
Transistor Gijutsu, October 2014, Special Features Chapter 7,Software of the LCRmeter トランジスタ技術2014年10月号 特集第7章のソフトウェア,サバイバルLCRメータ
Dependencies: mbed
lcd_P4bit.cpp
00001 // 00002 // HD44780 type 4bit parallel LCD control 00003 // 00004 #include "mbed.h" 00005 00006 DigitalOut LCDRS(P0_3); // LCD module Register Select 00007 DigitalOut LCDE(P0_1); // LCD module Enable 00008 DigitalOut LCDD4(P1_19); // LCD data lines(4bit) 00009 DigitalOut LCDD5(P0_5); 00010 DigitalOut LCDD6(P0_4); 00011 DigitalOut LCDD7(P1_15); 00012 00013 void enable( void ) 00014 { 00015 wait_us( 1 ); 00016 LCDE = 1; 00017 wait_us( 1 ); 00018 LCDE = 0; 00019 wait_us( 1 ); 00020 } 00021 00022 void lcd_set4bit( unsigned char d ) 00023 { 00024 LCDD4 = d & 0x01; 00025 LCDD5 = d & 0x02; 00026 LCDD6 = d & 0x04; 00027 LCDD7 = d & 0x08; 00028 } 00029 00030 void lcd_write4bit( unsigned char d ) 00031 { 00032 lcd_set4bit( d ); 00033 enable(); 00034 } 00035 00036 void LCD_cmd( char c ) 00037 { 00038 LCDRS = 0; 00039 lcd_write4bit( c / 16 ); 00040 lcd_write4bit( c & 0x0f ); 00041 wait_us( 30 ); 00042 } 00043 00044 void LCD_data( char d ) 00045 { 00046 LCDRS = 1; 00047 lcd_write4bit( d / 16 ); 00048 lcd_write4bit( d & 0x0f ); 00049 wait_us( 30 ); 00050 } 00051 00052 void LCD_puts( char *s ) 00053 { 00054 while( *s ) LCD_data( *s++ ); 00055 } 00056 00057 void LCD_iniz( void ) 00058 { 00059 LCDE = 0; 00060 LCDRS = 0; 00061 wait_ms( 40 ); // wait 40mS 00062 lcd_write4bit( 0x03 ); 00063 wait_ms( 5 ); 00064 lcd_write4bit( 0x03 ); 00065 wait_ms( 2 ); 00066 lcd_write4bit( 0x03 ); 00067 wait_ms( 2 ); 00068 lcd_write4bit( 0x02 ); // 4bit interface select 00069 LCD_cmd( 0x28 ); // 2 lines 5x8 chr. 00070 LCD_cmd( 0x08 ); // display off 00071 LCD_cmd( 0x01 ); // display clear 00072 wait_ms( 2 ); 00073 LCD_cmd( 0x06 ); // entry mode 00074 LCD_cmd( 0x0C ); // display on 00075 } 00076
Generated on Mon Jul 18 2022 17:55:24 by
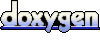