
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
main.cpp
00001 #include "mbed.h" 00002 #include "EasyAttach_CameraAndLCD.h" 00003 #include "recognition_proc.h" 00004 #include "touch_proc.h" 00005 00006 static DisplayBase Display; 00007 static Thread recognitionTask(osPriorityNormal, 1024 * 8); 00008 static Thread touchTask; 00009 00010 /****** LCD ******/ 00011 static void IntCallbackFunc_LoVsync(DisplayBase::int_type_t int_type) { 00012 /* Interrupt callback function for Vsync interruption */ 00013 touch_lcd_int(int_type); 00014 } 00015 00016 /****** main ******/ 00017 int main(void) { 00018 /* Initialization of LCD */ 00019 EasyAttach_Init(Display); 00020 00021 /* Interrupt callback function setting (Vsync signal output from scaler 0) */ 00022 Display.Graphics_Irq_Handler_Set(DisplayBase::INT_TYPE_S0_LO_VSYNC, 0, IntCallbackFunc_LoVsync); 00023 00024 /* Start recognition processing */ 00025 recognitionTask.start(callback(recognition_task, &Display)); 00026 00027 /* Start touch panel processing */ 00028 touchTask.start(callback(touch_task, &Display)); 00029 00030 /* Backlight on */ 00031 ThisThread::sleep_for(200); 00032 EasyAttach_LcdBacklight(true); 00033 00034 /* Wait for the threads to finish */ 00035 recognitionTask.join(); 00036 touchTask.join(); 00037 }
Generated on Fri Jul 15 2022 01:15:47 by
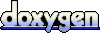