
Hello World for JCU(JPEG Codec Unit). JCU is JPEG codec unit of RZ/A1. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
main.cpp
00001 #include "mbed.h" 00002 #include "JPEG_Converter.h" 00003 #include "FATFileSystem.h" 00004 #include "USBHostMSD.h" 00005 00006 #define SAMPLE_WIDTH (320) 00007 #define SAMPLE_HEIGHT (240) 00008 #define SAMPLE_BUFF_SIZE (SAMPLE_WIDTH * SAMPLE_HEIGHT * 4) 00009 00010 #define MAX_FILE_NAME_LENGTH (64) 00011 #define FOLD_COUNT (5) 00012 00013 DigitalOut led1(LED1); 00014 Serial pc(USBTX, USBRX); 00015 00016 #if defined(__ICCARM__) 00017 #pragma data_alignment=32 00018 static uint8_t JCUBuffer_INPUT[SAMPLE_BUFF_SIZE]@ ".mirrorram"; //32 bytes aligned!; 00019 #pragma data_alignment=32 00020 static uint8_t JCUBuffer_OUTPUT[SAMPLE_BUFF_SIZE]@ ".mirrorram"; //32 bytes aligned!; 00021 #else 00022 static uint8_t JCUBuffer_INPUT[SAMPLE_BUFF_SIZE]__attribute((section("NC_BSS"),aligned(32))); //32 bytes aligned!; 00023 static uint8_t JCUBuffer_OUTPUT[SAMPLE_BUFF_SIZE]__attribute((section("NC_BSS"),aligned(32))); //32 bytes aligned!; 00024 #endif 00025 00026 bool get_file_name(char * p_buf, int size) { 00027 bool ret; 00028 char ch; 00029 int i = 0; 00030 00031 while (1) { 00032 ch = (char)pc.getc(); 00033 if (ch == '\r'){ 00034 p_buf[i] = '\0'; 00035 pc.putc('\n'); 00036 ret = true; 00037 break; 00038 } else if (ch == '\n') { 00039 // Do Nothing 00040 } else if (ch == '\b') { 00041 if (i > 0) { 00042 pc.puts("\b \b"); 00043 i--; 00044 p_buf[i] = '\0'; 00045 } 00046 } else if (i < size) { 00047 p_buf[i] = ch; 00048 i++; 00049 pc.putc(ch); 00050 } else { 00051 ret = false; 00052 break; 00053 } 00054 } 00055 00056 return ret; 00057 } 00058 00059 int main() { 00060 char file_name[MAX_FILE_NAME_LENGTH + FOLD_COUNT]; 00061 JPEG_Converter decoder; 00062 JPEG_Converter::bitmap_buff_info_t aBitmapData; 00063 FILE * rd_fp = NULL; 00064 FILE * wr_fp = NULL; 00065 int filesize; 00066 size_t EncodeSize; 00067 00068 FATFileSystem fs("usb"); 00069 USBHostMSD msd; 00070 00071 file_name[0] = '/'; 00072 file_name[1] = 'u'; 00073 file_name[2] = 's'; 00074 file_name[3] = 'b'; 00075 file_name[4] = '/'; 00076 00077 //try to connect a MSD device 00078 while(!msd.connect()) { 00079 ThisThread::sleep_for(500); 00080 } 00081 00082 //Now that USB flash disk is detected, file system is mounted 00083 fs.mount(&msd); 00084 00085 pc.printf("%dpix x %dpix\n", SAMPLE_WIDTH, SAMPLE_HEIGHT); 00086 00087 while (1) { 00088 pc.printf("\nInput file name (.jpg or .bin)>"); 00089 if (get_file_name(&file_name[FOLD_COUNT], MAX_FILE_NAME_LENGTH) == false) { 00090 pc.printf("Error:Max file name length is %d\n", MAX_FILE_NAME_LENGTH); 00091 } else { 00092 size_t len = strlen(file_name); 00093 if ((len > 4) && (file_name[len - 4] == (char)'.') 00094 && ((file_name[len - 3] | 0x20u) == (char)'j') 00095 && ((file_name[len - 2] | 0x20u) == (char)'p') 00096 && ((file_name[len - 1] | 0x20u) == (char)'g')) { 00097 // input ".jpg" 00098 // decode JPG file to bitmap file 00099 rd_fp = fopen(file_name, "r"); 00100 if (rd_fp == NULL) { 00101 pc.printf("Error:File is not exist \n"); 00102 } else { 00103 fseek(rd_fp, 0, SEEK_END); 00104 filesize = ftell(rd_fp); 00105 if (filesize > SAMPLE_BUFF_SIZE) { 00106 pc.printf("Error:File size over \n"); 00107 } else { 00108 led1 = 1; 00109 fseek(rd_fp, 0, SEEK_SET); 00110 fread(&JCUBuffer_INPUT[0], sizeof(char), filesize, rd_fp); 00111 pc.printf("[Decode Mode]\n"); 00112 pc.printf("\nOutput file name (.bin)>"); 00113 if (get_file_name(&file_name[FOLD_COUNT], MAX_FILE_NAME_LENGTH) == false) { 00114 pc.printf("Error:Max file name length is %d\n", MAX_FILE_NAME_LENGTH); 00115 } else { 00116 //YCbCr setting 00117 aBitmapData.width = SAMPLE_WIDTH; 00118 aBitmapData.height = SAMPLE_HEIGHT; 00119 aBitmapData.format = JPEG_Converter::WR_RD_YCbCr422; //YCbCr[0] & ARGB8888[1] is 4byte, not RGB565[2] is 2byte 00120 aBitmapData.buffer_address = (void *)JCUBuffer_OUTPUT; 00121 pc.printf("File decode start\n"); 00122 // JPEG_Converter 00123 if (decoder.decode((void *)JCUBuffer_INPUT, &aBitmapData) == JPEG_Converter::JPEG_CONV_OK) { 00124 pc.printf("File decode done %dbyte\n", (SAMPLE_WIDTH * SAMPLE_HEIGHT * 4)); 00125 pc.printf("File write start\n"); 00126 wr_fp = fopen(file_name, "w"); 00127 fwrite(JCUBuffer_OUTPUT, sizeof(char), (SAMPLE_WIDTH * SAMPLE_HEIGHT * 4), wr_fp); 00128 00129 fclose(wr_fp); 00130 pc.printf("File write done\n"); 00131 led1 = 0; 00132 } else { 00133 pc.printf("Error:JCU decode error\n"); 00134 led1 = 0; 00135 } 00136 } 00137 } 00138 fclose(rd_fp); 00139 } 00140 } else if ((file_name[len - 4] == (char)'.') 00141 && ((file_name[len - 3] | 0x20u) == (char)'b') 00142 && ((file_name[len - 2] | 0x20u) == (char)'i') 00143 && ((file_name[len - 1] | 0x20u) == (char)'n')) { 00144 // input ".bin" 00145 // encode bitmap file to JPEG file 00146 rd_fp = fopen(file_name, "r"); 00147 if (rd_fp == NULL) { 00148 pc.printf("Error:File is not exist\n"); 00149 } else { 00150 fseek(rd_fp, 0, SEEK_END); 00151 filesize = ftell(rd_fp); 00152 if (filesize > SAMPLE_BUFF_SIZE) { 00153 fclose(wr_fp); 00154 pc.printf("Error:File size over\n"); 00155 } else { 00156 led1 = 1; 00157 fseek(rd_fp, 0, SEEK_SET); 00158 fread(&JCUBuffer_INPUT[0], sizeof(char), filesize, rd_fp); 00159 pc.printf("[Encode Mode]\n"); 00160 pc.printf("\nOutput file name (.jpg)>"); 00161 if (get_file_name(&file_name[FOLD_COUNT], MAX_FILE_NAME_LENGTH) == false) { 00162 pc.printf("Error:Max file name length is %d\n", MAX_FILE_NAME_LENGTH); 00163 fclose(rd_fp); 00164 } else { 00165 //YCbCr setting 00166 aBitmapData.width = SAMPLE_WIDTH; 00167 aBitmapData.height = SAMPLE_HEIGHT; 00168 aBitmapData.format = JPEG_Converter::WR_RD_YCbCr422; //YCbCr[0] & ARGB8888[1] is 4byte, not RGB565[2] is 2byte 00169 aBitmapData.buffer_address = (void *)JCUBuffer_INPUT; 00170 pc.printf("File encode start\n"); 00171 // JPEG_Converter 00172 if (decoder.encode(&aBitmapData, JCUBuffer_OUTPUT, &EncodeSize) == JPEG_Converter::JPEG_CONV_OK) { 00173 pc.printf("File encode done %dbyte\n", EncodeSize); 00174 pc.printf("File write start\n"); 00175 wr_fp = fopen(file_name, "w"); 00176 fwrite(JCUBuffer_OUTPUT, sizeof(char), EncodeSize, wr_fp); 00177 00178 fclose(wr_fp); 00179 pc.printf("File write done\n"); 00180 led1 = 0; 00181 } else { 00182 pc.printf("Error:JCU encode error\n"); 00183 led1 = 0; 00184 } 00185 } 00186 } 00187 fclose(rd_fp); 00188 } 00189 } else { 00190 pc.printf("Error:Not supported extension (.jpg or .bin)\n"); 00191 } 00192 } 00193 } 00194 }
Generated on Thu Jul 14 2022 00:57:17 by
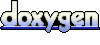