Class for BSP AUDIO for DISCO_F746NG
Dependents: DISCO-F746NG_Monttory_Base DISCO-F746NG_Monttory_Base DISCO-F746NG_AUDIO_demo DISCO-F746NG_AUDIO_demo_copy
AUDIO_DISCO_F746NG.cpp
00001 /* Copyright (c) 2010-2016 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "AUDIO_DISCO_F746NG.h" 00020 00021 // Constructor 00022 AUDIO_DISCO_F746NG::AUDIO_DISCO_F746NG() 00023 { 00024 BSP_AUDIO_IN_OUT_Init(INPUT_DEVICE_DIGITAL_MICROPHONE_2, OUTPUT_DEVICE_HEADPHONE, DEFAULT_AUDIO_IN_FREQ, DEFAULT_AUDIO_IN_BIT_RESOLUTION, DEFAULT_AUDIO_IN_CHANNEL_NBR); 00025 } 00026 00027 // Destructor 00028 AUDIO_DISCO_F746NG::~AUDIO_DISCO_F746NG() 00029 { 00030 00031 } 00032 00033 //================================================================================================================= 00034 // Public methods 00035 //================================================================================================================= 00036 00037 uint8_t AUDIO_DISCO_F746NG::OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) 00038 { 00039 return BSP_AUDIO_OUT_Init(OutputDevice, Volume, AudioFreq); 00040 } 00041 00042 uint8_t AUDIO_DISCO_F746NG::OUT_Play(uint16_t* pBuffer, uint32_t Size) 00043 { 00044 return BSP_AUDIO_OUT_Play(pBuffer, Size); 00045 } 00046 00047 void AUDIO_DISCO_F746NG::OUT_ChangeBuffer(uint16_t *pData, uint16_t Size) 00048 { 00049 BSP_AUDIO_OUT_ChangeBuffer(pData, Size); 00050 } 00051 00052 uint8_t AUDIO_DISCO_F746NG::OUT_Pause(void) 00053 { 00054 return BSP_AUDIO_OUT_Pause(); 00055 } 00056 00057 uint8_t AUDIO_DISCO_F746NG::OUT_Resume(void) 00058 { 00059 return BSP_AUDIO_OUT_Resume(); 00060 } 00061 00062 uint8_t AUDIO_DISCO_F746NG::OUT_Stop(uint32_t Option) 00063 { 00064 return BSP_AUDIO_OUT_Stop(Option); 00065 } 00066 00067 uint8_t AUDIO_DISCO_F746NG::OUT_SetVolume(uint8_t Volume) 00068 { 00069 return BSP_AUDIO_OUT_SetVolume(Volume); 00070 } 00071 00072 uint8_t AUDIO_DISCO_F746NG::OUT_SetMute(uint32_t Cmd) 00073 { 00074 return BSP_AUDIO_OUT_SetMute(Cmd); 00075 } 00076 00077 uint8_t AUDIO_DISCO_F746NG::OUT_SetOutputMode(uint8_t Output) 00078 { 00079 return BSP_AUDIO_OUT_SetOutputMode(Output); 00080 } 00081 00082 void AUDIO_DISCO_F746NG::OUT_SetFrequency(uint32_t AudioFreq) 00083 { 00084 BSP_AUDIO_OUT_SetFrequency(AudioFreq); 00085 } 00086 00087 void AUDIO_DISCO_F746NG::OUT_SetAudioFrameSlot(uint32_t AudioFrameSlot) 00088 { 00089 BSP_AUDIO_OUT_SetAudioFrameSlot(AudioFrameSlot); 00090 } 00091 00092 void AUDIO_DISCO_F746NG::OUT_DeInit(void) 00093 { 00094 BSP_AUDIO_OUT_DeInit(); 00095 } 00096 00097 uint8_t AUDIO_DISCO_F746NG::IN_Init(uint16_t InputDevice, uint8_t Volume, uint32_t AudioFreq) 00098 { 00099 return BSP_AUDIO_IN_Init(InputDevice, Volume, AudioFreq); 00100 } 00101 00102 uint8_t AUDIO_DISCO_F746NG::IN_OUT_Init(uint16_t InputDevice, uint16_t OutputDevice, uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr) 00103 { 00104 return BSP_AUDIO_IN_OUT_Init(InputDevice, OutputDevice, AudioFreq, BitRes, ChnlNbr); 00105 } 00106 00107 uint8_t AUDIO_DISCO_F746NG::IN_Record(uint16_t* pbuf, uint32_t size) 00108 { 00109 return BSP_AUDIO_IN_Record(pbuf, size); 00110 } 00111 00112 uint8_t AUDIO_DISCO_F746NG::IN_Stop(uint32_t Option) 00113 { 00114 return BSP_AUDIO_IN_Stop(Option); 00115 } 00116 00117 uint8_t AUDIO_DISCO_F746NG::IN_Pause(void) 00118 { 00119 return BSP_AUDIO_IN_Pause(); 00120 } 00121 00122 uint8_t AUDIO_DISCO_F746NG::IN_Resume(void) 00123 { 00124 return BSP_AUDIO_IN_Resume(); 00125 } 00126 00127 uint8_t AUDIO_DISCO_F746NG::IN_SetVolume(uint8_t Volume) 00128 { 00129 return BSP_AUDIO_IN_SetVolume(Volume); 00130 } 00131 00132 void AUDIO_DISCO_F746NG::IN_DeInit(void) 00133 { 00134 BSP_AUDIO_IN_DeInit(); 00135 }
Generated on Thu Jul 14 2022 09:28:47 by
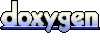