
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
STBPeAPI.c
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #include "STBPeAPI.h" 00018 00019 00020 /*---------------------------------------------------------------------*/ 00021 // PeSlideFacesRec 00022 /*---------------------------------------------------------------------*/ 00023 void PeSlideFacesRec ( STB_PE_DET *facesRec , STBExecFlg *execFlg) 00024 { 00025 STB_INT32 t , i ,j; 00026 00027 for( t = STB_PE_BACK_MAX -2 ; t >= 0 ; t-- ) 00028 { 00029 facesRec [ t + 1 ].num = facesRec[ t + 0 ].num; 00030 for( i = 0 ; i < facesRec [ t + 1 ].num ; i++ ) 00031 { 00032 facesRec[ t + 1 ].fcDet[i].nDetID = facesRec[ t ].fcDet[i].nDetID ; 00033 facesRec[ t + 1 ].fcDet[i].nTraID = facesRec[ t ].fcDet[i].nTraID ; 00034 if( execFlg->gen == STB_TRUE ) 00035 { 00036 facesRec[ t + 1 ].fcDet[i].genDetVal = facesRec[ t ].fcDet[i].genDetVal ; 00037 facesRec[ t + 1 ].fcDet[i].genStatus = facesRec[ t ].fcDet[i].genStatus ; 00038 facesRec[ t + 1 ].fcDet[i].genDetConf = facesRec[ t ].fcDet[i].genDetConf ; 00039 } 00040 if( execFlg->age == STB_TRUE ) 00041 { 00042 facesRec[ t + 1 ].fcDet[i].ageDetVal = facesRec[ t ].fcDet[i].ageDetVal ; 00043 facesRec[ t + 1 ].fcDet[i].ageStatus = facesRec[ t ].fcDet[i].ageStatus ; 00044 facesRec[ t + 1 ].fcDet[i].ageDetConf = facesRec[ t ].fcDet[i].ageDetConf ; 00045 } 00046 if( execFlg->exp == STB_TRUE ) 00047 { 00048 facesRec[ t + 1 ].fcDet[i].expDetConf = facesRec[ t ].fcDet[i].expDetConf ; 00049 for( j = 0 ; j < STB_EX_MAX ; j++) 00050 { 00051 facesRec[ t + 1 ].fcDet[ i ].expDetVal[ j ] 00052 = facesRec[ t + 0 ].fcDet[ i ].expDetVal[ j ]; 00053 } 00054 } 00055 if( execFlg->gaz == STB_TRUE ) 00056 { 00057 facesRec[ t + 1 ].fcDet[i].gazDetLR = facesRec[ t ].fcDet[i].gazDetLR ; 00058 facesRec[ t + 1 ].fcDet[i].gazDetUD = facesRec[ t ].fcDet[i].gazDetUD ; 00059 } 00060 //if( execFlg->dir == STB_TRUE ) 00061 //{ 00062 facesRec[ t + 1 ].fcDet[i].dirDetRoll = facesRec[ t ].fcDet[i].dirDetRoll ; 00063 facesRec[ t + 1 ].fcDet[i].dirDetPitch = facesRec[ t ].fcDet[i].dirDetPitch; 00064 facesRec[ t + 1 ].fcDet[i].dirDetYaw = facesRec[ t ].fcDet[i].dirDetYaw ; 00065 facesRec[ t + 1 ].fcDet[i].dirDetConf = facesRec[ t ].fcDet[i].dirDetConf ; 00066 //} 00067 if( execFlg->bli == STB_TRUE ) 00068 { 00069 facesRec[ t + 1 ].fcDet[i].bliDetL = facesRec[ t ].fcDet[i].bliDetL ; 00070 facesRec[ t + 1 ].fcDet[i].bliDetR = facesRec[ t ].fcDet[i].bliDetR ; 00071 } 00072 } 00073 } 00074 00075 } 00076 /*---------------------------------------------------------------------*/ 00077 // PeCurFaces 00078 /*---------------------------------------------------------------------*/ 00079 void PeCurFaces ( STB_PE_DET *facesRec , STB_PE_DET *srcFace ,STBExecFlg *execFlg) 00080 { 00081 STB_INT32 i ,j; 00082 00083 00084 facesRec [ 0 ].num = srcFace->num; 00085 for( i = 0 ; i < facesRec [ 0 ].num ; i++ ) 00086 { 00087 facesRec[ 0 ].fcDet[ i ].nDetID = srcFace[ 0 ].fcDet[ i ].nDetID ; 00088 facesRec[ 0 ].fcDet[ i ].nTraID = srcFace[ 0 ].fcDet[ i ].nTraID ; 00089 if( execFlg->gen == STB_TRUE ) 00090 { 00091 facesRec[ 0 ].fcDet[ i ].genDetVal = srcFace[ 0 ].fcDet[ i ].genDetVal ; 00092 facesRec[ 0 ].fcDet[ i ].genStatus = STB_STATUS_NO_DATA ; 00093 facesRec[ 0 ].fcDet[ i ].genDetConf = srcFace[ 0 ].fcDet[ i ].genDetConf; 00094 } 00095 if( execFlg->age == STB_TRUE ) 00096 { 00097 facesRec[ 0 ].fcDet[ i ].ageDetVal = srcFace[ 0 ].fcDet[ i ].ageDetVal ; 00098 facesRec[ 0 ].fcDet[ i ].ageStatus = STB_STATUS_NO_DATA ; 00099 facesRec[ 0 ].fcDet[ i ].ageDetConf = srcFace[ 0 ].fcDet[ i ].ageDetConf; 00100 } 00101 if( execFlg->exp == STB_TRUE ) 00102 { 00103 facesRec[ 0 ].fcDet[ i ].expDetConf = srcFace[ 0 ].fcDet[ i ].expDetConf ; 00104 for( j = 0 ; j < STB_EX_MAX ; j++) 00105 { 00106 facesRec[ 0 ].fcDet[ i ].expDetVal[ j] = srcFace[ 0 ].fcDet[ i ].expDetVal[ j]; 00107 } 00108 } 00109 if( execFlg->gaz == STB_TRUE ) 00110 { 00111 facesRec[ 0 ].fcDet[ i ].gazDetLR = srcFace[ 0 ].fcDet[ i ].gazDetLR ; 00112 facesRec[ 0 ].fcDet[ i ].gazDetUD = srcFace[ 0 ].fcDet[ i ].gazDetUD ; 00113 } 00114 //if( execFlg->dir == STB_TRUE ) 00115 //{ 00116 facesRec[ 0 ].fcDet[ i ].dirDetRoll = srcFace[ 0 ].fcDet[ i ].dirDetRoll ; 00117 facesRec[ 0 ].fcDet[ i ].dirDetYaw = srcFace[ 0 ].fcDet[ i ].dirDetYaw ; 00118 facesRec[ 0 ].fcDet[ i ].dirDetPitch = srcFace[ 0 ].fcDet[ i ].dirDetPitch ; 00119 facesRec[ 0 ].fcDet[ i ].dirDetConf = srcFace[ 0 ].fcDet[ i ].dirDetConf ; 00120 //} 00121 if( execFlg->bli == STB_TRUE ) 00122 { 00123 facesRec[ 0 ].fcDet[ i ].bliDetL = srcFace[ 0 ].fcDet[ i ].bliDetL ; 00124 facesRec[ 0 ].fcDet[ i ].bliDetR = srcFace[ 0 ].fcDet[ i ].bliDetR ; 00125 } 00126 } 00127 } 00128 00129 /*----------------------------------------------------------------------------------------------------*/ 00130 /* PeExpressID */ 00131 /*----------------------------------------------------------------------------------------------------*/ 00132 STB_INT32 PeExpressID( STB_INT32* exp ) 00133 { 00134 int i; 00135 int tmpVal; 00136 int retVal; 00137 00138 retVal = 0; 00139 tmpVal = 0; 00140 for( i = 0 ; i < STB_EX_MAX ; i++) 00141 { 00142 if( tmpVal < exp[i] && exp[i] != STB_ERR_PE_CANNOT ) 00143 { 00144 tmpVal = exp[i]; 00145 retVal = i; 00146 } 00147 } 00148 return retVal; 00149 } 00150 /*---------------------------------------------------------------------*/ 00151 // PeStbFaceEasy 00152 /*---------------------------------------------------------------------*/ 00153 void PeStbFaceEasy 00154 ( 00155 STB_PE_RES *peRes , 00156 STB_PE_DET *peRec , 00157 STB_INT32 dirThr , 00158 STB_INT32 dirUDMax , 00159 STB_INT32 dirUDMin , 00160 STB_INT32 dirLRMax , 00161 STB_INT32 dirLRMin , 00162 STB_INT32 frmMax , 00163 STBExecFlg *execFlg 00164 ) 00165 { 00166 00167 00168 /*Checking the past data here, fill in all peRes.*/ 00169 STB_INT32 k ,t,i ; 00170 STB_INT32 trID; 00171 00172 STB_INT32 recCnt; 00173 STB_INT32 recVal [STB_PE_BACK_MAX]; 00174 STB_INT32 recConf [STB_PE_BACK_MAX]; 00175 STB_INT32 tmpVal; 00176 STB_INT32 tmpConf; 00177 STB_INT32 expVal [STB_EX_MAX] = {0}; 00178 00179 STB_STATUS preSAge ; 00180 STB_STATUS preSGen ; 00181 STB_INT32 preVAge ; 00182 STB_INT32 preVGen ; 00183 STB_INT32 preCAge ; 00184 STB_INT32 preCGen ; 00185 STB_STATUS tmpS ; 00186 00187 00188 00189 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00190 { 00191 recVal [t] = 0; 00192 recConf[t] = 0; 00193 } 00194 00195 00196 /*do stabilization processing each tracking person*/ 00197 peRes->peCnt = peRec[0].num ;//a number of tracking people(present) 00198 for( k = 0; k < peRes->peCnt ; k++) 00199 { 00200 trID = peRec[0].fcDet[k].nTraID;/*Tracking person number in the through frame*/ 00201 00202 00203 // peRes Add ------------------------------------------------------------------------------------------------- 00204 peRes->peFace[k].nTraID = trID; 00205 00206 00207 // preStatus ------------------------------------------------------------------------------------------------- 00208 preSAge = STB_STATUS_NO_DATA ; 00209 preSGen = STB_STATUS_NO_DATA ; 00210 preVAge = 0 ; 00211 preVGen = 0 ; 00212 preCAge = 0 ; 00213 preCGen = 0 ; 00214 if( execFlg->age == STB_TRUE || execFlg->gen == STB_TRUE ) 00215 { 00216 for( i = 0; i < peRec[1].num ; i++) 00217 { 00218 if( peRec[1].fcDet[i].nTraID == trID ) 00219 { 00220 preSAge = peRec[1].fcDet[i].ageStatus ; 00221 preSGen = peRec[1].fcDet[i].genStatus ; 00222 preVAge = peRec[1].fcDet[i].ageDetVal ; 00223 preVGen = peRec[1].fcDet[i].genDetVal ; 00224 preCAge = peRec[1].fcDet[i].ageDetConf ; 00225 preCGen = peRec[1].fcDet[i].genDetConf ; 00226 break; 00227 } 00228 } 00229 } 00230 00231 // age ------------------------------------------------------------------------------------------------- 00232 if( execFlg->age == STB_TRUE ) 00233 { 00234 if ( preSAge == STB_STATUS_NO_DATA //stabilization impossible: no data of the relevant people 00235 || preSAge == STB_STATUS_CALCULATING //during stabilization : a number of data for relevant people aren't enough(a number of frames that relevant people are taken) 00236 ) 00237 { 00238 recCnt = 0; 00239 for( t = 0; t < STB_PE_BACK_MAX ; t++) //previous t frame 00240 { 00241 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00242 { 00243 if( 00244 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00245 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00246 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00247 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00248 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00249 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00250 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00251 && peRec[t].fcDet[i].ageDetVal != STB_ERR_PE_CANNOT // 00252 && peRec[t].fcDet[i].ageDetConf != STB_ERR_PE_CANNOT // 00253 ) 00254 { 00255 recVal [ recCnt ] = peRec[ t ].fcDet[ i ].ageDetVal ; 00256 recConf[ recCnt ] = peRec[ t ].fcDet[ i ].ageDetConf ; 00257 recCnt++; 00258 } 00259 } 00260 } 00261 tmpS = STB_STATUS_NO_DATA; 00262 if ( recCnt == 0 ) { tmpS = STB_STATUS_NO_DATA ; }//stabilization impossible 00263 else if ( recCnt < frmMax ) { tmpS = STB_STATUS_CALCULATING; }//during stabilization 00264 else if ( recCnt >= frmMax ) { tmpS = STB_STATUS_COMPLETE ; }//Just after stabilization 00265 tmpVal = 0; 00266 tmpConf = 0; 00267 for( i = 0; i < recCnt ; i++ ) 00268 { 00269 tmpVal += recVal [ i ] ; 00270 tmpConf += recConf[ i ] ; 00271 } 00272 if ( recCnt == 0 ) { recCnt = 1 ; } 00273 tmpVal /= recCnt; 00274 tmpConf /= recCnt; 00275 peRes->peFace[k].age.value = tmpVal ; 00276 peRes->peFace[k].age.conf = STB_CONF_NO_DATA ; 00277 peRes->peFace[k].age.status = tmpS ; 00278 peRec[0].fcDet[k].ageStatus = tmpS ; 00279 if( tmpS == STB_STATUS_COMPLETE )//Just after stabilization 00280 { 00281 peRec[0].fcDet[k].ageDetVal = tmpVal ; 00282 peRec[0].fcDet[k].ageDetConf = tmpConf ; 00283 } 00284 }else if ( preSAge == STB_STATUS_COMPLETE //Just after stabilization 00285 || preSAge == STB_STATUS_FIXED //already stabilized 00286 ) 00287 { 00288 peRes->peFace[k].age.value = preVAge ; 00289 peRes->peFace[k].age.conf = preCAge; 00290 peRes->peFace[k].age.status = STB_STATUS_FIXED ;//already stabilized 00291 peRec[0].fcDet[k].ageDetVal = preVAge ; 00292 peRec[0].fcDet[k].ageDetConf = preCAge ; 00293 peRec[0].fcDet[k].ageStatus = STB_STATUS_FIXED ;//already stabilized 00294 } 00295 } 00296 00297 00298 // gender ------------------------------------------------------------------------------------------------- 00299 if( execFlg->gen == STB_TRUE ) 00300 { 00301 if ( preSGen == STB_STATUS_NO_DATA //stabilization impossible: no data of the relevant people 00302 || preSGen == STB_STATUS_CALCULATING //during stabilization : a number of data for relevant people aren't enough(a number of frames that relevant people are taken) 00303 ) 00304 { 00305 recCnt = 0; 00306 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00307 { 00308 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00309 { 00310 if( 00311 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00312 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00313 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00314 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00315 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00316 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00317 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00318 && peRec[t].fcDet[i].genDetVal != STB_ERR_PE_CANNOT // 00319 && peRec[t].fcDet[i].genDetConf != STB_ERR_PE_CANNOT // 00320 ) 00321 { 00322 recVal [ recCnt ] = peRec[ t ].fcDet[ i ].genDetVal ;// 1:man 0:woman 00323 recConf[ recCnt ] = peRec[ t ].fcDet[ i ].genDetConf; 00324 recCnt++; 00325 } 00326 } 00327 } 00328 tmpS = STB_STATUS_NO_DATA; 00329 if ( recCnt == 0 ) { tmpS = STB_STATUS_NO_DATA ; }//stabilization impossible 00330 else if ( recCnt < frmMax ) { tmpS = STB_STATUS_CALCULATING; }//during stabilization 00331 else if ( recCnt >= frmMax ) { tmpS = STB_STATUS_COMPLETE ; }//Just after stabilization 00332 tmpVal = 0; 00333 tmpConf = 0; 00334 for( i = 0; i < recCnt ; i++ ) 00335 { 00336 tmpVal += recVal [ i ] ; 00337 tmpConf += recConf[ i ] ; 00338 } 00339 if ( recCnt == 0 ) { recCnt = 1 ; } 00340 tmpConf /= recCnt; 00341 if ( tmpVal * 2 <= recCnt ) 00342 { 00343 peRes->peFace[k].gen.value = 0 ;// 1:man 0:woman 00344 peRes->peFace[k].gen.status = tmpS ; 00345 peRes->peFace[k].gen.conf = STB_CONF_NO_DATA ; 00346 peRec[0].fcDet[k].genStatus = tmpS ; 00347 if( tmpS == STB_STATUS_COMPLETE )//Just after stabilization 00348 { 00349 peRec[0].fcDet[k].genDetVal = 0 ; 00350 peRec[0].fcDet[k].genDetConf = tmpConf ; 00351 } 00352 } 00353 else 00354 { 00355 peRes->peFace[k].gen.value = 1 ;// 1:man 0:woman 00356 peRes->peFace[k].gen.status = tmpS ; 00357 peRes->peFace[k].gen.conf = STB_CONF_NO_DATA ; 00358 peRec[0].fcDet[k].genStatus = tmpS ; 00359 if( tmpS == STB_STATUS_COMPLETE )//Just after stabilization 00360 { 00361 peRec[0].fcDet[k].genDetVal = 1 ; 00362 peRec[0].fcDet[k].genDetConf = tmpConf ; 00363 } 00364 } 00365 }else if ( preSGen == STB_STATUS_COMPLETE //Just after stabilization 00366 || preSGen == STB_STATUS_FIXED //already stabilized 00367 ) 00368 { 00369 peRes->peFace[k].gen.value = preVGen ; 00370 peRes->peFace[k].gen.conf = preCGen; 00371 peRes->peFace[k].gen.status = STB_STATUS_FIXED ;//already stabilized 00372 peRec[0].fcDet[k].genDetVal = preVGen ; 00373 peRec[0].fcDet[k].genStatus = STB_STATUS_FIXED ;//already stabilized 00374 peRec[0].fcDet[k].genDetConf = preCGen ; 00375 } 00376 } 00377 00378 // gazeLR ------------------------------------------------------------------------------------------------- 00379 if( execFlg->gaz == STB_TRUE ) 00380 { 00381 recCnt = 0; 00382 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00383 { 00384 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00385 { 00386 if( 00387 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00388 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00389 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00390 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00391 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00392 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00393 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00394 && peRec[t].fcDet[i].gazDetLR != STB_ERR_PE_CANNOT // 00395 ) 00396 { 00397 recVal[ recCnt ] = peRec[ t ].fcDet [ i ].gazDetLR ; 00398 recCnt++; 00399 } 00400 } 00401 } 00402 if ( recCnt == 0 ) { peRes->peFace[k].gaz.status = STB_STATUS_NO_DATA ; }//stabilization impossible 00403 else { peRes->peFace[k].gaz.status = STB_STATUS_CALCULATING; }//during stabilization 00404 peRes->peFace[k].gaz.conf = STB_CONF_NO_DATA;//no Confidence 00405 tmpVal = 0; 00406 for( i = 0; i < recCnt ; i++ ) { tmpVal += recVal[ i ] ; } 00407 if ( recCnt == 0 ) { recCnt = 1 ; } 00408 peRes->peFace[k].gaz.LR = tmpVal / recCnt; 00409 // gazeUD ------------------------------------------------------------------------------------------------- 00410 recCnt = 0; 00411 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00412 { 00413 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00414 { 00415 if( 00416 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00417 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00418 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00419 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00420 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00421 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00422 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00423 && peRec[t].fcDet[i].gazDetUD != STB_ERR_PE_CANNOT // 00424 ) 00425 { 00426 recVal[ recCnt ] = peRec[ t ].fcDet [ i ].gazDetUD ; 00427 recCnt++; 00428 } 00429 } 00430 } 00431 tmpVal = 0; 00432 for( i = 0; i < recCnt ; i++ ) { tmpVal += recVal[ i ] ; } 00433 if ( recCnt == 0 ) { recCnt = 1 ; } 00434 peRes->peFace[k].gaz.UD = tmpVal / recCnt; 00435 } 00436 00437 00438 // expression ------------------------------------------------------------------------------------------------- 00439 if( execFlg->exp == STB_TRUE ) 00440 { 00441 recCnt = 0; 00442 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00443 { 00444 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00445 { 00446 if( 00447 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00448 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00449 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00450 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00451 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00452 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00453 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00454 && peRec[t].fcDet[i].expDetConf != STB_ERR_PE_CANNOT // 00455 ) 00456 { 00457 recVal[ recCnt ] = PeExpressID ( peRec[ t ].fcDet[i].expDetVal ); 00458 recCnt++; 00459 } 00460 } 00461 } 00462 for( i = 0; i < STB_EX_MAX; i++ ) { expVal[ i ] = 0 ; } 00463 for( i = 0; i < recCnt ; i++ ) { expVal[ recVal[ i ] ] += 1 ; } 00464 peRes->peFace[k].exp.value = PeExpressID ( expVal ); 00465 peRes->peFace[k].exp.conf = STB_CONF_NO_DATA;//no Confidence 00466 if ( recCnt == 0 ) { peRes->peFace[k].exp.status = STB_STATUS_NO_DATA ; }//stabilization impossible 00467 else { peRes->peFace[k].exp.status = STB_STATUS_CALCULATING; }//during stabilization 00468 } 00469 00470 // blink L ------------------------------------------------------------------------------------------------- 00471 if( execFlg->bli == STB_TRUE ) 00472 { 00473 recCnt = 0; 00474 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00475 { 00476 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00477 { 00478 if( 00479 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00480 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00481 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00482 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00483 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00484 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00485 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00486 && peRec[t].fcDet[i].bliDetL != STB_ERR_PE_CANNOT // 00487 ) 00488 { 00489 recVal[ recCnt ] = peRec[ t ].fcDet[ i ].bliDetL ; 00490 recCnt++; 00491 } 00492 } 00493 } 00494 if ( recCnt == 0 ) { peRes->peFace[k].bli.status = STB_STATUS_NO_DATA ; }//stabilization impossible 00495 else { peRes->peFace[k].bli.status = STB_STATUS_CALCULATING; }//during stabilization 00496 tmpVal = 0; 00497 for( i = 0; i < recCnt ; i++ ) { tmpVal += recVal[ i ] ; } 00498 if ( recCnt == 0 ) { recCnt = 1 ; } 00499 peRes->peFace[k].bli.ratioL = tmpVal / recCnt; 00500 // blink R ------------------------------------------------------------------------------------------------- 00501 recCnt = 0; 00502 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00503 { 00504 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00505 { 00506 if( 00507 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00508 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00509 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00510 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00511 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00512 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00513 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00514 && peRec[t].fcDet[i].bliDetR != STB_ERR_PE_CANNOT // 00515 ) 00516 { 00517 recVal[ recCnt ] = peRec[ t ].fcDet [ i ].bliDetR; 00518 recCnt++; 00519 } 00520 } 00521 } 00522 tmpVal = 0; 00523 for( i = 0; i < recCnt ; i++ ) { tmpVal += recVal[ i ] ; } 00524 if ( recCnt == 0 ) { recCnt = 1 ; } 00525 peRes->peFace[k].bli.ratioR = tmpVal / recCnt; 00526 } 00527 00528 00529 00530 // dirYaw ------------------------------------------------------------------------------------------------- 00531 if( execFlg->dir == STB_TRUE ) 00532 { 00533 recCnt = 0; 00534 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00535 { 00536 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00537 { 00538 if( 00539 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00540 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00541 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00542 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00543 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00544 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00545 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00546 ) 00547 { 00548 recVal[ recCnt ] = peRec[ t ].fcDet [ i ].dirDetYaw ; 00549 recCnt++; 00550 } 00551 } 00552 } 00553 if ( recCnt == 0 ) { peRes->peFace[k].dir.status = STB_STATUS_NO_DATA ; }//stabilization impossible 00554 else { peRes->peFace[k].dir.status = STB_STATUS_CALCULATING; }//during stabilization 00555 peRes->peFace[k].dir.conf = STB_CONF_NO_DATA;//no Confidence 00556 tmpVal = 0; 00557 for( i = 0; i < recCnt ; i++ ) { tmpVal += recVal[ i ] ; } 00558 if ( recCnt == 0 ) { recCnt = 1 ; } 00559 peRes->peFace[k].dir.yaw = tmpVal / recCnt; 00560 // dirRoll ------------------------------------------------------------------------------------------------- 00561 recCnt = 0; 00562 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00563 { 00564 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00565 { 00566 if( 00567 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00568 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00569 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00570 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00571 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00572 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00573 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00574 ) 00575 { 00576 recVal[ recCnt ] = peRec[ t ].fcDet [ i ].dirDetRoll; 00577 recCnt++; 00578 } 00579 } 00580 } 00581 tmpVal = 0; 00582 for( i = 0; i < recCnt ; i++ ) { tmpVal += recVal[ i ] ; } 00583 if ( recCnt == 0 ) { recCnt = 1 ; } 00584 peRes->peFace[k].dir.roll = tmpVal / recCnt; 00585 // dirPitch ------------------------------------------------------------------------------------------------- 00586 recCnt = 0; 00587 for( t = 0; t < STB_PE_BACK_MAX ; t++) 00588 { 00589 for( i = 0; i < peRec[t].num ; i++) //a number of tracking people(previous t frame) 00590 { 00591 if( 00592 peRec[t].fcDet[i].nTraID == trID //the same tracking number 00593 && peRec[t].fcDet[i].nDetID >= 0 //not lost 00594 && peRec[t].fcDet[i].dirDetConf >= dirThr // Face angle : confidence 00595 && peRec[t].fcDet[i].dirDetPitch >= dirUDMin // Face angle : pitch 00596 && peRec[t].fcDet[i].dirDetPitch <= dirUDMax // Face angle : pitch 00597 && peRec[t].fcDet[i].dirDetYaw >= dirLRMin // Face angle : yaw 00598 && peRec[t].fcDet[i].dirDetYaw <= dirLRMax // Face angle : yaw 00599 ) 00600 { 00601 recVal[ recCnt ] = peRec[ t ].fcDet [ i ].dirDetPitch ; 00602 recCnt++; 00603 } 00604 } 00605 } 00606 tmpVal = 0; 00607 for( i = 0; i < recCnt ; i++ ) { tmpVal += recVal[ i ] ; } 00608 if ( recCnt == 0 ) { recCnt = 1 ; } 00609 peRes->peFace[k].dir.pitch = tmpVal / recCnt; 00610 } 00611 00612 }//for( k = 0; k < peRes->peCnt ; k++) 00613 00614 } 00615 /*---------------------------------------------------------------------*/ 00616 // StbPeExec 00617 /*---------------------------------------------------------------------*/ 00618 int StbPeExec ( PEHANDLE handle ) 00619 { 00620 00621 int retVal = 0 ; 00622 00623 /* Face --------------------------------------*/ 00624 PeSlideFacesRec ( handle->peDetRec , 00625 handle->execFlg );//Shift the time series of past data before stabilization. 00626 PeCurFaces ( handle->peDetRec , 00627 &(handle->peDet) , 00628 handle->execFlg );//Setting "present data before the stabilization" to past data before the stabilization. 00629 00630 PeStbFaceEasy ( &(handle->peRes) , 00631 handle->peDetRec , 00632 handle->peFaceDirThr , 00633 handle->peFaceDirUDMax , 00634 handle->peFaceDirUDMin , 00635 handle->peFaceDirLRMax , 00636 handle->peFaceDirLRMin , 00637 handle->peFrameCount , 00638 handle->execFlg );//Calculate "current data after stabilization" from "past data before stabilization". 00639 00640 00641 00642 00643 return retVal; 00644 } 00645 00646 00647
Generated on Fri Jul 15 2022 01:15:47 by
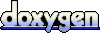