1-Wire® library for mbed. Complete 1-Wire library that supports our silicon masters along with a bit-bang master on the MAX32600MBED platform with one common interface for mbed. Slave support has also been included and more slaves will be added as time permits.
Dependents: MAXREFDES131_Qt_Demo MAX32630FTHR_iButton_uSD_Logger MAX32630FTHR_DS18B20_uSD_Logger MAXREFDES130_131_Demo ... more
DS2431.h
00001 /******************************************************************//** 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 **********************************************************************/ 00032 00033 #ifndef OneWire_Slaves_Memory_DS2431 00034 #define OneWire_Slaves_Memory_DS2431 00035 00036 #include "Slaves/OneWireSlave.h" 00037 00038 namespace OneWire 00039 { 00040 /** 00041 * @brief DS2431 1024-Bit 1-Wire EEPROM 00042 * @details The DS2431 is a 1024-bit, 1-Wire® EEPROM chip organized 00043 * as four memory pages of 256 bits each. Data is written to an 8-byte 00044 * scratchpad, verified, and then copied to the EEPROM memory. As a 00045 * special feature, the four memory pages can individually be write 00046 * protected or put in EPROM-emulation mode, where bits can only be 00047 * changed from a 1 to a 0 state. The DS2431 communicates over the 00048 * single-conductor 1-Wire bus. The communication follows the standard 00049 * 1-Wire protocol. Each device has its own unalterable and unique 00050 * 64-bit ROM registration number that is factory lasered into the chip. 00051 * The registration number is used to address the device in a multidrop, 00052 * 1-Wire net environment. 00053 */ 00054 class DS2431 : public OneWireSlave 00055 { 00056 public: 00057 typedef array<uint8_t, 8> Scratchpad ; 00058 typedef uint16_t Address; 00059 00060 /**********************************************************//** 00061 * @brief DS2431 constructor 00062 * 00063 * @details Instantiate a DS2431 object that encapsulates the 00064 * 1-Wire master and ROM commands for selecting the device via 00065 * the RandomAccessRomIterator sub-class passed as an argument 00066 * to the constructor. 00067 * 00068 * This allows the user to focus on the use of the DS2431 in 00069 * their application vs. the low level details of the 1-Wire 00070 * protocol. 00071 * 00072 * On Entry: 00073 * @param[in] selector - reference to RandomAccessRomIterator 00074 * sub-class; i.e. SingledropRomIterator, MultidropRomIterator, etc. 00075 * See RomId/RomIterator.h 00076 * 00077 * On Exit: 00078 * 00079 * @return 00080 **************************************************************/ 00081 DS2431(RandomAccessRomIterator & selector); 00082 00083 /**********************************************************//** 00084 * @brief writeMemory 00085 * 00086 * @details Writes data to EEPROM. Wraps up writeScratchPad, 00087 * readScratchPad and copyScratchPad into single function. 00088 * 00089 * On Entry: 00090 * @param[in] targetAddress - EEPROM memory address to start 00091 * writing at. 00092 * 00093 * @param[in] data - Pointer to memory holding data. 00094 * 00095 * @param[in] numBytes - Number of bytes to write. 00096 * 00097 * On Exit: 00098 * 00099 * @return Result of operation 00100 **************************************************************/ 00101 OneWireSlave::CmdResult writeMemory(Address targetAddress, const Scratchpad & data); 00102 00103 /**********************************************************//** 00104 * @brief readMemory 00105 * 00106 * @details Reads block of data from EEPROM memory. 00107 * 00108 * On Entry: 00109 * @param[in] targetAddress - EEPROM memory address to start. 00110 * reading from 00111 * 00112 * @param[out] data - Pointer to memory for storing data. 00113 * 00114 * @param[in] numBytes - Number of bytes to read. 00115 * 00116 * On Exit: 00117 * 00118 * @return Result of operation 00119 **************************************************************/ 00120 OneWireSlave::CmdResult readMemory(Address targetAddress, uint8_t numBytes, uint8_t * data); 00121 00122 private: 00123 /**********************************************************//** 00124 * @brief writeScratchpad 00125 * 00126 * @details Writes 8 bytes to the scratchpad. 00127 * 00128 * On Entry: 00129 * @param[in] targetAddress - EEPROM memory address that this data 00130 * will be copied to. Must be on row boundary. 00131 * 00132 * @param[in] data - reference to bounded array type Scratchpad. 00133 * 00134 * On Exit: 00135 * 00136 * @return Result of operation 00137 **************************************************************/ 00138 OneWireSlave::CmdResult writeScratchpad(Address targetAddress, const Scratchpad & data); 00139 00140 /**********************************************************//** 00141 * @brief readScratchpad 00142 * 00143 * @details Reads contents of scratchpad. 00144 * 00145 * On Entry: 00146 * @param[out] data - reference to bounded array type Scratchpad. 00147 * 00148 * On Exit: 00149 * 00150 * @return Result of operation 00151 **************************************************************/ 00152 OneWireSlave::CmdResult readScratchpad(Scratchpad & data, uint8_t & esByte); 00153 00154 /**********************************************************//** 00155 * @brief copyScratchpad 00156 * 00157 * @details Copies contents of sractshpad to EEPROM. 00158 * 00159 * On Entry: 00160 * @param[in] targetAddress - EEPROM memory address that this data 00161 * will be copied to. Must be on row boundary. 00162 * 00163 * @param[in] esByte - Returned from reading scratchpad. 00164 * 00165 * On Exit: 00166 * 00167 * @return Result of operation 00168 **************************************************************/ 00169 OneWireSlave::CmdResult copyScratchpad(Address targetAddress, uint8_t esByte); 00170 }; 00171 } 00172 00173 #endif /*OneWire_Slaves_Memory_DS2431*/
Generated on Tue Jul 12 2022 15:46:20 by
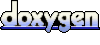