
NerfUS game coordinator for the Nerf gun firing range
Dependencies: HardwareInterface mbed-rtos mbed
Fork of NerfUS by
GoogleMockTest.cpp
00001 #include "gmock/gmock.h" 00002 #include "gtest/gtest.h" 00003 00004 class Adder 00005 { 00006 public: 00007 virtual ~Adder() {} 00008 virtual int add(int a, int b) 00009 { 00010 return a + b; 00011 } 00012 }; 00013 00014 class MockAdder : public Adder 00015 { 00016 public: 00017 MOCK_METHOD2(add, int(int a, int b)); 00018 }; 00019 00020 TEST(GoogleMockTest, ExpectCalled) 00021 { 00022 MockAdder adder; 00023 00024 EXPECT_CALL(adder, add(1, 2)); 00025 00026 adder.add(1, 2); 00027 } 00028 00029 TEST(GoogleMockTest, DefineReturnedValue) 00030 { 00031 using ::testing::Return; 00032 MockAdder adder; 00033 00034 EXPECT_CALL(adder, add(1, 2)) 00035 .WillOnce(Return(42)); 00036 00037 ASSERT_EQ(42, adder.add(1, 2)); 00038 }
Generated on Thu Jul 14 2022 07:10:37 by
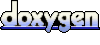