
Sample program showing how to connect GR-PEACH into Watson IoT through mbed Connector and Watson's Connector Bridge
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
DeviceManagementResponder.h
00001 /** 00002 * @file DeviceManagementResponder.h 00003 * @brief mbed CoAP Endpoint Device Management Responder class 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2016 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __DEVICE_MANAGEMENT_RESPONDER_H__ 00024 #define __DEVICE_MANAGEMENT_RESPONDER_H__ 00025 00026 // Logger 00027 #include "mbed-connector-interface/Logger.h" 00028 00029 // Authenticator Support 00030 #include "mbed-connector-interface/Authenticator.h" 00031 00032 // invocation handler signature 00033 typedef void (*initialize_fn)(const void *logger); 00034 typedef bool (*manifest_fn)(const void *ep,const void *logger,const void *manifest,uint32_t manifest_length); 00035 typedef bool (*image_set_fn)(const void *ep,const void *logger,const void *image,uint32_t image_length); 00036 typedef bool (*responder_fn)(const void *ep,const void *logger,const void *data); 00037 00038 class DeviceManagementResponder { 00039 public: 00040 /** 00041 Default constructor 00042 @param logger input logger instance 00043 @param authenticator input authentication instance 00044 */ 00045 DeviceManagementResponder(const Logger *logger,const Authenticator *authenticator); 00046 00047 /** 00048 Copy constructor 00049 @param resource input the DeviceManagementResponder that is to be deep copied 00050 */ 00051 DeviceManagementResponder(const DeviceManagementResponder &manager); 00052 00053 /** 00054 Destructor 00055 */ 00056 virtual ~DeviceManagementResponder(); 00057 00058 /** 00059 Set the Endpoint instance 00060 @param ep input the endpoint instance pointer 00061 */ 00062 void setEndpoint(const void *ep); 00063 00064 /** 00065 Set our Initialize handler function 00066 @param initialize_fn input the device initialize function pointer 00067 */ 00068 void setInitializeHandler(initialize_fn initialize_fn); 00069 00070 /** 00071 Set our Reboot Responder handler function 00072 @param reboot_responder_fn input the device reboot responder function pointer 00073 */ 00074 void setRebootResponderHandler(responder_fn reboot_responder_fn); 00075 00076 /** 00077 Set our Reset Responder handler function 00078 @param reset_responder_fn input the device reset responder function pointer 00079 */ 00080 void setResetResponderHandler(responder_fn reset_responder_fn); 00081 00082 /** 00083 Set our FOTA manifest handler function 00084 @param fota_manifest_fn input the FOTA manifest handler function pointer 00085 */ 00086 void setFOTAManifestHandler(manifest_fn fota_manifest_fn); 00087 00088 /** 00089 Set our FOTA image set handler function 00090 @param fota_image_set_fn input the FOTA image set function pointer 00091 */ 00092 void setFOTAImageHandler(image_set_fn fota_image_set_fn); 00093 00094 /** 00095 Set our FOTA invocation handler function 00096 @param fota_invocation_fn input the FOTA invocation handler function pointer 00097 */ 00098 void setFOTAInvocationHandler(responder_fn fota_invocation_fn); 00099 00100 /** 00101 ACTION: Deregister device 00102 @param challenge input the input authentication challenge 00103 */ 00104 virtual void deregisterDevice(const void *challenge); 00105 00106 /** 00107 ACTION: Reboot device 00108 @param challenge input the input authentication challenge 00109 */ 00110 virtual void rebootDevice(const void *challenge); 00111 00112 /** 00113 ACTION: Reset device 00114 @param challenge input the input authentication challenge 00115 */ 00116 virtual void resetDevice(const void *challenge); 00117 00118 /** 00119 Set our FOTA manifest 00120 @param manifest input the FOTA manifest 00121 @param manifest_length input the length of the FOTA manifest 00122 */ 00123 virtual void setFOTAManifest(char *manifest,uint32_t manifest_length); 00124 00125 /** 00126 Get our FOTA manifest 00127 @return the FOTA manifest 00128 */ 00129 virtual char *getFOTAManifest(); 00130 00131 /** 00132 Get our FOTA manifest length 00133 @return the FOTA manifest length 00134 */ 00135 virtual uint32_t getFOTAManifestLength(); 00136 00137 /** 00138 Set our FOTA image 00139 @param fota_image input the FOTA image 00140 @param fota_image_length input the length of the fota image 00141 */ 00142 virtual void setFOTAImage(void *fota_image,uint32_t fota_image_length); 00143 00144 /** 00145 Get our FOTA image 00146 @return the FOTA image 00147 */ 00148 virtual void *getFOTAImage(); 00149 00150 /** 00151 Get our FOTA image length 00152 @return the FOTA image length 00153 */ 00154 virtual uint32_t getFOTAImageLength(); 00155 00156 /** 00157 ACTION: Invoke FOTA (default: empty action) 00158 @param challenge input the input authentication challenge 00159 */ 00160 virtual void invokeFOTA(const void *challenge); 00161 00162 private: 00163 Logger *m_logger; 00164 Authenticator *m_authenticator; 00165 void *m_endpoint; 00166 char *m_manifest; 00167 int m_manifest_length; 00168 void *m_fota_image; 00169 uint32_t m_fota_image_length; 00170 00171 initialize_fn m_initialize_fn; 00172 responder_fn m_reboot_responder_fn; 00173 responder_fn m_reset_responder_fn; 00174 manifest_fn m_fota_manifest_fn; 00175 image_set_fn m_fota_image_set_fn; 00176 responder_fn m_fota_invocation_fn; 00177 00178 bool authenticate(const void *challenge); 00179 }; 00180 00181 #endif // __DEVICE_MANAGEMENT_RESPONDER_H__
Generated on Thu Jul 14 2022 21:25:54 by
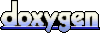