These are the examples provided for [[/users/frank26080115/libraries/LPC1700CMSIS_Lib/]] Note, the entire "program" is not compilable!
libnosys_gnu.c
00001 /*********************************************************************** 00002 * $Id:: libnosys_gnu.c 1388 2008-12-01 19:20:06Z pdurgesh $ 00003 * 00004 * Project: Linosys function for GNU c compiler 00005 * 00006 * Description: 00007 * Definitions for OS interface, stub function required by newlibc 00008 * used by Codesourcery GNU compiler. 00009 * 00010 *********************************************************************** 00011 * Software that is described herein is for illustrative purposes only 00012 * which provides customers with programming information regarding the 00013 * products. This software is supplied "AS IS" without any warranties. 00014 * NXP Semiconductors assumes no responsibility or liability for the 00015 * use of the software, conveys no license or title under any patent, 00016 * copyright, or mask work right to the product. NXP Semiconductors 00017 * reserves the right to make changes in the software without 00018 * notification. NXP Semiconductors also make no representation or 00019 * warranty that such application will be suitable for the specified 00020 * use without further testing or modification. 00021 **********************************************************************/ 00022 #if defined(__GNUC__) 00023 00024 #include "lpc_types.h" 00025 #include <errno.h> 00026 #include <sys/times.h> 00027 #include <sys/stat.h> 00028 00029 /* errno definition */ 00030 #undef errno 00031 extern int errno; 00032 00033 char *__env[1] = { 0 }; 00034 char **environ = __env; 00035 00036 int _close(int file) 00037 { 00038 return -1; 00039 } 00040 00041 int _execve(char *name, char **argv, char **env) 00042 { 00043 errno = ENOMEM; 00044 return -1; 00045 } 00046 int _exit() 00047 { 00048 return 0; 00049 } 00050 00051 int _fork() 00052 { 00053 errno = EAGAIN; 00054 return -1; 00055 } 00056 00057 int _fstat(int file, struct stat *st) 00058 { 00059 st->st_mode = S_IFCHR; 00060 return 0; 00061 } 00062 00063 int _getpid() 00064 { 00065 return 1; 00066 } 00067 00068 int _kill(int pid, int sig) 00069 { 00070 errno = EINVAL; 00071 return(-1); 00072 } 00073 00074 int isatty(int fildes) 00075 { 00076 return 1; 00077 } 00078 int _isatty(int fildes) 00079 { 00080 return 1; 00081 } 00082 00083 int _link(char *old, char *new) 00084 { 00085 errno = EMLINK; 00086 return -1; 00087 } 00088 00089 int _lseek(int file, int ptr, int dir) 00090 { 00091 return 0; 00092 } 00093 00094 int _open(const char *name, int flags, int mode) 00095 { 00096 return -1; 00097 } 00098 00099 int _read(int file, char *ptr, int len) 00100 { 00101 return 0; 00102 } 00103 00104 caddr_t _sbrk(int incr) 00105 { 00106 extern char estack; /* Defined by the linker */ 00107 static char *heap_end; 00108 char *prev_heap_end; 00109 00110 if (heap_end == 0) 00111 { 00112 heap_end = &estack; 00113 /* give 16KB area for stacks and use the rest of memory for heap*/ 00114 heap_end += 0x4000; 00115 } 00116 prev_heap_end = heap_end; 00117 00118 heap_end += incr; 00119 return (caddr_t) prev_heap_end; 00120 } 00121 00122 int _stat(char *file, struct stat *st) 00123 { 00124 st->st_mode = S_IFCHR; 00125 return 0; 00126 } 00127 int _times(struct tms *buf) 00128 { 00129 return -1; 00130 } 00131 00132 int _unlink(char *name) 00133 { 00134 errno = ENOENT; 00135 return -1; 00136 } 00137 00138 int _wait(int *status) 00139 { 00140 errno = ECHILD; 00141 return -1; 00142 } 00143 00144 int _write(int file, char *ptr, int len) 00145 { 00146 return 0; 00147 } 00148 00149 #endif /*__GNUC__*/
Generated on Tue Jul 12 2022 17:28:09 by
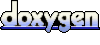