Analog Joystick Library
Fork of SparkfunAnalogJoystick by
Embed:
(wiki syntax)
Show/hide line numbers
SparkfunAnalogJoystick.cpp
Go to the documentation of this file.
00001 /** Analog Joystick APIs 00002 * Used as a user interface for Sparkfun Analog Joystick Module 00003 * @file SparkfunAnalogJoystick.cpp 00004 * @author Yuan,ZHANG; Jiajie,YANG 00005 */ 00006 00007 #include "SparkfunAnalogJoystick.h" 00008 #include "math.h" 00009 00010 #define M_PI 3.14159265358979323846 00011 00012 /** Constructor of Joystick object 00013 */ 00014 SparkfunAnalogJoystick::SparkfunAnalogJoystick(PinName vert, PinName horz, PinName sel): VERT(vert), HORZ(horz), SEL(sel) 00015 { 00016 SEL.mode(PullUp); 00017 } 00018 00019 //! Get the button status 00020 int SparkfunAnalogJoystick::button(){ 00021 return 1-SEL; 00022 } 00023 00024 //! X axis value, reverse the value to get the x value in a normal Cartesian coordinate system 00025 float SparkfunAnalogJoystick::xAxis(){ 00026 return -(HORZ-0.5)*2; 00027 } 00028 00029 //! Y axis value 00030 float SparkfunAnalogJoystick::yAxis(){ 00031 return (VERT-0.5)*2; 00032 } 00033 00034 //! Calculate the angle value in a polar coordinate system 00035 float SparkfunAnalogJoystick::angle(){ 00036 float horz=-(HORZ-0.5)*2; 00037 float vert=(VERT-0.5)*2; 00038 float angle=0; 00039 if (vert==0&&horz>0) return 0; 00040 if (vert==0&&horz<0) return 180; 00041 if (horz==0&&vert>0) return 90; 00042 if (horz==0&&vert<0) return 270; 00043 if (horz>0) angle=atan(vert/horz)*180/M_PI; 00044 else angle=180+atan(vert/horz)*180/M_PI; 00045 if (angle<0) angle+=360; 00046 return angle; 00047 } 00048 00049 //! Calculate the normalized distance value in a polar coordinate system 00050 float SparkfunAnalogJoystick::distance(){ 00051 float horz=-(HORZ-0.5)*2; 00052 float vert=(VERT-0.5)*2; 00053 float angle=SparkfunAnalogJoystick::angle(); 00054 float oneAxis=tan(angle*M_PI/180.0); 00055 if (oneAxis<0) oneAxis=-oneAxis; 00056 if (oneAxis>1) oneAxis=1/oneAxis; 00057 float maxdistance=sqrt(1+oneAxis*oneAxis); 00058 float temp=horz*horz+vert*vert; 00059 return sqrt(temp)/maxdistance; 00060 00061 }
Generated on Tue Jul 12 2022 18:33:56 by
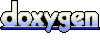