
MCU Code for FRDM-K64F which works with Graphical User Interface available on Freescale website to control a brushed DC motor using X-FRDM-34931SEVM. The code enables controlling the PWM frequency, duty cycle, enabling/disabling while monitoring status flag pin for undervoltge, short circuit and over-temperature events as well as real time load current for implementing advanced diagnostics.
Fork of Brushed DC Motor Control using X-FRDM-34931SEVM and FRDM-K64F by
main.cpp
00001 #include "mbed.h" 00002 #include "USBHID.h" 00003 00004 // We declare a USBHID device. 00005 // HID In/Out Reports are 64 Bytes long 00006 // Vendor ID (VID): 0x15A2 00007 // Product ID (PID): 0x0138 00008 // Serial Number: 0x0001 00009 USBHID hid(64, 64, 0x15A2, 0x0138, 0x0001, true); 00010 00011 //Setup Digital Outputs for the LEDs on the FRDM 00012 //PwmOut red_led(LED1); 00013 //DigitalOut green_led(LED2); 00014 //DigitalOut blue_led(LED3); 00015 00016 //Setup PWM and Digital Outputs from FRDM-K64F 00017 PwmOut IN1(PTC2); // Pin IN1 input to MC34931 (FRDM PIN Name) 00018 PwmOut IN2 (PTA2); // Pin IN2 input to MC34931 (FRDM PIN Name) 00019 DigitalOut EN(PTE25); // Pin EN input to MC34931 (FRDM PIN Name) 00020 DigitalOut DIS(PTC17); // Pin D1 input to MC34931 (FRDM PIN Name) 00021 DigitalIn STATUS(PTB11); 00022 AnalogIn CFB(PTB2); 00023 //DigitalOut READY(PTC7); // Pin READY input to Motor Control Board (FRDM PIN Name) 00024 00025 //Variables 00026 int pwm_freq_lo; 00027 int pwm_freq_hi; 00028 int frequencyHz = 500; 00029 int runstop = 0; 00030 int direction = 1; 00031 int braking; 00032 int dutycycle = 75; 00033 int newDataFlag = 0; 00034 int status = 0; 00035 uint16_t CurrFB; 00036 uint16_t CFBArray[101]; 00037 uint32_t CFBTotal; 00038 uint16_t CFBAvg; 00039 uint16_t CFBtemp; 00040 00041 //storage for send and receive data 00042 HID_REPORT send_report; 00043 HID_REPORT recv_report; 00044 00045 bool initflag = true; 00046 00047 // USB COMMANDS 00048 // These are sent from the PC 00049 #define WRITE_LED 0x20 00050 #define WRITE_GEN_EN 0x40 00051 #define WRITE_DUTY_CYCLE 0x50 00052 #define WRITE_PWM_FREQ 0x60 00053 #define WRITE_RUN_STOP 0x70 00054 #define WRITE_DIRECTION 0x71 00055 #define WRITE_BRAKING 0x90 00056 #define WRITE_RESET 0xA0 00057 #define WRITE_D1 0xB1 00058 #define WRITE_D2_B 0xC1 00059 00060 #define scaleFactor 0.11868 00061 00062 // LOGICAL CONSTANTS 00063 #define OFF 0x00 00064 #define ON 0x01 00065 00066 00067 int main() 00068 { 00069 send_report.length = 64; 00070 recv_report.length = 64; 00071 00072 00073 while(1) 00074 { 00075 //try to read a msg 00076 if(hid.readNB(&recv_report)) 00077 { 00078 switch(recv_report.data[0]) //byte 0 of recv_report.data is command 00079 { 00080 //----------------------------------------------------------------------------------------------------------------- 00081 // COMMAND PARSER 00082 //----------------------------------------------------------------------------------------------------------------- 00083 //////// 00084 case WRITE_LED: 00085 break; 00086 //////// 00087 00088 //////// 00089 case WRITE_DUTY_CYCLE: 00090 dutycycle = recv_report.data[1]; 00091 newDataFlag = 1; 00092 break; 00093 //////// 00094 case WRITE_PWM_FREQ: //PWM frequency can be larger than 1 byte 00095 pwm_freq_lo = recv_report.data[1]; //so we have to re-assemble the number 00096 pwm_freq_hi = recv_report.data[2] * 100; 00097 frequencyHz = pwm_freq_lo + pwm_freq_hi; 00098 newDataFlag = 1; 00099 break; 00100 //////// 00101 case WRITE_RUN_STOP: 00102 newDataFlag = 1; 00103 if(recv_report.data[1] != 0) 00104 { 00105 runstop = 1; 00106 } 00107 else 00108 { 00109 runstop = 0; 00110 } 00111 break; 00112 00113 //////// 00114 case WRITE_DIRECTION: 00115 newDataFlag = 1; 00116 00117 if(recv_report.data[1] != 0) 00118 { 00119 direction = 1; 00120 } 00121 else 00122 { 00123 direction = 0; 00124 } 00125 break; 00126 //////// 00127 case WRITE_BRAKING: 00128 newDataFlag = 1; 00129 if(recv_report.data[1] == 1) 00130 { 00131 braking = 1; 00132 } 00133 else 00134 { 00135 braking = 0; 00136 } 00137 break; 00138 //////// 00139 case WRITE_D1: 00140 newDataFlag = 1; 00141 if(recv_report.data[1] == 1) 00142 { 00143 DIS = 1; 00144 } 00145 else 00146 { 00147 DIS = 0; 00148 } 00149 break; 00150 //////// 00151 case WRITE_D2_B: 00152 newDataFlag = 1; 00153 if(recv_report.data[1] == 1) 00154 { 00155 EN = 0; 00156 } 00157 else 00158 { 00159 EN = 1; 00160 } 00161 break; 00162 00163 //////// 00164 default: 00165 break; 00166 }// End Switch recv report data[0] 00167 00168 //----------------------------------------------------------------------------------------------------------------- 00169 // end command parser 00170 //----------------------------------------------------------------------------------------------------------------- 00171 00172 status = STATUS; 00173 send_report.data[0] = status; // Echo Command 00174 send_report.data[1] = recv_report.data[1]; // Echo Subcommand 1 00175 send_report.data[2] = recv_report.data[2]; // Echo Subcommand 2 00176 send_report.data[3] = 0x00; 00177 send_report.data[4] = 0x00; 00178 send_report.data[5] = 0x00; 00179 send_report.data[6] = (CFBAvg << 8) >> 8; 00180 send_report.data[7] = CFBAvg >> 8; 00181 00182 //Send the report 00183 hid.send(&send_report); 00184 }// End If(hid.readNB(&recv_report)) 00185 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00186 //End of USB message handling 00187 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00188 00189 CurrFB = CFB.read_u16(); 00190 CFBArray[0] = CurrFB; 00191 CFBTotal = 0; 00192 int i = 0; 00193 for(i=0; i<100; i++) 00194 { 00195 CFBTotal = CFBTotal + CFBArray[i]; 00196 } 00197 CFBAvg = CFBTotal / 100; 00198 00199 for(i=100; i>=0; i--) 00200 { 00201 00202 CFBArray[i+1] = CFBArray[i]; 00203 } 00204 00205 if(newDataFlag != 0) //GUI setting changed 00206 { 00207 newDataFlag = 0; 00208 if(runstop != 0) //Running 00209 { 00210 if(direction == 0) //reverse 00211 { 00212 if(braking == 1) //dynamic 00213 { 00214 IN1.period(1/(float)frequencyHz); 00215 IN1 = (float)dutycycle/100.0; 00216 IN2 = 1; 00217 } 00218 else //coast 00219 { 00220 IN1 = 0; 00221 IN2.period(1/(float)frequencyHz); 00222 IN2 = (float)dutycycle/100.0; 00223 } 00224 } 00225 else //forward 00226 { 00227 if(braking == 1) //dynamic 00228 { 00229 IN1 = 1; 00230 IN2.period(1/(float)frequencyHz); 00231 IN2 = (float)dutycycle/100.0; 00232 } 00233 else //coast 00234 { 00235 IN1.period(1/(float)frequencyHz); 00236 IN1 = (float)dutycycle/100.0; 00237 IN2 = 0; 00238 } 00239 } 00240 } 00241 else //Stopped 00242 { 00243 if(braking == 1) //braking 00244 { 00245 IN1.period(1); 00246 IN2.period(1); 00247 IN1.write(1); 00248 IN2.write(1); 00249 } 00250 else //coasting 00251 { 00252 IN1.period(1); 00253 IN2.period(1); 00254 IN1.write(0); 00255 IN2.write(0); 00256 00257 } 00258 } 00259 } 00260 } 00261 } 00262
Generated on Thu Jul 14 2022 05:18:48 by
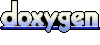