
Sample program showing how to connect GR-PEACH into Watson IoT through mbed Connector and Watson's Connector Bridge
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
StaticResource.h
00001 /** 00002 * @file StaticResource.h 00003 * @brief mbed CoAP Endpoint Static Resource class 00004 * @author Doug Anson/Chris Paola 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __STATIC_RESOURCE_H__ 00024 #define __STATIC_RESOURCE_H__ 00025 00026 // Base Class 00027 #include "mbed-connector-interface/Resource.h" 00028 00029 // DataWrapper support 00030 #include "mbed-connector-interface/DataWrapper.h" 00031 00032 /** StaticResource is a static (GET only) resource with a value type pinned as a string type 00033 */ 00034 class StaticResource : public Resource<string> 00035 { 00036 public: 00037 /** 00038 Default constructor 00039 @param logger input logger instance for this resource 00040 @param obj_name input the Object 00041 @param res_name input the Resource URI/Name 00042 @param value input the Resource value (a string) 00043 */ 00044 StaticResource(const Logger *logger,const char *obj_name,const char *res_name,const char *value); 00045 00046 /** 00047 string value constructor 00048 @param logger input logger instance for this resource 00049 @param obj_name input the Object 00050 @param name input the Resource URI/Name 00051 @param value input the Resource value (a string) 00052 */ 00053 StaticResource(const Logger *logger,const char *obj_name,const char *res_name,const string value); 00054 00055 /** 00056 constructor with buffer lengths 00057 @param logger input logger instance for this resource 00058 @param obj_name input the Object 00059 @param name input the Resource URI/Name 00060 #param name_length input the length of the Resource URI/Name 00061 @param value input the Resource value (or NULL) 00062 */ 00063 StaticResource(const Logger *logger,const string obj_name,const string res_name,const string value); 00064 00065 /** 00066 Copy constructor 00067 @param resource input the StaticResource that is to be deep copied 00068 */ 00069 StaticResource(const StaticResource &resource); 00070 00071 /** 00072 Destructor 00073 */ 00074 virtual ~StaticResource(); 00075 00076 /** 00077 Bind resource to endpoint 00078 @param ep input endpoint instance pointer 00079 */ 00080 virtual void bind(void *ep); 00081 00082 /** 00083 Set the data wrapper 00084 @param data_wrapper input the data wrapper instance 00085 */ 00086 void setDataWrapper(DataWrapper *data_wrapper) { this->m_data_wrapper = data_wrapper; } 00087 00088 protected: 00089 DataWrapper *getDataWrapper() { return this->m_data_wrapper; } 00090 00091 private: 00092 DataWrapper *m_data_wrapper; 00093 M2MResource *m_res; 00094 }; 00095 00096 #endif // __STATIC_RESOURCE_H__ 00097
Generated on Thu Jul 14 2022 21:25:54 by
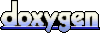