A collection of Analog Devices drivers for the mbed platform
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * @file main.cpp 00003 * @brief Main file of the driver diag tool 00004 * @author Analog Devices Inc. 00005 * 00006 * For support please go to: 00007 * Github: https://github.com/analogdevicesinc/mbed-adi 00008 * Support: https://ez.analog.com/community/linux-device-drivers/microcontroller-no-os-drivers 00009 * More: https://wiki.analog.com/resources/tools-software/mbed-drivers-all 00010 00011 ******************************************************************************** 00012 * Copyright 2016(c) Analog Devices, Inc. 00013 * 00014 * All rights reserved. 00015 * 00016 * Redistribution and use in source and binary forms, with or without 00017 * modification, are permitted provided that the following conditions are met: 00018 * - Redistributions of source code must retain the above copyright 00019 * notice, this list of conditions and the following disclaimer. 00020 * - Redistributions in binary form must reproduce the above copyright 00021 * notice, this list of conditions and the following disclaimer in 00022 * the documentation and/or other materials provided with the 00023 * distribution. 00024 * - Neither the name of Analog Devices, Inc. nor the names of its 00025 * contributors may be used to endorse or promote products derived 00026 * from this software without specific prior written permission. 00027 * - The use of this software may or may not infringe the patent rights 00028 * of one or more patent holders. This license does not release you 00029 * from the requirement that you obtain separate licenses from these 00030 * patent holders to use this software. 00031 * - Use of the software either in source or binary form, must be run 00032 * on or directly connected to an Analog Devices Inc. component. 00033 * 00034 * THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00035 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00036 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00037 * IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00038 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00039 * LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00040 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00041 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00042 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00043 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00044 * 00045 ********************************************************************************/ 00046 00047 #include "mbed.h" 00048 #include "stdio.h" 00049 #include <iostream> 00050 #include <string> 00051 #include <vector> 00052 00053 #include "config.h" 00054 00055 Serial pc(SERIAL_TX, SERIAL_RX); 00056 Serial test(D8, D2); 00057 vector<string> cmdbuffer; 00058 00059 class commands 00060 { 00061 public: 00062 commands(string str, int p, void (*h)() ) : cmd_str(str), nr_of_param(p), fktPtr(h) { } 00063 const static int VAR = -1; // variable number of params 00064 string cmd_str; 00065 int nr_of_param; 00066 void (*fktPtr)(void); 00067 }; 00068 00069 00070 // *INDENT-OFF* 00071 const vector<commands> cmdlist = { 00072 {"nop" , 0, [](){ }}, 00073 /* 00074 {"echo",commands::VAR, [](){ for(auto i = begin(cmdbuffer) + 1, e = end(cmdbuffer); i!=e; ++i) 00075 printf("%s ", i->c_str()); printf("\r\n");}},*/ 00076 00077 {"help", 0 , [](){ for(auto command : cmdlist) 00078 pc.printf("%s ",command.cmd_str.c_str()); }}, 00079 /* #### SPI ####*/ 00080 #ifdef SPI_LOW_LEVEL 00081 {"csa", 0, [](){ spibus.format(8, 3);CSA_pin = !CSA_pin; wait_us(2); 00082 pc.printf("CS ADC pin set %s", ((CSA_pin.read()) ? "high" : "low") ); }}, 00083 {"csr", 0, [](){ spibus.format(8, 1);CSR_pin = !CSR_pin; wait_us(2); 00084 pc.printf("CS RDAC pin set %s", ((CSR_pin.read()) ? "high" : "low") );} }, 00085 {"spi", 1, [](){ uint8_t spibyte = strtol(cmdbuffer[1].c_str(), NULL, 16); 00086 pc.printf("writing 0x%x to SPI", spibyte); 00087 pc.printf("\r\nreturned: 0x%x ", spibus.write(spibyte)); }}, 00088 #endif 00089 00090 #ifdef AD7791_PRESENT 00091 {"adrst", 0, [](){ad7791diag.reset();}}, 00092 {"adwrm", 1, [](){ad7791diag.write_mode();}}, 00093 {"adrdm", 0, [](){ad7791diag.read_mode();}}, 00094 {"adwrf", 1, [](){ad7791diag.write_filter();}}, 00095 {"adrdf", 0, [](){ad7791diag.read_filter();}}, 00096 {"adrdd", 0, [](){ad7791diag.read_data();}}, 00097 {"adrds", 0, [](){ad7791diag.read_status();}}, 00098 {"adread32", 0, [](){ad7791diag.read();}}, 00099 {"adread", 0, [](){ad7791diag.read_u16();}}, 00100 {"adreadv", 0, [](){ad7791diag.read_voltage();}}, 00101 {"adsetc", 1, [](){ad7791diag.set_continous_mode();}}, 00102 {"adsetref", 1, [](){ad7791diag.set_reference_voltage();}}, 00103 {"adsetch", 1, [](){ad7791diag.set_channel();}}, 00104 #endif 00105 00106 #ifdef CN0216_PRESENT 00107 {"cninit" , 1, [](){cn0216diag.init();}}, 00108 {"cncal" , 1, [](){cn0216diag.calibrate();}}, 00109 {"cnrdw" , 0, [](){cn0216diag.read_weight();}}, 00110 #endif 00111 00112 #ifdef AD7790_PRESENT 00113 {"adrst", 0, [](){ad7790diag.reset();}}, 00114 {"adwrm", 1, [](){ad7790diag.write_mode();}}, 00115 {"adrdm", 0, [](){ad7790diag.read_mode();}}, 00116 {"adwrf", 1, [](){ad7790diag.write_filter();}}, 00117 {"adrdf", 0, [](){ad7790diag.read_filter();}}, 00118 {"adrdd", 0, [](){ad7790diag.read_data();}}, 00119 {"adrds", 0, [](){ad7790diag.read_status();}}, 00120 {"adread", 0, [](){ad7790diag.read_u16();}}, 00121 {"adreadv", 0, [](){ad7790diag.read_voltage();}}, 00122 {"adsetc", 1, [](){ad7790diag.set_continous_mode();}}, 00123 {"adsetref", 1, [](){ad7790diag.set_reference_voltage();}}, 00124 {"adsetch", 1, [](){ad7790diag.set_channel();}}, 00125 #endif 00126 00127 #ifdef AD5270_PRESENT 00128 {"rdwrr" , 1, [](){ad5270diag.write_RDAC();}}, 00129 {"rdrdr" , 0, [](){ad5270diag.read_RDAC();}}, 00130 {"rdwrcmd" , 2, [](){ad5270diag.write_cmd();}}, 00131 {"rdsetz" , 0, [](){ad5270diag.set_HiZ();}}, 00132 {"rd50en", 0, [](){ad5270diag.enable_50TP_programming();}}, 00133 {"rd50ds", 0, [](){ad5270diag.disable_50TP_programming();}}, 00134 {"rd50st", 0, [](){ad5270diag.store_50TP();}}, 00135 {"rd50a" , 0, [](){ad5270diag.read_50TP_last_address();}}, 00136 {"rd50m" , 1, [](){ad5270diag.read_50TP_memory();}}, 00137 {"rdwrc" , 1, [](){ad5270diag.write_ctrl_reg();}}, 00138 {"rdrdc" , 0, [](){ad5270diag.read_ctrl_reg();}}, 00139 {"rdrst" , 0, [](){ad5270diag.reset_RDAC();}}, 00140 {"rdchm" , 1, [](){ad5270diag.change_mode();}}, 00141 {"rdwrw" , 1, [](){ad5270diag.write_wiper_reg();}}, 00142 {"rdrdw" , 0, [](){ad5270diag.read_wiper_reg();}}, 00143 #endif 00144 00145 #ifdef CN0357_PRESENT 00146 {"cnwrdac" , 1, [](){cn0357diag.set_RDAC();}}, 00147 {"cnrppm" , 0, [](){cn0357diag.read_ppm();}}, 00148 {"cnparam" , 2, [](){cn0357diag.set_sensor_param();}} 00149 #endif 00150 00151 #ifdef ADXL362_PRESENT 00152 {"xlrst" , 0, [](){adxl362diag.reset();}}, 00153 {"xlscan" , 0, [](){adxl362diag.scan();}}, 00154 {"xlwr" , 2, [](){adxl362diag.write_reg();}}, 00155 {"xlrd" , 1, [](){adxl362diag.read_reg();}}, 00156 {"xlstat", 0 , [](){adxl362diag.read_status();}}, 00157 {"xlctl", 1 , [](){adxl362diag.write_ctl(); }}, 00158 {"xlftl", 1 , [](){adxl362diag.write_ftl(); }}, 00159 00160 {"xlfrn", 0, [](){adxl362diag.fifo_read_nr_of_entries(); }}, 00161 {"xlfset", 2, [](){adxl362diag.fifo_setup(); }}, 00162 {"xlfr16", 0, [](){adxl362diag.fifo_read_u16(); }}, 00163 {"xlfrs", 0, [](){adxl362diag.fifo_scan(); }}, 00164 {"xlintinit",0,[](){adxl362diag.intinit();}}, 00165 {"xlawake",0,[](){adxl362diag.checkawake();}}, 00166 #endif 00167 00168 00169 // UART 00170 {"uart" , 0, [](){test.putc('\n');}}, 00171 {"uartecho" , 1, [](){test.printf(cmdbuffer[1].c_str());}}, 00172 {"uartlisten",0 , [](){ 00173 char buff[100] = {0}; 00174 size_t readPosition = 0; 00175 00176 // read from console until newline 00177 while(1) { 00178 buff[readPosition] = test.getc(); 00179 if(buff[readPosition] == '\n' || buff[readPosition] == '\r') { 00180 buff[readPosition] = ' '; 00181 break; 00182 } 00183 readPosition++; 00184 } 00185 readPosition++; 00186 buff[readPosition] = '\0'; 00187 //create std::string from char buffer 00188 00189 pc.printf("%s",buff);}}, 00190 00191 #ifdef AD7124_PRESENT 00192 {"adrst" , 0, [](){ad7124diag.reset();}}, 00193 {"adrd" , 1, [](){ad7124diag.read_reg();}}, 00194 {"adrdd" , 0, [](){ad7124diag.read_data();}}, 00195 {"adrdv" , 0, [](){ad7124diag.read_volt();}}, 00196 {"adwr" , 2, [](){ad7124diag.write_reg();}}, 00197 {"adsetup",0,[]() {ad7124diag.setup();pc.printf("setup done\r\n");ad7124diag.mvpInit();pc.printf("init done\r\n");}} 00198 00199 00200 00201 #endif 00202 00203 #ifdef CN0398_PRESENT 00204 {"cnrst" , 0, [](){cn0398diag.reset();}}, 00205 {"cnrd" , 1, [](){cn0398diag.read_reg();}}, 00206 /*{"cnrdd" , 0, [](){ad7124diag.read_data();}}, 00207 {"cnrdv" , 0, [](){ad7124diag.read_volt();}},*/ 00208 {"cnwr" , 2, [](){cn0398diag.write_reg();}}, 00209 {"cnsetup",0,[]() {cn0398diag.init();pc.printf("init done\r\n");}}, 00210 {"cnled0",1,[]() {cn0398diag.toggle_output(1,strtol(cmdbuffer[1].c_str(), NULL, 16)); pc.printf("LED1 toggled"); }}, 00211 {"cnled1",1,[]() {cn0398diag.toggle_output(2,strtol(cmdbuffer[1].c_str(), NULL, 16));pc.printf("LED2 toggled");}}, 00212 {"cnoffp",0,[]() {cn0398diag.offsetph();pc.printf("OffsetVoltage measured");}}, 00213 {"cnpcal0",1,[]() {cn0398diag.calibp(0);pc.printf("calibration point 0");}}, 00214 {"cnpcal1",1,[]() {cn0398diag.calibp(1);pc.printf("calibration point 1");}}, 00215 {"cnreadp",0,[]() {cn0398diag.readp();pc.printf("calibration point 1");}}, 00216 {"cnreadm",0,[]() {cn0398diag.readm();pc.printf("calibration point 1");}}, 00217 {"cnreadt",0,[]() {cn0398diag.readt();pc.printf("Temperature Read");}}, 00218 00219 00220 00221 00222 #endif 00223 00224 #ifdef CN0397_PRESENT 00225 {"cnrst" , 0, [](){cn0397diag.reset();}}, 00226 /* {"cnrd" , 1, [](){cn0397diag.read_reg();}}, 00227 {"cnwr" , 2, [](){cn0397diag.write_reg();}},*/ 00228 {"cnsetup",0,[]() {cn0397diag.init();pc.printf("init done\r\n");}}, 00229 {"cnread",0,[]() {cn0397diag.read();pc.printf("calibration point 1");}}, 00230 #endif 00231 00232 #ifdef CN0396_PRESENT 00233 {"cntrst" , 0, [](){pc.printf("reset temp sensor \r\n");cn0396diag.dut.temp.reset();}}, 00234 {"cntrds" , 0, [](){pc.printf("temp status: %x \r\n",cn0396diag.dut.temp.read_status());}}, 00235 {"cntrdt" , 0, [](){pc.printf("temp val: %x \r\n",cn0396diag.dut.temp.read_temp());}}, 00236 {"cntrdc" , 0, [](){pc.printf("temp val: %x \r\n",cn0396diag.dut.temp.read_config());}}, 00237 {"cntwcf" , 1, [](){pc.printf("wrote config: %x \r\n",strtol(cmdbuffer[1].c_str(), NULL, 16)); 00238 cn0396diag.dut.temp.write_config(strtol(cmdbuffer[1].c_str(), NULL, 16));}}, 00239 {"cnardi" , 0, [](){pc.printf("adid : %x \r\n",cn0396diag.dut.ad.get_register_value(3,1,true));}}, 00240 00241 {"rdwrr" , 1, [](){pc.printf("aa");ad5270diag.write_RDAC();}}, 00242 {"rdrdr" , 0, [](){ad5270diag.read_RDAC();}}, 00243 {"rdwrcmd" , 2, [](){ad5270diag.write_cmd();}}, 00244 {"rdsetz" , 0, [](){ad5270diag.set_HiZ();}}, 00245 {"rd50en", 0, [](){ad5270diag.enable_50TP_programming();}}, 00246 {"rd50ds", 0, [](){ad5270diag.disable_50TP_programming();}}, 00247 {"rd50st", 0, [](){ad5270diag.store_50TP();}}, 00248 {"rd50a" , 0, [](){ad5270diag.read_50TP_last_address();}}, 00249 {"rd50m" , 1, [](){ad5270diag.read_50TP_memory();}}, 00250 {"rdwrc" , 1, [](){ad5270diag.write_ctrl_reg();}}, 00251 {"rdrdc" , 0, [](){ad5270diag.read_ctrl_reg();}}, 00252 {"rdrst" , 0, [](){ad5270diag.reset_RDAC();}}, 00253 {"rdchm" , 1, [](){ad5270diag.change_mode();}}, 00254 {"rdwrw" , 1, [](){ad5270diag.write_wiper_reg();}}, 00255 {"rdrdw" , 0, [](){ad5270diag.read_wiper_reg();}}, 00256 00257 00258 #endif 00259 }; 00260 // *INDENT-ON* 00261 00262 00263 00264 void read_from_console() 00265 { 00266 char buffer[100] = {0}; 00267 size_t readPosition = 0; 00268 00269 // read from console until newline 00270 while(1) { 00271 buffer[readPosition] = pc.getc(); 00272 if(buffer[readPosition] == '\n' || buffer[readPosition] == '\r') { 00273 buffer[readPosition] = ' '; 00274 break; 00275 } 00276 readPosition++; 00277 } 00278 readPosition++; 00279 buffer[readPosition] = '\0'; 00280 //create std::string from char buffer 00281 string s(buffer); 00282 00283 // create std::vector of std:string, each string contains parameter 00284 size_t pos = 0; 00285 string delimiter = " "; 00286 string token; 00287 while ((pos = s.find(delimiter)) != std::string::npos) { 00288 token = s.substr(0, pos); 00289 cmdbuffer.push_back(token); 00290 s.erase(0, pos + delimiter.length()); 00291 } 00292 00293 } 00294 00295 void run_command() 00296 { 00297 for(auto i : cmdlist) { 00298 if(i.cmd_str == cmdbuffer[0]) { 00299 if(static_cast<int>(cmdbuffer.size()) - 1 == i.nr_of_param || i.nr_of_param == commands::VAR) { 00300 pc.printf("RX> "); 00301 i.fktPtr(); 00302 } else { 00303 pc.printf("RX> "); 00304 pc.printf("Command %s expects %d parameters, found %d", i.cmd_str.c_str(), i.nr_of_param, cmdbuffer.size() - 1); 00305 } 00306 return; 00307 } 00308 } 00309 pc.printf("RX> "); 00310 pc.printf("Command %s not found", cmdbuffer[0].c_str()); 00311 00312 } 00313 00314 int main() 00315 00316 { 00317 pc.baud(115200); 00318 test.baud(76800); 00319 // ad7791.frequency(100000); 00320 pc.printf("\r\n#### DrvDiag ####\r\n"); 00321 00322 while(1) { 00323 pc.printf("\r\nTX> "); 00324 read_from_console(); 00325 run_command(); 00326 cmdbuffer.clear(); 00327 } 00328 00329 } 00330
Generated on Tue Jul 12 2022 17:59:52 by
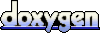