
Basic demo showing how to use the touch screen present on the DISCO_F469NI board.
Dependencies: BSP_DISCO_F469NI LCD_DISCO_F469NI TS_DISCO_F469NI mbed
main.cpp
00001 #include "mbed.h" 00002 #include "TS_DISCO_F469NI.h" 00003 #include "LCD_DISCO_F469NI.h" 00004 00005 LCD_DISCO_F469NI lcd; 00006 TS_DISCO_F469NI ts; 00007 00008 int main() 00009 { 00010 TS_StateTypeDef TS_State; 00011 uint16_t x, y; 00012 uint8_t text[30]; 00013 uint8_t status; 00014 uint8_t idx; 00015 uint8_t cleared = 0; 00016 uint8_t prev_nb_touches = 0; 00017 00018 BSP_LCD_SetFont(&Font24); 00019 00020 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN DEMO", CENTER_MODE); 00021 wait(1); 00022 00023 status = ts.Init(lcd.GetXSize(), lcd.GetYSize()); 00024 if (status != TS_OK) 00025 { 00026 lcd.Clear(LCD_COLOR_RED); 00027 lcd.SetBackColor(LCD_COLOR_RED); 00028 lcd.SetTextColor(LCD_COLOR_WHITE); 00029 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT FAIL", CENTER_MODE); 00030 } 00031 else 00032 { 00033 lcd.Clear(LCD_COLOR_GREEN); 00034 lcd.SetBackColor(LCD_COLOR_GREEN); 00035 lcd.SetTextColor(LCD_COLOR_WHITE); 00036 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT OK", CENTER_MODE); 00037 } 00038 00039 wait(1); 00040 lcd.SetBackColor(LCD_COLOR_BLUE); 00041 lcd.SetTextColor(LCD_COLOR_WHITE); 00042 00043 while(1) 00044 { 00045 00046 ts.GetState(&TS_State); 00047 if (TS_State.touchDetected) 00048 { 00049 // Clear lines corresponding to old touches coordinates 00050 if (TS_State.touchDetected < prev_nb_touches) 00051 { 00052 for (idx = (TS_State.touchDetected + 1); idx <= 5; idx++) 00053 { 00054 lcd.ClearStringLine(idx); 00055 } 00056 } 00057 prev_nb_touches = TS_State.touchDetected; 00058 00059 cleared = 0; 00060 00061 sprintf((char*)text, "Touches: %d", TS_State.touchDetected); 00062 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); 00063 00064 for (idx = 0; idx < TS_State.touchDetected; idx++) 00065 { 00066 x = TS_State.touchX[idx]; 00067 y = TS_State.touchY[idx]; 00068 sprintf((char*)text, "Touch %d: x=%d y=%d ", idx+1, x, y); 00069 lcd.DisplayStringAt(0, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00070 lcd.FillCircle(TS_State.touchX[idx], TS_State.touchY[idx], 20); 00071 } 00072 } 00073 else 00074 { 00075 if (!cleared) 00076 { 00077 lcd.Clear(LCD_COLOR_BLUE); 00078 sprintf((char*)text, "Touches: 0"); 00079 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); 00080 cleared = 1; 00081 } 00082 } 00083 } 00084 }
Generated on Thu Jul 14 2022 19:19:06 by
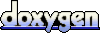