Library used to control ST Nucleo Evaluation board IHM04A1, based on L6206 motor control driver.
Dependencies: ST_INTERFACES
Dependents: HelloWorld_IHM04A1 RoboCane_Motore arm_dcmotor_can arm_linear_can_2 ... more
Fork of X_NUCLEO_IHM04A1 by
L6206_def.h
00001 /** 00002 ****************************************************************************** 00003 * @file L6206_def.h 00004 * @author IPC Rennes 00005 * @version V1.2.0 00006 * @date March 30, 2016 00007 * @brief Header for L6206 driver (dual full bridge driver) 00008 * @note (C) COPYRIGHT 2015 STMicroelectronics 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __L6206_H 00041 #define __L6206_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "L6206_config.h" 00049 #include "../Common/motor_def.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup Components 00056 * @{ 00057 */ 00058 00059 /** @addtogroup L6206 00060 * @{ 00061 */ 00062 00063 /* Exported Constants --------------------------------------------------------*/ 00064 00065 /** @defgroup L6206_Exported_Defines L6206 Exported Defines 00066 * @{ 00067 */ 00068 00069 /// Current FW version 00070 #define L6206_FW_VERSION (0) 00071 ///Max number of Brush DC motors 00072 #define L6206_NB_MAX_MOTORS (4) 00073 ///Max number of Bridges 00074 #define L6206_NB_MAX_BRIDGES (2) 00075 ///Max number of input of Bridges 00076 #define L6206_NB_MAX_BRIDGE_INPUT (2 * L6206_NB_MAX_BRIDGES) 00077 /// MCU wait time in ms after power bridges are enabled 00078 #define BSP_MOTOR_CONTROL_BOARD_BRIDGE_TURN_ON_DELAY (20) 00079 00080 /** 00081 * @} 00082 */ 00083 00084 00085 /* Exported Types -------------------------------------------------------*/ 00086 00087 /** @defgroup L6206_Exported_Types L6206 Exported Types 00088 * @{ 00089 */ 00090 00091 00092 00093 00094 /** @defgroup Initialization_Structure Initialization Structure 00095 * @{ 00096 */ 00097 00098 typedef struct 00099 { 00100 /// Bridge configuration structure. 00101 dualFullBridgeConfig_t config; 00102 00103 /// PWM frequency 00104 uint32_t pwmFreq[L6206_NB_MAX_BRIDGE_INPUT]; 00105 00106 /// Motor speed 00107 uint16_t speed[L6206_NB_MAX_MOTORS]; 00108 00109 /// Motor direction 00110 motorDir_t direction[L6206_NB_MAX_MOTORS]; 00111 00112 /// Current motor state 00113 motorState_t motionState[L6206_NB_MAX_MOTORS]; 00114 00115 /// Bridge enabled (true) or not (false) 00116 bool bridgeEnabled[L6206_NB_MAX_BRIDGES]; 00117 } deviceParams_t; 00118 00119 00120 00121 00122 typedef deviceParams_t L6206_init_t; 00123 00124 00125 00126 /** 00127 * @} 00128 */ 00129 00130 00131 /** @defgroup Data_Structure Data Structure 00132 * @{ 00133 */ 00134 00135 /* ACTION --------------------------------------------------------------------* 00136 * Declare here the structure of component's data, if any, one variable per * 00137 * line without initialization. * 00138 * * 00139 * Example: * 00140 * typedef struct * 00141 * { * 00142 * int T0_out; * 00143 * int T1_out; * 00144 * float T0_degC; * 00145 * float T1_degC; * 00146 * } COMPONENT_Data_t; * 00147 *----------------------------------------------------------------------------*/ 00148 typedef struct 00149 { 00150 /// Function pointer to flag interrupt call back 00151 void (*flagInterruptCallback)(void); 00152 00153 /// Function pointer to error handler call back 00154 void (*errorHandlerCallback)(uint16_t error); 00155 00156 /// number of L6206 devices 00157 uint8_t numberOfDevices; 00158 00159 /// L6206 driver instance 00160 uint8_t deviceInstance; 00161 00162 /// L6206 Device Paramaters structure 00163 deviceParams_t devicePrm; 00164 } L6206_Data_t; 00165 00166 /** 00167 * @} 00168 */ 00169 00170 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /* Exported functions --------------------------------------------------------*/ 00177 00178 00179 /** @defgroup MotorControl_Board_Linked_Functions MotorControl Board Linked Functions 00180 * @{ 00181 */ 00182 ///Delay of the requested number of milliseconds 00183 extern void L6206_Board_Delay(void *handle, uint32_t delay); 00184 ///Disable the specified bridge 00185 extern void L6206_Board_DisableBridge(void *handle, uint8_t bridgeId); 00186 ///Enable the specified bridge 00187 extern void L6206_Board_EnableBridge(void *handle, uint8_t bridgeId, uint8_t addDelay); 00188 //Get the status of the flag and enable Pin 00189 extern uint32_t L6206_Board_GetFlagPinState(void *handle, uint8_t bridgeId); 00190 ///Initialise GPIOs used for L6206s 00191 extern void L6206_Board_GpioInit(void *handle); 00192 ///Set Briges Inputs PWM frequency and start it 00193 extern void L6206_Board_PwmSetFreq(void *handle, uint8_t bridgeInput, uint32_t newFreq, uint8_t duty); 00194 ///Deinitialise the PWM of the specified bridge input 00195 extern void L6206_Board_PwmDeInit(void *handle, uint8_t bridgeInput); 00196 ///init the PWM of the specified bridge input 00197 extern void L6206_Board_PwmInit(void *handle, uint8_t bridgeInput); 00198 ///Stop the PWM of the specified bridge input 00199 extern void L6206_Board_PwmStop(void *handle, uint8_t bridgeInput); 00200 /** 00201 * @} 00202 */ 00203 00204 00205 00206 /** 00207 * @} 00208 */ 00209 00210 00211 /** 00212 * @} 00213 */ 00214 00215 /** 00216 * @} 00217 */ 00218 00219 #ifdef __cplusplus 00220 } 00221 #endif 00222 00223 #endif /* #ifndef __L6206_H */ 00224 00225 00226 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00227 00228
Generated on Fri Jul 15 2022 19:41:39 by
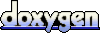