
Sample program showing how to connect GR-PEACH into Watson IoT through mbed Connector and Watson's Connector Bridge
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
Utils.cpp
00001 /** 00002 * @file Utils.cpp 00003 * @brief mbed CoAP Endpoint misc utils collection 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 // mbed Endpoint includes 00024 #include "mbed-connector-interface/ConnectorEndpoint.h" 00025 #include "mbed-connector-interface/OptionsBuilder.h" 00026 #include "mbed-connector-interface/mbedEndpointNetwork.h" 00027 #include "mbed-connector-interface/DeviceManager.h" 00028 #include "mbed-connector-interface/ObjectInstanceManager.h" 00029 00030 // External references (defined in main.cpp) 00031 Connector::Options *configure_endpoint(Connector::OptionsBuilder &builder); 00032 extern Logger logger; 00033 00034 // Our Endpoint 00035 Connector::Endpoint *__endpoint = NULL; 00036 00037 // Our Endpoint configured Options 00038 Connector::OptionsBuilder config; 00039 Connector::Options *options = NULL; 00040 00041 // initialize the Connector::Endpoint instance 00042 void *utils_init_endpoint(bool canActAsRouterNode) { 00043 // alloc Endpoint 00044 logger.logging("Endpoint: allocating endpoint instance..."); 00045 Connector::Endpoint *ep = new Connector::Endpoint(&logger,options); 00046 if (ep != NULL) { 00047 // link to config object 00048 config.setEndpoint((void *)ep); 00049 00050 // not sure if we need this anymore... 00051 ep->asRouterNode(canActAsRouterNode); 00052 00053 // Add ObjectInstanceManager 00054 ObjectInstanceManager *oim = new ObjectInstanceManager(&logger,(void *)ep); 00055 ep->setObjectInstanceManager(oim); 00056 } 00057 return (void *)ep; 00058 } 00059 00060 // further simplifies the endpoint main() configuration by removing the final initialization details of the endpoint... 00061 void utils_configure_endpoint(void *p) 00062 { 00063 // our Endpoint 00064 Connector::Endpoint *ep = (Connector::Endpoint *)p; 00065 00066 // default configuration - see mbedConnectorInterface.h for definitions... 00067 logger.logging("Endpoint: setting defaults..."); 00068 config.setEndpointNodename(NODE_NAME); 00069 config.setEndpointType(DEFAULT_ENDPOINT_TYPE); 00070 config.setLifetime(REG_LIFETIME_SEC); 00071 00072 // WiFi defaults 00073 config.setWiFiSSID((char *)WIFI_DEFAULT_SSID); // default: changeme 00074 config.setWiFiAuthType(WIFI_NONE); // default: none 00075 config.setWiFiAuthKey((char *)WIFI_DEFAULT_AUTH_KEY); // default: changeme 00076 00077 // Default CoAP Connection Type 00078 config.setCoAPConnectionType(COAP_UDP); // default CoAP Connection Type 00079 00080 // Set the default IP Address Type 00081 #if MBED_CONF_APP_NETWORK_INTERFACE == MESH_LOWPAN_ND || MBED_CONF_APP_NETWORK_INTERFACE == MESH_THREAD 00082 config.setIPAddressType(IP_ADDRESS_TYPE_IPV6); // IPv6 is the default for mesh 00083 #else 00084 config.setIPAddressType(IP_ADDRESS_TYPE_IPV4); // IPv4 is the default for all but mesh 00085 #endif 00086 00087 // Establish default CoAP observation behavior 00088 config.setImmedateObservationEnabled(true); 00089 00090 // Establish default CoAP GET-based observation control behavior 00091 config.setEnableGETObservationControl(false); 00092 00093 // Device Manager installation 00094 DeviceManager *device_manager = (DeviceManager *)ep->getDeviceManager(); 00095 if (device_manager != NULL) { 00096 logger.logging("Endpoint: installing and setting up device manager and its resources..."); 00097 device_manager->install((void *)ep,(void *)&config); 00098 } 00099 else { 00100 logger.logging("Endpoint: no device manager installed..."); 00101 } 00102 00103 // main.cpp can override or change any of the above defaults... 00104 logger.logging("Endpoint: gathering configuration overrides..."); 00105 options = configure_endpoint(config); 00106 00107 // set our options 00108 ep->setOptions(options); 00109 00110 // DONE 00111 logger.logging("Endpoint: endpoint configuration completed."); 00112 } 00113 00114 // build out the endpoint and its resources 00115 void utils_build_endpoint(void *p) 00116 { 00117 if (p != NULL) { 00118 // Build the Endpoint 00119 logger.logging("Endpoint: building endpoint and its resources..."); 00120 Connector::Endpoint *ep = (Connector::Endpoint *)p; 00121 ep->buildEndpoint(); 00122 } 00123 } 00124 00125 // parse out the CoAP port number from the connection URL 00126 uint16_t extract_port_from_url(char *url,uint16_t default_port) 00127 { 00128 uint16_t port = default_port; 00129 00130 if (url != NULL && strlen(url) > 0) { 00131 char buffer[MAX_CONN_URL_LENGTH+1]; 00132 char uri[MAX_CONN_URL_LENGTH+1]; 00133 char path[MAX_CONN_URL_LENGTH+1]; 00134 00135 // initialize the buffer 00136 memset(buffer,0,MAX_CONN_URL_LENGTH+1); 00137 memset(uri,0,MAX_CONN_URL_LENGTH+1); 00138 memset(path,0,MAX_CONN_URL_LENGTH+1); 00139 int length = strlen(url); 00140 00141 // truncate if needed 00142 if (length >MAX_CONN_URL_LENGTH) length = MAX_CONN_URL_LENGTH; 00143 00144 // make a copy... 00145 memcpy(buffer,url,length); 00146 00147 // remove the forward slashes and colons 00148 for(int i=0;i<length;++i) { 00149 if (buffer[i] == ':') buffer[i] = ' '; 00150 if (buffer[i] == '/') buffer[i] = ' '; 00151 if (buffer[i] == '[') buffer[i] = ' '; 00152 if (buffer[i] == ']') buffer[i] = ' '; 00153 } 00154 00155 // parse 00156 sscanf(buffer,"%s%s%d",uri,path,(int *)&port); 00157 00158 // IPv6 kludge until we parse it properly... 00159 if (strcmp(uri,"coaps") == 0 && strcmp(path,"2607") == 0) { 00160 // IPv6 in use - so nail to Connector's IPv6 address 00161 strcpy(uri,"coap"); 00162 strcpy(path,"2607:f0d0:2601:52::20"); 00163 port = default_port; 00164 00165 // DEBUG 00166 logger.logging("Endpoint: Connector IPV6 uri: %s path: %s port: %d",uri,path,port); 00167 } 00168 } 00169 00170 // DEBUG 00171 logger.logging("Endpoint: Connector URL: %s CoAP port: %u",url,port); 00172 00173 // return the port 00174 return port; 00175 }
Generated on Thu Jul 14 2022 21:25:54 by
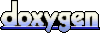