No more update~~ please use W5500Interface.
Fork of EthernetInterfaceW5500 by
EthernetInterfaceW5500.cpp
00001 // EthernetInterfaceW5500.cpp 2014/7/17 00002 00003 #include "EthernetInterfaceW5500.h" 00004 #include "DHCPClient.h" 00005 00006 EthernetInterfaceW5500::EthernetInterfaceW5500(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset) : 00007 WIZnet_Chip(mosi, miso, sclk, cs, reset) 00008 { 00009 ip_set = false; 00010 } 00011 00012 EthernetInterfaceW5500::EthernetInterfaceW5500(SPI* spi, PinName cs, PinName reset) : 00013 WIZnet_Chip(spi, cs, reset) 00014 { 00015 ip_set = false; 00016 } 00017 00018 int EthernetInterfaceW5500::init() 00019 { 00020 dhcp = true; 00021 // 00022 //for (int i =0; i < 6; i++) this->mac[i] = mac[i]; 00023 // 00024 reset(); 00025 return 0; 00026 } 00027 00028 int EthernetInterfaceW5500::init(uint8_t * mac) 00029 { 00030 dhcp = true; 00031 // 00032 for (int i =0; i < 6; i++) this->mac[i] = mac[i]; 00033 // 00034 reset(); 00035 setmac(); 00036 return 0; 00037 } 00038 00039 // add this function, because sometimes no needed MAC address in init calling. 00040 int EthernetInterfaceW5500::init(const char* ip, const char* mask, const char* gateway) 00041 { 00042 dhcp = false; 00043 // 00044 //for (int i =0; i < 6; i++) this->mac[i] = mac[i]; 00045 // 00046 this->ip = str_to_ip(ip); 00047 strcpy(ip_string, ip); 00048 ip_set = true; 00049 this->netmask = str_to_ip(mask); 00050 this->gateway = str_to_ip(gateway); 00051 reset(); 00052 00053 // @Jul. 8. 2014 add code. should be called to write chip. 00054 setip(); 00055 00056 return 0; 00057 } 00058 00059 int EthernetInterfaceW5500::init(uint8_t * mac, const char* ip, const char* mask, const char* gateway) 00060 { 00061 dhcp = false; 00062 // 00063 for (int i =0; i < 6; i++) this->mac[i] = mac[i]; 00064 // 00065 this->ip = str_to_ip(ip); 00066 strcpy(ip_string, ip); 00067 ip_set = true; 00068 this->netmask = str_to_ip(mask); 00069 this->gateway = str_to_ip(gateway); 00070 reset(); 00071 00072 // @Jul. 8. 2014 add code. should be called to write chip. 00073 setmac(); 00074 setip(); 00075 00076 return 0; 00077 } 00078 00079 // Connect Bring the interface up, start DHCP if needed. 00080 int EthernetInterfaceW5500::connect() 00081 { 00082 if (dhcp) { 00083 int r = IPrenew(); 00084 if (r < 0) { 00085 return r; 00086 } 00087 } 00088 00089 if (WIZnet_Chip::setip() == false) return -1; 00090 return 0; 00091 } 00092 00093 // Disconnect Bring the interface down. 00094 int EthernetInterfaceW5500::disconnect() 00095 { 00096 if (WIZnet_Chip::disconnect() == false) return -1; 00097 return 0; 00098 } 00099 00100 char* EthernetInterfaceW5500::getIPAddress() 00101 { 00102 uint32_t ip = reg_rd<uint32_t>(SIPR); 00103 snprintf(ip_string, sizeof(ip_string), "%d.%d.%d.%d", (ip>>24)&0xff, (ip>>16)&0xff, (ip>>8)&0xff, ip&0xff); 00104 return ip_string; 00105 } 00106 00107 char* EthernetInterfaceW5500::getNetworkMask() 00108 { 00109 uint32_t ip = reg_rd<uint32_t>(SUBR); 00110 snprintf(mask_string, sizeof(mask_string), "%d.%d.%d.%d", (ip>>24)&0xff, (ip>>16)&0xff, (ip>>8)&0xff, ip&0xff); 00111 return mask_string; 00112 } 00113 00114 char* EthernetInterfaceW5500::getGateway() 00115 { 00116 uint32_t ip = reg_rd<uint32_t>(GAR); 00117 snprintf(gw_string, sizeof(gw_string), "%d.%d.%d.%d", (ip>>24)&0xff, (ip>>16)&0xff, (ip>>8)&0xff, ip&0xff); 00118 return gw_string; 00119 } 00120 00121 char* EthernetInterfaceW5500::getMACAddress() 00122 { 00123 uint8_t mac[6]; 00124 reg_rd_mac(SHAR, mac); 00125 snprintf(mac_string, sizeof(mac_string), "%02X:%02X:%02X:%02X:%02X:%02X", mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]); 00126 return mac_string; 00127 } 00128 00129 int EthernetInterfaceW5500::IPrenew(int timeout_ms) 00130 { 00131 // printf("DHCP Started, waiting for IP...\n"); 00132 DHCPClient dhcp; 00133 int err = dhcp.setup(timeout_ms); 00134 if (err == (-1)) { 00135 // printf("Timeout.\n"); 00136 return -1; 00137 } 00138 // printf("Connected, IP: %d.%d.%d.%d\n", dhcp.yiaddr[0], dhcp.yiaddr[1], dhcp.yiaddr[2], dhcp.yiaddr[3]); 00139 ip = (dhcp.yiaddr[0] <<24) | (dhcp.yiaddr[1] <<16) | (dhcp.yiaddr[2] <<8) | dhcp.yiaddr[3]; 00140 gateway = (dhcp.gateway[0]<<24) | (dhcp.gateway[1]<<16) | (dhcp.gateway[2]<<8) | dhcp.gateway[3]; 00141 netmask = (dhcp.netmask[0]<<24) | (dhcp.netmask[1]<<16) | (dhcp.netmask[2]<<8) | dhcp.netmask[3]; 00142 dnsaddr = (dhcp.dnsaddr[0]<<24) | (dhcp.dnsaddr[1]<<16) | (dhcp.dnsaddr[2]<<8) | dhcp.dnsaddr[3]; 00143 return 0; 00144 }
Generated on Wed Jul 13 2022 01:50:02 by
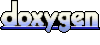