Library used to control ST Nucleo Evaluation board IHM04A1, based on L6206 motor control driver.
Dependencies: ST_INTERFACES
Dependents: HelloWorld_IHM04A1 RoboCane_Motore arm_dcmotor_can arm_linear_can_2 ... more
Fork of X_NUCLEO_IHM04A1 by
L6206.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file L6206.cpp 00004 * @author IPC Rennes 00005 * @version V1.1.0 00006 * @date March 02, 2016 00007 * @brief L6206 driver (dual full bridge driver) 00008 * @note (C) COPYRIGHT 2015 STMicroelectronics 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Generated with STM32CubeTOO -----------------------------------------------*/ 00041 00042 00043 /* Revision ------------------------------------------------------------------*/ 00044 /* 00045 Repository: http://svn.x-nucleodev.codex.cro.st.com/svnroot/X-NucleoDev 00046 Branch/Trunk/Tag: trunk 00047 Based on: X-CUBE-SPN4/trunk/Drivers/BSP/Components/L6206/L6206.c 00048 Revision: 0 00049 */ 00050 00051 /* Includes ------------------------------------------------------------------*/ 00052 #include "L6206.h" 00053 #include "string.h" 00054 00055 00056 /** @addtogroup BSP 00057 * @{ 00058 */ 00059 00060 /** @addtogroup Components 00061 * @{ 00062 */ 00063 00064 /** @addtogroup L6206 00065 * @{ 00066 */ 00067 00068 /* Private constants ---------------------------------------------------------*/ 00069 00070 00071 /* Private variables ---------------------------------------------------------*/ 00072 00073 /** @defgroup L6206_Private_Variables L6206 Private Variables 00074 * @{ 00075 */ 00076 static uint8_t l6206ArrayNbMaxMotorsByConfig[PARALLELING_END_ENUM] = {2,3,3,4,2,3,2,3,2,1,2,1,1}; 00077 /** 00078 * @} 00079 */ 00080 00081 /* Private constant ---------------------------------------------------------*/ 00082 00083 00084 /* Public Function prototypes -----------------------------------------------*/ 00085 00086 00087 00088 /* Private function prototypes -----------------------------------------------*/ 00089 00090 /** @defgroup L6206_Private_functions L6206 Private functions 00091 * @{ 00092 */ 00093 00094 00095 /******************************************************//** 00096 * @brief Attaches a user callback to the error Handler. 00097 * The call back will be then called each time the library 00098 * detects an error 00099 * @param[in] callback Name of the callback to attach 00100 * to the error Hanlder 00101 * @retval None 00102 **********************************************************/ 00103 void L6206::L6206_AttachErrorHandler(void (*callback)(uint16_t error)) 00104 { 00105 errorHandlerCallback = (void (*)(uint16_t error)) callback; 00106 } 00107 00108 /******************************************************//** 00109 * @brief Attaches a user callback to the flag Interrupt 00110 * The call back will be then called each time the status 00111 * flag pin will be pulled down due to the occurrence of 00112 * a programmed alarms ( OCD, thermal alert) 00113 * @param[in] callback Name of the callback to attach 00114 * to the Flag Interrupt 00115 * @retval None 00116 **********************************************************/ 00117 void L6206::L6206_attach_flag_interrupt(void (*callback)(void)) 00118 { 00119 flagInterruptCallback = (void (*)(void))callback; 00120 } 00121 00122 /******************************************************//** 00123 * @brief Disable the specified bridge 00124 * @param[in] bridgeId (from 0 for bridge A to 1 for bridge B) 00125 * @retval None 00126 * @note When input of different brigdes are parallelized 00127 * together, the disabling of one bridge leads to the disabling 00128 * of the second one 00129 **********************************************************/ 00130 void L6206::L6206_DisableBridge(uint8_t bridgeId) 00131 { 00132 L6206_Board_DisableBridge(bridgeId); 00133 00134 devicePrm.bridgeEnabled[bridgeId] = FALSE; 00135 if (devicePrm.config >= PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR) 00136 { 00137 if (bridgeId == BRIDGE_A) 00138 { 00139 L6206_Board_DisableBridge(BRIDGE_B); 00140 devicePrm.bridgeEnabled[BRIDGE_B] = FALSE; 00141 } 00142 else 00143 { 00144 L6206_Board_DisableBridge(BRIDGE_A); 00145 devicePrm.bridgeEnabled[BRIDGE_A] = FALSE; 00146 } 00147 } 00148 } 00149 00150 /******************************************************//** 00151 * @brief Enable the specified bridge 00152 * @param[in] bridgeId (from 0 for bridge A to 1 for bridge B) 00153 * @retval None 00154 * @note When input of different brigdes are parallelized 00155 * together, the enabling of one bridge leads to the enabling 00156 * of the second one 00157 **********************************************************/ 00158 void L6206::L6206_EnableBridge(uint8_t bridgeId) 00159 { 00160 devicePrm.bridgeEnabled[bridgeId] = TRUE; 00161 if (devicePrm.config >= PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR) 00162 { 00163 L6206_Board_EnableBridge(bridgeId, 0); 00164 if (bridgeId == BRIDGE_A) 00165 { 00166 L6206_Board_EnableBridge(BRIDGE_B, 1); 00167 devicePrm.bridgeEnabled[BRIDGE_B] = TRUE; 00168 } 00169 else 00170 { 00171 L6206_Board_EnableBridge(BRIDGE_A, 1); 00172 devicePrm.bridgeEnabled[BRIDGE_A] = TRUE; 00173 } 00174 } 00175 else 00176 { 00177 L6206_Board_EnableBridge(bridgeId, 1); 00178 } 00179 } 00180 00181 /******************************************************//** 00182 * @brief Start the L6206 library 00183 * @param[in] init pointer to the initialization data 00184 * @retval None 00185 **********************************************************/ 00186 status_t L6206::L6206_Init(void *init) 00187 { 00188 deviceInstance++; 00189 00190 /* Initialise the GPIOs */ 00191 L6206_Board_GpioInit(); 00192 00193 if (init == NULL) 00194 { 00195 /* Set context variables to the predefined values from l6206_target_config.h */ 00196 /* Set GPIO according to these values */ 00197 L6206_SetDeviceParamsToPredefinedValues(); 00198 } 00199 else 00200 { 00201 L6206_SetDeviceParamsToGivenValues((L6206_init_t*) init); 00202 } 00203 00204 /* Initialise input bridges PWMs */ 00205 L6206_SetDualFullBridgeConfig(devicePrm.config); 00206 00207 return COMPONENT_OK; 00208 } 00209 00210 /******************************************************//** 00211 * @brief Get the PWM frequency of the specified bridge 00212 * @param[in] bridgeId 0 for bridge A, 1 for bridge B 00213 * @retval Freq in Hz 00214 **********************************************************/ 00215 uint32_t L6206::L6206_GetBridgeInputPwmFreq(uint8_t bridgeId) 00216 { 00217 return (devicePrm.pwmFreq[(bridgeId << 1)]); 00218 } 00219 00220 /******************************************************//** 00221 * @brief Returns the current speed of the specified motor 00222 * @param[in] motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00223 * @retval current speed in % from 0 to 100 00224 **********************************************************/ 00225 uint16_t L6206::L6206_GetCurrentSpeed(uint8_t motorId) 00226 { 00227 uint16_t speed = 0; 00228 00229 if (motorId > l6206ArrayNbMaxMotorsByConfig[devicePrm.config]) 00230 { 00231 L6206_ErrorHandler(L6206_ERROR_1); 00232 } 00233 else 00234 { 00235 if (devicePrm.motionState[motorId] != INACTIVE) 00236 { 00237 speed = devicePrm.speed[motorId]; 00238 } 00239 } 00240 00241 return (speed); 00242 } 00243 00244 /******************************************************//** 00245 * @brief Returns the device state 00246 * @param[in] motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00247 * @retval State (STEADY or INACTIVE) 00248 **********************************************************/ 00249 motorState_t L6206::L6206_get_device_state(uint8_t motorId) 00250 { 00251 motorState_t state = INACTIVE; 00252 00253 if (motorId > l6206ArrayNbMaxMotorsByConfig[devicePrm.config]) 00254 { 00255 L6206_ErrorHandler(L6206_ERROR_1); 00256 } 00257 else 00258 { 00259 state = devicePrm.motionState[motorId]; 00260 } 00261 return (state); 00262 } 00263 00264 /******************************************************//** 00265 * @brief Returns the FW version of the library 00266 * @retval L6206_FW_VERSION 00267 **********************************************************/ 00268 uint8_t L6206::L6206_GetFwVersion(void) 00269 { 00270 return (L6206_FW_VERSION); 00271 } 00272 00273 /******************************************************//** 00274 * @brief Returns the max speed of the specified motor 00275 * @param[in] motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00276 * @retval maxSpeed in % from 0 to 100 00277 **********************************************************/ 00278 uint16_t L6206::L6206_GetMaxSpeed(uint8_t motorId) 00279 { 00280 uint16_t speed = 0; 00281 if (motorId > l6206ArrayNbMaxMotorsByConfig[devicePrm.config]) 00282 { 00283 L6206_ErrorHandler(L6206_ERROR_1); 00284 } 00285 else 00286 { 00287 speed = devicePrm.speed[motorId]; 00288 } 00289 return (speed); 00290 } 00291 00292 00293 /******************************************************//** 00294 * @brief Get the status of the bridge enabling of the corresponding bridge 00295 * @param[in] bridgeId from 0 for bridge A to 1 for bridge B 00296 * @retval State of the Enable&Flag pin of the corresponding bridge (1 set, 0 for reset) 00297 **********************************************************/ 00298 uint16_t L6206::L6206_GetBridgeStatus(uint8_t bridgeId) 00299 { 00300 uint16_t status = L6206_Board_GetFlagPinState(bridgeId); 00301 00302 return (status); 00303 } 00304 00305 /******************************************************//** 00306 * @brief Immediatly stops the motor and disable the power bridge 00307 * @param[in] motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00308 * @retval None 00309 * @note if two motors uses the same power bridge, the 00310 * power bridge will be disable only if the two motors are 00311 * stopped 00312 **********************************************************/ 00313 void L6206::L6206_HardHiz(uint8_t motorId) 00314 { 00315 if (motorId > l6206ArrayNbMaxMotorsByConfig[devicePrm.config]) 00316 { 00317 L6206_ErrorHandler(L6206_ERROR_1); 00318 } 00319 else 00320 { 00321 /* Get bridge Id of the corresponding motor */ 00322 uint8_t bridgeId = L6206_GetBridgeIdUsedByMotorId(motorId); 00323 00324 if (devicePrm.bridgeEnabled[bridgeId] != FALSE) 00325 { 00326 bool skip = FALSE; 00327 00328 /* Check if another motor is currently running by using the same bridge */ 00329 switch (devicePrm.config) 00330 { 00331 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00332 if ((motorId > 0) && (devicePrm.motionState[1] == STEADY) && (devicePrm.motionState[2] == STEADY)) 00333 { 00334 skip = TRUE; 00335 } 00336 break; 00337 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00338 if ((motorId < 2) && (devicePrm.motionState[0] == STEADY) && (devicePrm.motionState[1] == STEADY)) 00339 { 00340 skip = TRUE; 00341 } 00342 break; 00343 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00344 if (((motorId < 2) && (devicePrm.motionState[0] == STEADY) && (devicePrm.motionState[1] == STEADY))|| 00345 ((motorId > 1) && (devicePrm.motionState[2] == STEADY) && (devicePrm.motionState[3] == STEADY))) 00346 { 00347 skip = TRUE; 00348 } 00349 break; 00350 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00351 if ((motorId > 0) && (devicePrm.motionState[1] == STEADY) && (devicePrm.motionState[2] == STEADY)) 00352 { 00353 skip = TRUE; 00354 } 00355 break; 00356 case PARALLELING_IN1B_IN2B__2_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00357 if ((motorId < 2) && (devicePrm.motionState[0] == STEADY) && (devicePrm.motionState[1] == STEADY)) 00358 { 00359 skip = TRUE; 00360 } 00361 break; 00362 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_UNDIR_MOTOR_BRIDGE_1A__1_UNDIR_MOTOR_BRIDGE_2A: 00363 if ((devicePrm.motionState[0] == STEADY) && (devicePrm.motionState[1] == STEADY)) 00364 { 00365 skip = TRUE; 00366 } 00367 break; 00368 default: 00369 break; 00370 } 00371 00372 if (skip == FALSE) 00373 { 00374 /* Disable the bridge */ 00375 L6206_DisableBridge(bridgeId); 00376 } 00377 } 00378 /* Disable the PWM */ 00379 L6206_HardStop(motorId); 00380 } 00381 } 00382 00383 /******************************************************//** 00384 * @brief Stops the motor without disabling the bridge 00385 * @param[in] motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00386 * @retval none 00387 **********************************************************/ 00388 void L6206::L6206_HardStop(uint8_t motorId) 00389 { 00390 if (motorId > l6206ArrayNbMaxMotorsByConfig[devicePrm.config]) 00391 { 00392 L6206_ErrorHandler(L6206_ERROR_1); 00393 } 00394 else 00395 { 00396 if (devicePrm.motionState[motorId] != INACTIVE) 00397 { 00398 uint8_t bridgeInput; 00399 00400 /* Get bridge input of the corresponding motor */ 00401 bridgeInput = L6206_GetBridgeInputUsedByMotorId(motorId); 00402 00403 /* Disable corresponding PWM */ 00404 L6206_Board_PwmStop(bridgeInput); 00405 00406 /* for bidirectionnal motor, disable second PWM*/ 00407 if (L6206_IsBidirectionnalMotor(motorId)) 00408 { 00409 bridgeInput = L6206_GetSecondBridgeInputUsedByMotorId(motorId); 00410 L6206_Board_PwmStop(bridgeInput); 00411 } 00412 /* Set inactive state */ 00413 devicePrm.motionState[motorId] = INACTIVE; 00414 } 00415 } 00416 } 00417 00418 /******************************************************//** 00419 * @brief Read id 00420 * @retval Id of the l6206 Driver Instance 00421 **********************************************************/ 00422 status_t L6206::L6206_ReadId(uint8_t *id) 00423 { 00424 *id = deviceInstance; 00425 00426 return COMPONENT_OK; 00427 } 00428 00429 /******************************************************//** 00430 * @brief Runs the motor 00431 * @param[in] motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00432 * @param[in] direction FORWARD or BACKWARD 00433 * @retval None 00434 * @note For unidirectionnal motor, direction parameter has 00435 * no effect 00436 **********************************************************/ 00437 void L6206::L6206_Run(uint8_t motorId, motorDir_t direction) 00438 { 00439 if (motorId > l6206ArrayNbMaxMotorsByConfig[devicePrm.config]) 00440 { 00441 L6206_ErrorHandler(L6206_ERROR_1); 00442 } 00443 else 00444 { 00445 if ((devicePrm.motionState[motorId] == INACTIVE) || 00446 (devicePrm.direction[motorId] != direction)) 00447 { 00448 uint8_t bridgeId; 00449 uint8_t bridgeInput; 00450 00451 /* Eventually deactivate motor */ 00452 if (devicePrm.motionState[motorId] != INACTIVE) 00453 { 00454 L6206_HardStop(motorId); 00455 } 00456 00457 /* Store new direction */ 00458 devicePrm.direction[motorId] = direction; 00459 00460 /* Switch to steady state */ 00461 devicePrm.motionState[motorId] = STEADY; 00462 00463 /* Get bridge Id of the corresponding motor */ 00464 bridgeId = L6206_GetBridgeIdUsedByMotorId(motorId); 00465 00466 /* Get bridge input of the corresponding motor */ 00467 bridgeInput = L6206_GetBridgeInputUsedByMotorId(motorId); 00468 00469 /* Enable bridge */ 00470 L6206_EnableBridge(bridgeId); 00471 00472 /* Set PWM */ 00473 if (L6206_IsBidirectionnalMotor(motorId)) 00474 { 00475 /* for bidirectionnal motor */ 00476 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],(100 - devicePrm.speed[motorId])); 00477 bridgeInput = L6206_GetSecondBridgeInputUsedByMotorId(motorId); 00478 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],100); 00479 } 00480 else 00481 { 00482 /* for unidirectionnal motor */ 00483 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],devicePrm.speed[motorId]); 00484 } 00485 } 00486 } 00487 } 00488 /******************************************************//** 00489 * @brief Set dual full bridge parallelling configuration 00490 * @param[in] newConfig bridge configuration to apply from 00491 * dualFullBridgeConfig_t enum 00492 * @retval None 00493 **********************************************************/ 00494 void L6206::L6206_SetDualFullBridgeConfig(uint8_t newConfig) 00495 { 00496 devicePrm.config = (dualFullBridgeConfig_t)newConfig; 00497 00498 /* Start to reset all else if several inits are used successively */ 00499 /* they will fail */ 00500 L6206_Board_PwmDeInit(INPUT_1A); 00501 L6206_Board_PwmDeInit(INPUT_2A); 00502 L6206_Board_PwmDeInit(INPUT_1B); 00503 L6206_Board_PwmDeInit(INPUT_2B); 00504 00505 00506 /* Initialise the bridges inputs PWMs --------------------------------------*/ 00507 switch (devicePrm.config) 00508 { 00509 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00510 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00511 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00512 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00513 L6206_Board_PwmInit(INPUT_1A); 00514 L6206_Board_PwmInit(INPUT_2A); 00515 L6206_Board_PwmInit(INPUT_1B); 00516 L6206_Board_PwmInit(INPUT_2B); 00517 break; 00518 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00519 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00520 L6206_Board_PwmDeInit(INPUT_2A); 00521 L6206_Board_PwmInit(INPUT_1A); 00522 L6206_Board_PwmInit(INPUT_1B); 00523 L6206_Board_PwmInit(INPUT_2B); 00524 break; 00525 case PARALLELING_IN1B_IN2B__1_BIDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00526 case PARALLELING_IN1B_IN2B__2_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00527 L6206_Board_PwmDeInit(INPUT_2B); 00528 L6206_Board_PwmInit(INPUT_1A); 00529 L6206_Board_PwmInit(INPUT_2A); 00530 L6206_Board_PwmInit(INPUT_1B); 00531 break; 00532 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00533 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR: 00534 L6206_Board_PwmDeInit(INPUT_2A); 00535 L6206_Board_PwmDeInit(INPUT_2B); 00536 L6206_Board_PwmInit(INPUT_1A); 00537 L6206_Board_PwmInit(INPUT_1B); 00538 break; 00539 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_UNDIR_MOTOR_BRIDGE_1A__1_UNDIR_MOTOR_BRIDGE_2A: 00540 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_BIDIR_MOTOR: 00541 L6206_Board_PwmDeInit(INPUT_1B); 00542 L6206_Board_PwmDeInit(INPUT_2B); 00543 L6206_Board_PwmInit(INPUT_1A); 00544 L6206_Board_PwmInit(INPUT_2A); 00545 break; 00546 case PARALLELING_ALL_WITH_IN1A___1_UNDIR_MOTOR: 00547 L6206_Board_PwmDeInit(INPUT_2A); 00548 L6206_Board_PwmDeInit(INPUT_1B); 00549 L6206_Board_PwmDeInit(INPUT_2B); 00550 L6206_Board_PwmInit(INPUT_1A); 00551 break; 00552 default: 00553 break; 00554 } 00555 } 00556 /******************************************************//** 00557 * @brief Changes the max speed of the specified device 00558 * @param[in] motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00559 * @param[in] newMaxSpeed in % from 0 to 100 00560 * @retval true if the command is successfully executed, else false 00561 **********************************************************/ 00562 bool L6206::L6206_SetMaxSpeed(uint8_t motorId, uint16_t newMaxSpeed) 00563 { 00564 bool cmdExecuted = FALSE; 00565 00566 if (motorId > l6206ArrayNbMaxMotorsByConfig[devicePrm.config]) 00567 { 00568 L6206_ErrorHandler(L6206_ERROR_1); 00569 } 00570 else 00571 { 00572 devicePrm.speed[motorId] = newMaxSpeed; 00573 if (devicePrm.motionState[motorId] != INACTIVE) 00574 { 00575 uint8_t bridgeInput; 00576 00577 /* Get Bridge input of the corresponding motor */ 00578 bridgeInput = L6206_GetBridgeInputUsedByMotorId(motorId); 00579 00580 /* Set PWM frequency*/ 00581 if (L6206_IsBidirectionnalMotor(motorId)) 00582 { 00583 /* for bidirectionnal motor */ 00584 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],(100 - devicePrm.speed[motorId])); 00585 } 00586 else 00587 { 00588 /* for unidirectionnal motor */ 00589 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],devicePrm.speed[motorId]); 00590 } 00591 } 00592 cmdExecuted = TRUE; 00593 } 00594 return cmdExecuted; 00595 } 00596 00597 /******************************************************//** 00598 * @brief Changes the PWM frequency of the bridge input 00599 * @param[in] bridgeId 0 for bridge A, 1 for bridge B 00600 * @param[in] newFreq in Hz 00601 * @retval None 00602 **********************************************************/ 00603 void L6206::L6206_SetBridgeInputPwmFreq(uint8_t bridgeId, uint32_t newFreq) 00604 { 00605 uint8_t loop; 00606 00607 if (newFreq > L6206_MAX_PWM_FREQ) 00608 { 00609 newFreq = L6206_MAX_PWM_FREQ; 00610 } 00611 for (loop = 0; loop < 2;loop ++) 00612 { 00613 uint8_t motorId; 00614 uint8_t bridgeInput = (bridgeId << 1) + loop; 00615 devicePrm.pwmFreq[bridgeInput] = newFreq; 00616 00617 /* Get motor Id using this bridge */ 00618 motorId = L6206_GetMotorIdUsingbridgeInput(bridgeInput); 00619 00620 /* Immediatly update frequency if motor is running */ 00621 if (devicePrm.motionState[motorId] != INACTIVE) 00622 { 00623 /* Test if motor is bidir */ 00624 if (L6206_IsBidirectionnalMotor(motorId)) 00625 { 00626 if (bridgeInput != L6206_GetSecondBridgeInputUsedByMotorId(motorId)) 00627 { 00628 /* Set PWM frequency for bidirectionnal motor of the first bridge*/ 00629 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],(100 - devicePrm.speed[motorId])); 00630 } 00631 else 00632 { 00633 /* Set PWM frequency for bidirectionnal motor of the second bridge */ 00634 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],100); 00635 } 00636 } 00637 else 00638 { 00639 /* Set PWM frequency for unidirectionnal motor */ 00640 L6206_Board_PwmSetFreq(bridgeInput, devicePrm.pwmFreq[bridgeInput],devicePrm.speed[motorId]); 00641 } 00642 } 00643 } 00644 } 00645 /******************************************************//** 00646 * @brief Sets the number of devices to be used 00647 * @param[in] nbDevices (from 1 to MAX_NUMBER_OF_DEVICES) 00648 * @retval TRUE if successfull, FALSE if failure, attempt to set a number of 00649 * devices greater than MAX_NUMBER_OF_DEVICES 00650 **********************************************************/ 00651 bool L6206::L6206_SetNbDevices(uint8_t nbDevices) 00652 { 00653 if (nbDevices <= MAX_NUMBER_OF_DEVICES) 00654 { 00655 return TRUE; 00656 } 00657 else 00658 { 00659 return FALSE; 00660 } 00661 } 00662 00663 00664 /******************************************************//** 00665 * @brief Error handler which calls the user callback (if defined) 00666 * @param[in] error Number of the error 00667 * @retval None 00668 **********************************************************/ 00669 void L6206::L6206_ErrorHandler(uint16_t error) 00670 { 00671 if (errorHandlerCallback != 0) 00672 { 00673 (void) errorHandlerCallback(error); 00674 } 00675 else 00676 { 00677 while(1) 00678 { 00679 /* Infinite loop */ 00680 } 00681 } 00682 } 00683 00684 /******************************************************//** 00685 * @brief Handlers of the flag interrupt which calls the user callback (if defined) 00686 * @retval None 00687 **********************************************************/ 00688 void L6206::L6206_FlagInterruptHandler(void) 00689 { 00690 bool status; 00691 00692 status = L6206_GetBridgeStatus(BRIDGE_A); 00693 if (status != devicePrm.bridgeEnabled[BRIDGE_A]) 00694 { 00695 devicePrm.bridgeEnabled[BRIDGE_A] = status; 00696 } 00697 00698 status = L6206_GetBridgeStatus(BRIDGE_B); 00699 if (status != devicePrm.bridgeEnabled[BRIDGE_B]) 00700 { 00701 devicePrm.bridgeEnabled[BRIDGE_B] = status; 00702 } 00703 00704 if (flagInterruptCallback != 0) 00705 { 00706 flagInterruptCallback(); 00707 } 00708 } 00709 00710 /******************************************************//** 00711 * @brief Get the bridges Id used by a given motor 00712 * @param motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00713 * @retval bridgeId 0 for bridge A , 1 for bridge B 00714 **********************************************************/ 00715 uint8_t L6206::L6206_GetBridgeIdUsedByMotorId(uint8_t motorId) 00716 { 00717 uint8_t bridgeId; 00718 00719 switch (devicePrm.config) 00720 { 00721 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00722 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00723 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00724 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00725 case PARALLELING_IN1B_IN2B__1_BIDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00726 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00727 if (motorId == 0) 00728 { 00729 bridgeId = 0; 00730 } 00731 else 00732 { 00733 bridgeId = 1; 00734 } 00735 break; 00736 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00737 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00738 case PARALLELING_IN1B_IN2B__2_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00739 if (motorId < 2) 00740 { 00741 bridgeId = 0; 00742 } 00743 else 00744 { 00745 bridgeId = 1; 00746 } 00747 break; 00748 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR: 00749 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_UNDIR_MOTOR_BRIDGE_1A__1_UNDIR_MOTOR_BRIDGE_2A: 00750 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_BIDIR_MOTOR: 00751 case PARALLELING_ALL_WITH_IN1A___1_UNDIR_MOTOR: 00752 default: 00753 bridgeId = 0; 00754 break; 00755 } 00756 return (bridgeId); 00757 } 00758 00759 /******************************************************//** 00760 * @brief Get the motor Id which is using the specified bridge input 00761 * @param bridgeInput 0 for bridgeInput 1A, 1 for 2A, 2 for 1B, 3 for 3B 00762 * @retval bridgeId 0 for bridge A , 1 for bridge B 00763 **********************************************************/ 00764 uint8_t L6206::L6206_GetMotorIdUsingbridgeInput(uint8_t bridgeInput) 00765 { 00766 uint8_t motorId; 00767 00768 switch (devicePrm.config) 00769 { 00770 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00771 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00772 case PARALLELING_IN1B_IN2B__1_BIDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00773 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00774 if (bridgeInput >= INPUT_1B) 00775 { 00776 motorId = 1; 00777 } 00778 else 00779 { 00780 motorId = 0; 00781 } 00782 break; 00783 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00784 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00785 if (bridgeInput == INPUT_2B) 00786 { 00787 motorId = 2; 00788 } 00789 else 00790 { 00791 if (bridgeInput == INPUT_1B) 00792 { 00793 motorId = 1; 00794 } 00795 else 00796 { 00797 motorId = 0; 00798 } 00799 } 00800 break; 00801 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00802 case PARALLELING_IN1B_IN2B__2_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 00803 if (bridgeInput >= INPUT_1B) 00804 { 00805 motorId = 2; 00806 } 00807 else 00808 { 00809 if (bridgeInput == INPUT_2A) 00810 { 00811 motorId = 1; 00812 } 00813 else 00814 { 00815 motorId = 0; 00816 } 00817 } 00818 break; 00819 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00820 if (bridgeInput == INPUT_2B) 00821 { 00822 motorId = 3; 00823 } 00824 else 00825 { 00826 if (bridgeInput == INPUT_1B) 00827 { 00828 motorId = 2; 00829 } 00830 else 00831 { 00832 if (bridgeInput == INPUT_2A) 00833 { 00834 motorId = 1; 00835 } 00836 else 00837 { 00838 motorId = 0; 00839 } 00840 } 00841 } 00842 break; 00843 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_UNDIR_MOTOR_BRIDGE_1A__1_UNDIR_MOTOR_BRIDGE_2A: 00844 if ((bridgeInput == INPUT_2A) || (bridgeInput == INPUT_2B)) 00845 { 00846 motorId = 1; 00847 } 00848 else 00849 { 00850 motorId = 0; 00851 } 00852 break; 00853 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR: 00854 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_BIDIR_MOTOR: 00855 case PARALLELING_ALL_WITH_IN1A___1_UNDIR_MOTOR: 00856 default: 00857 motorId = 0; 00858 break; 00859 } 00860 00861 return (motorId); 00862 } 00863 /******************************************************//** 00864 * @brief Get the PWM input used by a given motor 00865 * @param motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 00866 * @retval PWM input 0 for 1A, 1 for 2A, 2 for 1B, 3 for 3B 00867 **********************************************************/ 00868 uint8_t L6206::L6206_GetBridgeInputUsedByMotorId(uint8_t motorId) 00869 { 00870 uint8_t bridgeInput; 00871 00872 switch (devicePrm.config) 00873 { 00874 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00875 if (motorId == 0) 00876 { 00877 if (devicePrm.direction[0] == FORWARD) 00878 { 00879 bridgeInput = INPUT_1A; 00880 } 00881 else 00882 { 00883 bridgeInput = INPUT_2A; 00884 } 00885 } 00886 else 00887 { 00888 if (devicePrm.direction[1] == FORWARD) 00889 { 00890 bridgeInput = INPUT_1B; 00891 } 00892 else 00893 { 00894 bridgeInput = INPUT_2B; 00895 } 00896 } 00897 break; 00898 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00899 if (motorId == 0) 00900 { 00901 if (devicePrm.direction[0] == FORWARD) 00902 { 00903 bridgeInput = INPUT_1A; 00904 } 00905 else 00906 { 00907 bridgeInput = INPUT_2A; 00908 } 00909 } 00910 else 00911 { 00912 if (motorId == 1) 00913 { 00914 bridgeInput = INPUT_1B; 00915 } 00916 else 00917 { 00918 bridgeInput = INPUT_2B; 00919 } 00920 } 00921 break; 00922 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00923 if (motorId == 0) 00924 { 00925 bridgeInput = INPUT_1A; 00926 } 00927 else 00928 { 00929 if (motorId == 1) 00930 { 00931 bridgeInput = INPUT_2A; 00932 } 00933 else 00934 { 00935 if (devicePrm.direction[2] == FORWARD) 00936 { 00937 bridgeInput = INPUT_1B; 00938 } 00939 else 00940 { 00941 bridgeInput = INPUT_2B; 00942 } 00943 } 00944 } 00945 break; 00946 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00947 if (motorId == 0) 00948 { 00949 bridgeInput = INPUT_1A; 00950 } 00951 else 00952 { 00953 if (motorId == 1) 00954 { 00955 bridgeInput = INPUT_2A; 00956 } 00957 else 00958 { 00959 if (motorId == 2) 00960 { 00961 bridgeInput = INPUT_1B; 00962 } 00963 else 00964 { 00965 bridgeInput = INPUT_2B; 00966 } 00967 } 00968 } 00969 break; 00970 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 00971 if (motorId == 0) 00972 { 00973 bridgeInput = INPUT_1A; 00974 } 00975 else 00976 { 00977 if (devicePrm.direction[1] == FORWARD) 00978 { 00979 bridgeInput = INPUT_1B; 00980 } 00981 else 00982 { 00983 bridgeInput = INPUT_2B; 00984 } 00985 } 00986 break; 00987 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 00988 if (motorId == 0) 00989 { 00990 bridgeInput = INPUT_1A; 00991 } 00992 else 00993 { 00994 if (motorId == 1) 00995 { 00996 bridgeInput = INPUT_1B; 00997 } 00998 else 00999 { 01000 bridgeInput = INPUT_2B; 01001 } 01002 } 01003 break; 01004 case PARALLELING_IN1B_IN2B__1_BIDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 01005 if (motorId == 0) 01006 { 01007 if (devicePrm.direction[0] == FORWARD) 01008 { 01009 bridgeInput = INPUT_1A; 01010 } 01011 else 01012 { 01013 bridgeInput = INPUT_2A; 01014 } 01015 } 01016 else 01017 { 01018 bridgeInput = INPUT_1B; 01019 } 01020 break; 01021 case PARALLELING_IN1B_IN2B__2_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 01022 if (motorId == 0) 01023 { 01024 bridgeInput = INPUT_1A; 01025 } 01026 else 01027 { 01028 if (motorId == 1) 01029 { 01030 bridgeInput = INPUT_2A; 01031 } 01032 else 01033 { 01034 bridgeInput = INPUT_1B; 01035 } 01036 } 01037 break; 01038 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_UNDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 01039 if (motorId == 0) 01040 { 01041 bridgeInput = INPUT_1A; 01042 } 01043 else 01044 { 01045 bridgeInput = INPUT_1B; 01046 } 01047 break; 01048 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR: 01049 if (devicePrm.direction[0] == FORWARD) 01050 { 01051 bridgeInput = INPUT_1A; 01052 } 01053 else 01054 { 01055 bridgeInput = INPUT_1B; 01056 } 01057 break; 01058 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_UNDIR_MOTOR_BRIDGE_1A__1_UNDIR_MOTOR_BRIDGE_2A: 01059 if (motorId == 0) 01060 { 01061 bridgeInput = INPUT_1A; 01062 } 01063 else 01064 { 01065 bridgeInput = INPUT_2A; 01066 } 01067 break; 01068 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_BIDIR_MOTOR: 01069 if (devicePrm.direction[0] == FORWARD) 01070 { 01071 bridgeInput = INPUT_1A; 01072 } 01073 else 01074 { 01075 bridgeInput = INPUT_2A; 01076 } 01077 break; 01078 case PARALLELING_ALL_WITH_IN1A___1_UNDIR_MOTOR: 01079 default: 01080 bridgeInput = INPUT_1A; 01081 break; 01082 } 01083 return (bridgeInput); 01084 } 01085 01086 /******************************************************//** 01087 * @brief Get the second PWM input used by a given bidirectionnal motor 01088 * @param motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 01089 * @retval PWM input 0 for 1A, 1 for 2A, 2 for 1B, 3 for 3B 01090 **********************************************************/ 01091 uint8_t L6206::L6206_GetSecondBridgeInputUsedByMotorId(uint8_t motorId) 01092 { 01093 uint8_t bridgeInput = 0xFF; 01094 01095 switch (devicePrm.config) 01096 { 01097 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 01098 if (motorId == 0) 01099 { 01100 if (devicePrm.direction[0] == FORWARD) 01101 { 01102 bridgeInput = INPUT_2A; 01103 } 01104 else 01105 { 01106 bridgeInput = INPUT_1A; 01107 } 01108 } 01109 else 01110 { 01111 if (devicePrm.direction[1] == FORWARD) 01112 { 01113 bridgeInput = INPUT_2B; 01114 } 01115 else 01116 { 01117 bridgeInput = INPUT_1B; 01118 } 01119 } 01120 break; 01121 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 01122 if (motorId == 0) 01123 { 01124 if (devicePrm.direction[0] == FORWARD) 01125 { 01126 bridgeInput = INPUT_2A; 01127 } 01128 else 01129 { 01130 bridgeInput = INPUT_1A; 01131 } 01132 } 01133 break; 01134 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 01135 if (motorId == 2) 01136 { 01137 if (devicePrm.direction[2] == FORWARD) 01138 { 01139 bridgeInput = INPUT_2B; 01140 } 01141 else 01142 { 01143 bridgeInput = INPUT_1B; 01144 } 01145 } 01146 break; 01147 01148 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 01149 if (motorId == 1) 01150 { 01151 if (devicePrm.direction[1] == FORWARD) 01152 { 01153 bridgeInput = INPUT_2B; 01154 } 01155 else 01156 { 01157 bridgeInput = INPUT_1B; 01158 } 01159 } 01160 break; 01161 01162 case PARALLELING_IN1B_IN2B__1_BIDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 01163 if (motorId == 0) 01164 { 01165 if (devicePrm.direction[0] == FORWARD) 01166 { 01167 bridgeInput = INPUT_2A; 01168 } 01169 else 01170 { 01171 bridgeInput = INPUT_1A; 01172 } 01173 } 01174 break; 01175 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR: 01176 if (devicePrm.direction[0] == FORWARD) 01177 { 01178 bridgeInput = INPUT_1B; 01179 } 01180 else 01181 { 01182 bridgeInput = INPUT_1A; 01183 } 01184 break; 01185 01186 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_BIDIR_MOTOR: 01187 if (devicePrm.direction[0] == FORWARD) 01188 { 01189 bridgeInput = INPUT_2A; 01190 } 01191 else 01192 { 01193 bridgeInput = INPUT_1A; 01194 } 01195 break; 01196 default: 01197 bridgeInput = 0XFF; 01198 break; 01199 } 01200 if (bridgeInput == 0XFF) 01201 { 01202 L6206_ErrorHandler(L6206_ERROR_2); 01203 } 01204 01205 return (bridgeInput); 01206 } 01207 01208 /******************************************************//** 01209 * @brief Test if motor is bidirectionnal 01210 * @param motorId from 0 to MAX_NUMBER_OF_BRUSH_DC_MOTORS 01211 * @retval True if motor is bidirectionnal, else false 01212 **********************************************************/ 01213 bool L6206::L6206_IsBidirectionnalMotor(uint8_t motorId) 01214 { 01215 bool isBiDir = FALSE; 01216 01217 switch (devicePrm.config) 01218 { 01219 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 01220 case PARALLELING_IN1A_IN2A__IN1B_IN2B__1_BIDIR_MOTOR: 01221 case PARALLELING_IN1A_IN1B__IN2A_IN2B__1_BIDIR_MOTOR: 01222 isBiDir = TRUE; 01223 break; 01224 01225 case PARALLELING_NONE___1_BIDIR_MOTOR_BRIDGE_A__2_UNDIR_MOTOR_BRIDGE_B: 01226 case PARALLELING_IN1B_IN2B__1_BIDIR_MOTOR_BRIDGE_A__1_UNDIR_MOTOR_BRIDGE_B: 01227 if (motorId == 0) 01228 { 01229 isBiDir = TRUE; 01230 } 01231 break; 01232 case PARALLELING_NONE___2_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 01233 if (motorId == 2) 01234 { 01235 isBiDir = TRUE; 01236 } 01237 break; 01238 case PARALLELING_IN1A_IN2A__1_UNDIR_MOTOR_BRIDGE_A__1_BIDIR_MOTOR_BRIDGE_B: 01239 if (motorId == 1) 01240 { 01241 isBiDir = TRUE; 01242 } 01243 break; 01244 01245 default: 01246 break; 01247 } 01248 01249 return (isBiDir); 01250 } 01251 01252 01253 /******************************************************//** 01254 * @brief Sets the parameters of the device to predefined values 01255 * from l6206_target_config.h 01256 * @retval None 01257 **********************************************************/ 01258 void L6206::L6206_SetDeviceParamsToPredefinedValues(void) 01259 { 01260 uint32_t i; 01261 01262 memset(&devicePrm, 0, sizeof(devicePrm)); 01263 01264 devicePrm.config = L6206_CONF_PARAM_PARALLE_BRIDGES; 01265 01266 devicePrm.pwmFreq[INPUT_1A] = L6206_CONF_PARAM_FREQ_PWM1A; 01267 devicePrm.pwmFreq[INPUT_2A] = L6206_CONF_PARAM_FREQ_PWM2A; 01268 devicePrm.pwmFreq[INPUT_1B] = L6206_CONF_PARAM_FREQ_PWM1B; 01269 devicePrm.pwmFreq[INPUT_2B] = L6206_CONF_PARAM_FREQ_PWM2B; 01270 01271 for (i = 0; i < MAX_NUMBER_OF_BRUSH_DC_MOTORS; i++) 01272 { 01273 devicePrm.speed[i] = 100; 01274 devicePrm.direction[i] = FORWARD; 01275 devicePrm.motionState[i] = INACTIVE; 01276 } 01277 for (i = 0; i < L6206_NB_MAX_BRIDGES; i++) 01278 { 01279 devicePrm.bridgeEnabled[i] = FALSE; 01280 } 01281 } 01282 01283 01284 /******************************************************//** 01285 * @brief Set the parameters of the device to values of initDevicePrm structure 01286 * Set GPIO according to these values 01287 * @param initDevicePrm structure containing values to initialize the device 01288 * parameters 01289 * @retval None 01290 **********************************************************/ 01291 void L6206::L6206_SetDeviceParamsToGivenValues(L6206_init_t* initDevicePrm) 01292 { 01293 memcpy(&devicePrm, initDevicePrm, sizeof(devicePrm)); 01294 } 01295 01296 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jul 15 2022 19:41:39 by
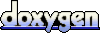