
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
STBTracking.c
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #include "STBTracking.h" 00018 #include "STB_Debug.h" 00019 00020 /*------------------------------------------------------------------------------------------------------------------*/ 00021 /* SetTrackingObjectBody */ 00022 /*------------------------------------------------------------------------------------------------------------------*/ 00023 VOID SetTrackingObjectBody(const STB_FRAME_RESULT_BODYS* stbINPUTbodys,TraObj *bodys) 00024 { 00025 00026 STB_INT32 nCount; 00027 STB_INT32 i; 00028 00029 00030 /*make the human body information*/ 00031 nCount = stbINPUTbodys->nCount; 00032 00033 for ( i = 0; i < nCount; i++) 00034 { 00035 bodys[i].nDetID = i; 00036 bodys[i].pos.x = stbINPUTbodys->body[i].center.nX ; 00037 bodys[i].pos.y = stbINPUTbodys->body[i].center.nY ; 00038 bodys[i].conf = stbINPUTbodys->body[i].nConfidence; 00039 bodys[i].size = stbINPUTbodys->body[i].nSize ; 00040 bodys[i].nTraID = STB_STATUS_NO_DATA; 00041 } 00042 00043 } 00044 /*------------------------------------------------------------------------------------------------------------------*/ 00045 /* SetTrackingObjectFace */ 00046 /*------------------------------------------------------------------------------------------------------------------*/ 00047 VOID SetTrackingObjectFace ( const STB_FRAME_RESULT_FACES *stbINPUTfaces ,TraObj *faces ) 00048 { 00049 00050 STB_INT32 nCount; 00051 STB_INT32 i; 00052 00053 00054 /*make the human body information*/ 00055 nCount = stbINPUTfaces->nCount; 00056 for ( i = 0; i < nCount; i++) 00057 { 00058 faces[i].nDetID = i; 00059 faces[i].pos.x = stbINPUTfaces->face[i].center.nX ; 00060 faces[i].pos.y = stbINPUTfaces->face[i].center.nY ; 00061 faces[i].conf = stbINPUTfaces->face[i].nConfidence; 00062 faces[i].size = stbINPUTfaces->face[i].nSize ; 00063 faces[i].nTraID = STB_STATUS_NO_DATA; 00064 } 00065 00066 } 00067 /*------------------------------------------------------------------------------------------------------------------*/ 00068 /* SetSrcTrFace */ 00069 /*------------------------------------------------------------------------------------------------------------------*/ 00070 VOID SetSrcTrFace ( STB_INT32 nDetCntFace , TraObj *trFace, STB_TR_DET *trSrcInfo) 00071 { 00072 STB_INT32 i; 00073 00074 trSrcInfo->fcNum = nDetCntFace; 00075 for( i = 0; i < nDetCntFace; i++) 00076 { 00077 trSrcInfo->fcDet[i].conf = trFace[i].conf ; 00078 trSrcInfo->fcDet[i].posX = trFace[i].pos .x ; 00079 trSrcInfo->fcDet[i].posY = trFace[i].pos .y ; 00080 trSrcInfo->fcDet[i].size = trFace[i].size ; 00081 } 00082 } 00083 /*------------------------------------------------------------------------------------------------------------------*/ 00084 /* SetSrcTrBody */ 00085 /*------------------------------------------------------------------------------------------------------------------*/ 00086 VOID SetSrcTrBody ( STB_INT32 nDetCntBody , TraObj *trBody, STB_TR_DET *trSrcInfo) 00087 { 00088 STB_INT32 i; 00089 00090 trSrcInfo->bdNum = nDetCntBody; 00091 00092 for( i = 0; i < nDetCntBody; i++) 00093 { 00094 trSrcInfo->bdDet[i].conf = trBody[i].conf ; 00095 trSrcInfo->bdDet[i].posX = trBody[i].pos .x ; 00096 trSrcInfo->bdDet[i].posY = trBody[i].pos .y ; 00097 trSrcInfo->bdDet[i].size = trBody[i].size ; 00098 } 00099 } 00100 00101 /*------------------------------------------------------------------------------------------------------------------*/ 00102 /* SetTrackingInfoToFace : Reflect tracking result in structure of detection result */ 00103 /*------------------------------------------------------------------------------------------------------------------*/ 00104 VOID SetTrackingInfoToFace( STB_TR_RES_FACES *fdResult ,STB_INT32 *pnTrackingNum ,TraObj *faces ) 00105 { 00106 STB_INT32 nIdx; 00107 00108 *pnTrackingNum = fdResult->cnt; 00109 for (nIdx = 0; nIdx < *pnTrackingNum; nIdx++) 00110 { 00111 faces[nIdx].nDetID = fdResult->face[nIdx].nDetID ; 00112 faces[nIdx].nTraID = fdResult->face[nIdx].nTraID ; 00113 faces[nIdx].pos .x = fdResult->face[nIdx].pos.x ; 00114 faces[nIdx].pos .y = fdResult->face[nIdx].pos.y ; 00115 faces[nIdx].size = fdResult->face[nIdx].size ; 00116 faces[nIdx].conf = fdResult->face[nIdx].conf ; 00117 } 00118 00119 return; 00120 } 00121 00122 VOID SetTrackingInfoToBody(STB_TR_RES_BODYS *bdResult,STB_INT32 *pnTrackingNum,TraObj *bodys) 00123 { 00124 STB_INT32 nIdx; 00125 00126 *pnTrackingNum = bdResult->cnt; 00127 for (nIdx = 0; nIdx < *pnTrackingNum; nIdx++) 00128 { 00129 bodys[nIdx].nDetID = bdResult->body[nIdx].nDetID ; 00130 bodys[nIdx].nTraID = bdResult->body[nIdx].nTraID ; 00131 bodys[nIdx].pos .x = bdResult->body[nIdx].pos.x ; 00132 bodys[nIdx].pos .y = bdResult->body[nIdx].pos.y ; 00133 bodys[nIdx].size = bdResult->body[nIdx].size ; 00134 bodys[nIdx].conf = bdResult->body[nIdx].conf ; 00135 } 00136 00137 return; 00138 }
Generated on Fri Jul 15 2022 01:15:47 by
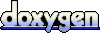