 00059 * @{ 00060 */ 00061 00062 /** @defgroup Motor_Exported_Constants Motor Exported Constants 00063 * @{ 00064 */ 00065 00066 /// boolean for false condition 00067 #ifndef FALSE 00068 #define FALSE (0) 00069 #endif 00070 /// boolean for true condition 00071 #ifndef TRUE 00072 #define TRUE (1) 00073 #endif 00074 00075 /** 00076 * @} 00077 */ 00078 00079 /** @defgroup Motor_Exported_Types Motor Exported Types 00080 * @{ 00081 */ 00082 00083 /** @defgroup Motor_Boolean_Type Motor Boolean Type 00084 * @{ 00085 */ 00086 ///bool Type 00087 //typedef uint8_t bool; 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup Device_Direction_Options Device Direction Options 00093 * @{ 00094 */ 00095 /// Direction options 00096 typedef enum { 00097 BACKWARD = 0, 00098 FORWARD = 1, 00099 UNKNOW_DIR = ((uint8_t)0xFF) 00100 } motorDir_t; 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup Device_Action_Options Device Action Options 00106 * @{ 00107 */ 00108 /// Action options 00109 typedef enum { 00110 ACTION_RESET = ((uint8_t)0x00), 00111 ACTION_COPY = ((uint8_t)0x08) 00112 } motorAction_t; 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup Device_States Device States 00118 * @{ 00119 */ 00120 /// Device states 00121 typedef enum { 00122 ACCELERATING = 0, 00123 DECELERATINGTOSTOP = 1, 00124 DECELERATING = 2, 00125 STEADY = 3, 00126 INDEX_ACCEL = 4, 00127 INDEX_RUN = 5, 00128 INDEX_DECEL = 6, 00129 INDEX_DWELL = 7, 00130 INACTIVE = 8, 00131 STANDBY = 9, 00132 STANDBYTOINACTIVE = 10 00133 } motorState_t; 00134 /** 00135 * @} 00136 */ 00137 00138 /** @defgroup Device_Step_mode Device Step mode 00139 * @{ 00140 */ 00141 /// Stepping options 00142 typedef enum { 00143 STEP_MODE_FULL = ((uint8_t)0x00), 00144 STEP_MODE_HALF = ((uint8_t)0x01), 00145 STEP_MODE_1_4 = ((uint8_t)0x02), 00146 STEP_MODE_1_8 = ((uint8_t)0x03), 00147 STEP_MODE_1_16 = ((uint8_t)0x04), 00148 STEP_MODE_1_32 = ((uint8_t)0x05), 00149 STEP_MODE_1_64 = ((uint8_t)0x06), 00150 STEP_MODE_1_128 = ((uint8_t)0x07), 00151 STEP_MODE_1_256 = ((uint8_t)0x08), 00152 STEP_MODE_UNKNOW = ((uint8_t)0xFE), 00153 STEP_MODE_WAVE = ((uint8_t)0xFF) 00154 } motorStepMode_t; 00155 00156 /** 00157 * @} 00158 */ 00159 00160 /** @defgroup Decay_mode Decay mode 00161 * @{ 00162 */ 00163 /// Decay Mode 00164 typedef enum { 00165 SLOW_DECAY = 0, 00166 FAST_DECAY = 1, 00167 UNKNOW_DECAY = ((uint8_t)0xFF) 00168 } motorDecayMode_t; 00169 /** 00170 * @} 00171 */ 00172 00173 /** @defgroup Stop_mode Stop mode 00174 * @{ 00175 */ 00176 /// Stop mode 00177 typedef enum 00178 { 00179 HOLD_MODE = 0, 00180 HIZ_MODE = 1, 00181 STANDBY_MODE = 2, 00182 UNKNOW_STOP_MODE = ((uint8_t)0xFF) 00183 } motorStopMode_t; 00184 /** 00185 * @} 00186 */ 00187 00188 /** @defgroup Torque_mode Torque mode 00189 * @{ 00190 */ 00191 /// Torque mode 00192 typedef enum 00193 { 00194 ACC_TORQUE = 0, 00195 DEC_TORQUE = 1, 00196 RUN_TORQUE = 2, 00197 HOLD_TORQUE = 3, 00198 CURRENT_TORQUE = 4, 00199 UNKNOW_TORQUE = ((uint8_t)0xFF) 00200 } motorTorqueMode_t; 00201 /** 00202 * @} 00203 */ 00204 00205 /** @defgroup Dual_Full_Bridge_Configuration Dual Full Bridge Configuration 00206 * @{ 00207 */ 00208 ///Dual full bridge configurations for brush DC motors 00209 typedef enum { 00210 PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B = 0, 00211 PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B = 1, 00212 PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B = 2, 00213 PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B = 3, 00214 PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B = 4, 00215 PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B = 5, 00216 PARALLELING_IN1B_IN2B__1_BIDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B = 6, 00217 PARALLELING_IN1B_IN2B__2_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B = 7, 00218 PARALLELING_IN1A_IN2A__IN1B_IN2B__1_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B = 8, 00219 PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR = 9, 00220 PARALLELING_IN1A_IN1B__IN2A_IN2B__1_UNDIR_MOTOR_BRIDGE_1A__1_UNDIR_MOTOR_BRIDGE_2A = 10, 00221 PARALLELING_IN1A_IN1B__IN2A_IN2B__1_BIDIR_MOTOR = 11, 00222 PARALLELING_ALL_WITH_IN1A___1_UNDIR_MOTOR = 12, 00223 PARALLELING_END_ENUM = 13 00224 } dualFullBridgeConfig_t; 00225 /** 00226 * @} 00227 */ 00228 00229 /** @defgroup Motor_Driver_Structure Motor Driver Structure 00230 * @{ 00231 */ 00232 /// Motor driver structure definition 00233 typedef struct 00234 { 00235 00236 /* Generic */ 00237 status_t (*init)(void *handle, void *init); 00238 status_t (*read_id)(void *handle, uint8_t *id); 00239 00240 /* Interrupts */ 00241 /// Function pointer to attach_error_handler 00242 void(*attach_error_handler)(void *handle, void (*callback)(void *handle, uint16_t error)); 00243 /// Function pointer to attach_flag_interrupt 00244 void (*attach_flag_interrupt)(void *handle, void (*callback)(void *handle)); 00245 /// Function pointer to AttachBusyInterrupt 00246 void (*AttachBusyInterrupt)(void *handle, void (*callback)(void *handle)); 00247 /// Function pointer to FlagInterruptHandler 00248 void (*FlagInterruptHandler)(void *handle); 00249 00250 /* Specific */ 00251 /// Function pointer to get_acceleration 00252 uint16_t (*get_acceleration)(void *handle); 00253 /// Function pointer to GetCurrentSpeed 00254 uint16_t (*GetCurrentSpeed)(void *handle, uint8_t motorId); 00255 /// Function pointer to get_deceleration 00256 uint16_t (*get_deceleration)(void *handle); 00257 /// Function pointer to get_device_state 00258 motorState_t(*get_device_state)(void *handle, uint8_t motorId); 00259 /// Function pointer to get_fw_version 00260 uint8_t (*get_fw_version)(void *handle); 00261 /// Function pointer to get_mark 00262 int32_t (*get_mark)(void *handle); 00263 /// Function pointer to get_max_speed 00264 uint16_t (*get_max_speed)(void *handle, uint8_t motorId); 00265 /// Function pointer to get_min_speed 00266 uint16_t (*get_min_speed)(void *handle, uint8_t motorId); 00267 /// Function pointer to get_position 00268 int32_t (*get_position)(void *handle); 00269 /// Function pointer to go_home 00270 void (*go_home)(void *handle); 00271 /// Function pointer to go_mark 00272 void (*go_mark)(void *handle); 00273 /// Function pointer to go_to 00274 void (*go_to)(void *handle, uint8_t deviceId, int32_t motorId); 00275 /// Function pointer to hard_stop 00276 void (*hard_stop)(void *handle, uint8_t motorId); 00277 /// Function pointer to move 00278 void (*move)(void *handle, motorDir_t direction, uint32_t stepCount); 00279 /// Function pointer to ResetAllDevices 00280 void (*ResetAllDevices)(void *handle); 00281 /// Function pointer to run 00282 void (*run)(void *handle, uint8_t stepCount, motorDir_t direction); 00283 /// Function pointer to set_acceleration 00284 bool(*set_acceleration)(void *handle, uint16_t newAcc); 00285 /// Function pointer to set_deceleration 00286 bool(*set_deceleration)(void *handle, uint16_t newDec); 00287 /// Function pointer to set_home 00288 void (*set_home)(void *handle); 00289 /// Function pointer to set_mark 00290 void (*set_mark)(void *handle); 00291 /// Function pointer to set_max_speed 00292 bool (*set_max_speed)(void *handle, uint8_t motorId, uint16_t newMaxSpeed); 00293 /// Function pointer to set_min_speed 00294 bool (*set_min_speed)(void *handle, uint8_t motorId, uint16_t newMinSpeed); 00295 /// Function pointer to soft_stop 00296 bool (*soft_stop)(void *handle); 00297 /// Function pointer to StepClockHandler 00298 void (*StepClockHandler)(void *handle); 00299 /// Function pointer to wait_while_active 00300 void (*wait_while_active)(void *handle); 00301 /// Function pointer to CmdDisable 00302 void (*CmdDisable)(void *handle, uint8_t bridgeId); 00303 /// Function pointer to CmdEnable 00304 void (*CmdEnable)(void *handle, uint8_t bridgeId); 00305 /// Function pointer to CmdGetParam 00306 uint32_t (*CmdGetParam)(void *handle, uint32_t param); 00307 /// Function pointer to CmdGetStatus 00308 uint16_t (*CmdGetStatus)(void *handle, uint8_t bridgeId); 00309 /// Function pointer to CmdNop 00310 void (*CmdNop)(void *handle); 00311 /// Function pointer to CmdSetParam 00312 void (*CmdSetParam)(void *handle, uint32_t param, uint32_t value); 00313 /// Function pointer to read_status_register 00314 uint16_t (*read_status_register)(void *handle); 00315 /// Function pointer to release_reset 00316 void (*release_reset)(void *handle); 00317 /// Function pointer to Reset 00318 void (*Reset)(void *handle); 00319 /// Function pointer to SelectStepMode 00320 void (*SelectStepMode)(void *handle, motorStepMode_t stepMode); 00321 /// Function pointer to set_direction 00322 void (*set_direction)(void *handle, motorDir_t direction); 00323 /// Function pointer to CmdGoToDir 00324 void (*CmdGoToDir)(void *handle, motorDir_t direction, int32_t abs_pos); 00325 /// Function pointer to check_busy_hw 00326 uint8_t (*check_busy_hw)(void *handle); 00327 /// Function pointer to check_status_hw 00328 uint8_t (*check_status_hw)(void *handle); 00329 /// Function pointer to CmdGoUntil 00330 void (*CmdGoUntil)(void *handle, motorAction_t action, motorDir_t direction, uint32_t speed); 00331 /// Function pointer to CmdHardHiZ 00332 void (*CmdHardHiZ)(void *handle, uint8_t motorId); 00333 /// Function pointer to CmdReleaseSw 00334 void (*CmdReleaseSw)(void *handle, motorAction_t action, motorDir_t direction); 00335 /// Function pointer to CmdResetDevice 00336 void (*CmdResetDevice)(void *handle); 00337 /// Function pointer to CmdResetPos 00338 void (*CmdResetPos)(void *handle); 00339 /// Function pointer to CmdRun 00340 void (*CmdRun)(void *handle, motorDir_t direction, uint32_t speed); 00341 /// Function pointer to CmdSoftHiZ 00342 void (*CmdSoftHiZ)(void *handle); 00343 /// Function pointer to CmdStepClock 00344 void (*CmdStepClock)(void *handle, motorDir_t direction); 00345 /// Function pointer to fetch_and_clear_all_status 00346 void (*fetch_and_clear_all_status)(void *handle); 00347 /// Function pointer to get_fetched_status 00348 uint16_t (*get_fetched_status)(void *handle); 00349 /// Function pointer to get_nb_devices 00350 uint8_t (*get_nb_devices)(void *handle); 00351 /// Function pointer to is_device_busy 00352 bool (*is_device_busy)(void *handle); 00353 /// Function pointer to send_queued_commands 00354 void (*send_queued_commands)(void *handle); 00355 /// Function pointer to queue_commands 00356 void (*queue_commands)(void *handle, uint8_t param, int32_t value); 00357 /// Function pointer to WaitForAllDevicesNotBusy 00358 void (*WaitForAllDevicesNotBusy)(void *handle); 00359 /// Function pointer to error_handler 00360 void (*error_handler)(void *handle, uint16_t error); 00361 /// Function pointer to BusyInterruptHandler 00362 void (*BusyInterruptHandler)(void *handle); 00363 /// Function pointer to CmdSoftStop 00364 void (*CmdSoftStop)(void *handle); 00365 /// Function pointer to StartStepClock 00366 void (*StartStepClock)(void *handle); 00367 /// Function pointer to StopStepClock 00368 void (*StopStepClock)(void *handle); 00369 /// Function pointer to set_dual_full_bridge_config 00370 void (*set_dual_full_bridge_config)(void *handle, uint8_t newConfig); 00371 /// Function pointer to get_bridge_input_pwm_freq 00372 uint32_t (*get_bridge_input_pwm_freq)(void *handle, uint8_t bridgeId); 00373 /// Function pointer to set_bridge_input_pwm_freq 00374 void (*set_bridge_input_pwm_freq)(void *handle, uint8_t bridgeId, uint32_t newFreq); 00375 /// Function pointer to set_stop_mode 00376 void (*set_stop_mode)(void *handle, motorStopMode_t stopMode); 00377 /// Function pointer to get_stop_mode 00378 motorStopMode_t (*get_stop_mode)(void *handle); 00379 /// Function pointer to set_decay_mode 00380 void (*set_decay_mode)(void *handle, motorDecayMode_t decayMode); 00381 /// Function pointer to get_decay_mode 00382 motorDecayMode_t (*get_decay_mode)(void *handle); 00383 /// Function pointer to get_step_mode 00384 motorStepMode_t (*get_step_mode)(void *handle); 00385 /// Function pointer to get_direction 00386 motorDir_t (*get_direction)(void *handle); 00387 /// Function pointer to ExitDeviceFromReset 00388 void (*ExitDeviceFromReset)(void *handle); 00389 /// Function pointer to set_torque 00390 void (*set_torque)(void *handle, motorTorqueMode_t torqueMode, uint8_t torqueValue); 00391 /// Function pointer to get_torque 00392 uint8_t (*get_torque)(void *handle, motorTorqueMode_t torqueMode); 00393 /// Function pointer to SetVRefFreq 00394 void (*SetRefFreq)(void *handle, uint32_t freq); 00395 /// Function pointer to GetVRefFreq 00396 uint32_t (*GetRefFreq)(void *handle); 00397 /// Function pointer to SetVRefDc 00398 void (*SetRefDc)(void *handle, uint8_t RefDc); 00399 /// Function pointer to GetVRefDc 00400 uint8_t (*GetRefDc)(void *handle); 00401 /// Function pointer to set_nb_devices 00402 bool (*set_nb_devices)(void *handle, uint8_t nbDevices); 00403 /// Function pointer to set a parameter 00404 bool (*set_analog_value)(void *handle, uint32_t param, float value); 00405 /// Function pointer to get a parameter 00406 float (*get_analog_value)(void *handle, uint32_t param); 00407 } MOTOR_VTable_t; 00408 00409 /** 00410 00411 * @} 00412 */ 00413 00414 /** 00415 * @} 00416 */ 00417 00418 /** 00419 * @} 00420 */ 00421 00422 /** 00423 * @} 00424 */ 00425 00426 /** 00427 * @} 00428 */ 00429 00430 #ifdef __cplusplus 00431 } 00432 #endif 00433 00434 #endif /* __MOTOR_H */ 00435 00436 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00437
Generated on Fri Jul 15 2022 19:41:39 by
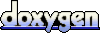