
Sample program showing how to connect GR-PEACH into Watson IoT through mbed Connector and Watson's Connector Bridge
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
StaticResource.cpp
00001 /** 00002 * @file StaticResource.h 00003 * @brief mbed CoAP Endpoint Static Resource class 00004 * @author Doug Anson/Chris Paola 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 // Class support 00024 #include "mbed-connector-interface/StaticResource.h" 00025 00026 // Endpoint 00027 #include "mbed-connector-interface/ConnectorEndpoint.h" 00028 00029 // Constructor 00030 StaticResource::StaticResource(const Logger *logger,const char *obj_name,const char *res_name, const char *value) : Resource<string>(logger,string(obj_name),string(res_name),string(value)), m_data_wrapper() 00031 { 00032 this->m_data_wrapper = NULL; 00033 this->m_res = NULL; 00034 } 00035 00036 // Constructor 00037 StaticResource::StaticResource(const Logger *logger,const char *obj_name,const char *res_name,const string value) : Resource<string>(logger,string(obj_name),string(res_name),string(value)) 00038 { 00039 this->m_data_wrapper = NULL; 00040 this->m_res = NULL; 00041 } 00042 00043 // Constructor with buffer lengths 00044 StaticResource::StaticResource(const Logger *logger,const string obj_name,const string res_name,const string value) : Resource<string>(logger,string(obj_name),string(res_name),string(value)) 00045 { 00046 this->m_data_wrapper = NULL; 00047 this->m_res = NULL; 00048 } 00049 00050 // Copy constructor 00051 StaticResource::StaticResource(const StaticResource &resource) : Resource<string>((const Resource<string> &)resource) 00052 { 00053 this->m_data_wrapper = resource.m_data_wrapper; 00054 this->m_res = resource.m_res; 00055 } 00056 00057 // Destructor 00058 StaticResource::~StaticResource() { 00059 } 00060 00061 // bind CoAP Resource.. 00062 void StaticResource::bind(void *ep) { 00063 // Static Resources nailed to STRING type... 00064 int type = (int)M2MResourceInstance::STRING; 00065 00066 // check our Endpoint instance... 00067 if (ep != NULL) { 00068 // cast 00069 Connector::Endpoint *endpoint = (Connector::Endpoint *)ep; 00070 00071 // get our ObjectInstanceManager 00072 ObjectInstanceManager *oim = endpoint->getObjectInstanceManager(); 00073 00074 if (this->getDataWrapper() != NULL) { 00075 // wrap the data... 00076 this->getDataWrapper()->wrap((uint8_t *)this->getValue().c_str(),(int)this->getValue().size()); 00077 00078 // Create our Resource 00079 this->m_res = (M2MResource *)oim->createStaticResourceInstance((char *)this->getObjName().c_str(),(char *)this->getResName().c_str(),(char *)"StaticResource",(int)type,(void *)this->getDataWrapper()->get(),(int)this->getDataWrapper()->length()); 00080 if (this->m_res != NULL) { 00081 // Record our Instance Number 00082 this->setInstanceNumber(oim->getLastCreatedInstanceNumber()); 00083 00084 // DEBUG 00085 this->logger()->logging("StaticResource: [%s] value: [%s] bound",this->getFullName().c_str(),this->getDataWrapper()->get()); 00086 } 00087 } 00088 else { 00089 // Create our Resource 00090 this->m_res = (M2MResource *)oim->createStaticResourceInstance((char *)this->getObjName().c_str(),(char *)this->getResName().c_str(),(char *)"StaticResource",(int)type,(void *)this->getValue().c_str(),(int)this->getValue().size()); 00091 if (this->m_res != NULL) { 00092 // Record our Instance Number 00093 this->setInstanceNumber(oim->getLastCreatedInstanceNumber()); 00094 00095 // DEBUG 00096 this->logger()->logging("StaticResource: [%s] value: [%s] bound",this->getFullName().c_str(),this->getValue().c_str()); 00097 } 00098 } 00099 } 00100 else { 00101 // no instance pointer to our endpoint 00102 this->logger()->logging("%s: NULL endpoint instance pointer in bind() request...",this->getFullName().c_str()); 00103 } 00104 }
Generated on Thu Jul 14 2022 21:25:54 by
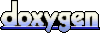