Demo the function of RTC module AM1805
Dependencies: mbed-dev
Fork of I2C_HelloWorld_Mbed by
AM1805.h
00001 /* AM1805.h , AM1805 Sample code: external RTC module is used by host MCU */ 00002 00003 typedef struct 00004 { 00005 uint8_t hundredth; 00006 uint8_t second; 00007 uint8_t minute; 00008 uint8_t hour; 00009 uint8_t date; 00010 uint8_t weekday; 00011 uint8_t month; 00012 uint8_t year; 00013 uint8_t century; 00014 uint8_t mode; 00015 } time_reg_struct_t; 00016 00017 typedef enum 00018 { 00019 XT1_INTERRUPT = 0x01, /**< WDI input pin will generate XT1 interrupt */ 00020 XT2_INTERRUPT = 0x02 /**< EXTI input pin will generate XT2 interrupt */ 00021 } input_interrupt_t; 00022 00023 typedef enum 00024 { 00025 DISABLE_ALARM = 0, /**< disable alarm */ 00026 ONCE_PER_YEAR = 1, /**< once per year */ 00027 ONCE_PER_MONTH = 2, /**< once per month */ 00028 ONCE_PER_WEEK = 3, /**< once per week */ 00029 ONCE_PER_DAY = 4, /**< once per day */ 00030 ONCE_PER_HOUR = 5, /**< once per hour */ 00031 ONCE_PER_MINUTE = 6, /**< once per minute */ 00032 ONCE_PER_SECOND = 7, /**< once per second */ 00033 ONCE_PER_10TH_SEC = 8, /**< once per 10th of a second */ 00034 ONCE_PER_100TH_SEC = 9 /**< once per 100th of a second */ 00035 } alarm_repeat_t; 00036 00037 typedef enum 00038 { 00039 PERIOD_US = 0, /**< period in us */ 00040 PERIOD_SEC = 1 /**< period in seconds */ 00041 } count_down_range_t; 00042 00043 typedef enum 00044 { 00045 SINGLE_LEVEL_INTERRUPT = 0, /**< single level interrupt */ 00046 REPEAT_PULSE_1_4096_SEC = 1, /**< a repeated pulsed interrupt, 1/4096 s (XT mode), 1/128 s (RC mode) (range must be 0) */ 00047 SINGLE_PULSE_1_4096_SEC = 2, /**< a single pulsed interrupt, 1/4096 s (XT mode), 1/128 s (RC mode) (range must be 0) */ 00048 REPEAT_PLUSE_1_128_SEC = 3, /**< a repeated pulsed interrupt, 1/128 s (range must be 0) */ 00049 SINGLE_PLUSE_1_128_SEC = 4, /**< a single pulsed interrupt, 1/128 s (range must be 0) */ 00050 REPEAT_PLUSE_1_64_SEC = 5, /**< a repeated pulsed interrupt, 1/64 s (range must be 1) */ 00051 SINGLE_PLUSE_1_64_SEC = 6 /**< a single pulsed interrupt, 1/64 s (range must be 1) */ 00052 } count_down_repeat_t; 00053 00054 typedef enum 00055 { 00056 LEVEL_INTERRUPT = 0x00, /**< level interrupt */ 00057 PULSE_1_8192_SEC = 0x01, /**< pulse of 1/8192s (XT) or 1/128 s (RC) */ 00058 PULSE_1_64_SEC = 0x10, /**< pulse of 1/64 s */ 00059 PULSE_1_4_SEC = 0x11 /**< pulse of 1/4 s */ 00060 } interrupt_mode_t; 00061 00062 typedef enum 00063 { 00064 INTERNAL_FLAG = 0, /**< internal flag only */ 00065 PIN_FOUT_nIRQ = 1, /**< generate the interrupt on FOUT/nIRQ */ 00066 PIN_PSW_nIRQ2 = 2, /**< generate the interrupt on PSW/nIRQ2 */ 00067 PIN_nTIRQ = 3 /**< generate the interrupt on nTIRQ (not apply to ALARM) */ 00068 } interrupt_pin_t; 00069 00070 bool am1805_init(void); 00071 00072 void am1805_register_read(char register_address, char *destination, uint8_t number_of_bytes); 00073 00074 void am1805_register_write(char register_address, uint8_t value); 00075 00076 void am1805_burst_write(uint8_t *value, uint8_t number_of_bytes); 00077 00078 uint8_t am1805_read_ram(uint8_t address); 00079 00080 void am1805_write_ram(uint8_t address, uint8_t data); 00081 00082 void am1805_config_input_interrupt(input_interrupt_t index_Interrupt); 00083 00084 void am1805_set_time(time_reg_struct_t time_regs); 00085 00086 void am1805_get_time(time_reg_struct_t *time_regs); 00087 00088 void am1805_config_alarm(time_reg_struct_t time_regs, alarm_repeat_t repeat, interrupt_mode_t intmode, interrupt_pin_t pin); 00089 00090 void am1805_config_countdown_timer(count_down_range_t range, int32_t period, count_down_repeat_t repeat, interrupt_pin_t pin); 00091 00092 void am1805_set_sleep(uint8_t timeout, uint8_t mode);
Generated on Wed Jul 13 2022 11:36:42 by
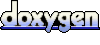