
Retro Invaders a space invaders clone by Chris Favreau. Written for the RetroMbuino development board from outrageouscircuits.com for the game programming contest.
input.h
00001 #include <mbed.h> 00002 00003 enum INPUT_BUTTON 00004 { 00005 eUp, 00006 eDown, 00007 eLeft, 00008 eRight, 00009 eCircle, 00010 eSquare, 00011 eLastButton, 00012 }; 00013 00014 #define BUTTON_DEBOUNCE_TICKS 3 00015 00016 class input 00017 { 00018 public: 00019 00020 input(); 00021 virtual ~input() {}; 00022 00023 void tick(); // Call this function at regular intervals (preferably the frame rate) 00024 00025 // Buttons 00026 int hit(INPUT_BUTTON button); 00027 bool pressed(INPUT_BUTTON button); 00028 void clear(); 00029 00030 // Accelerometer 00031 // X,Y,Z (0 to 100) 00032 int accel_x(); 00033 int accel_y(); 00034 int accel_z(); 00035 // Roll,Pitch (0 to 359) 00036 int accel_roll(); 00037 int accel_pitch(); 00038 00039 protected: 00040 00041 DigitalIn left; 00042 DigitalIn right; 00043 DigitalIn down; 00044 DigitalIn up; 00045 DigitalIn square; 00046 DigitalIn circle; 00047 int m_iPressedTicks[eLastButton]; 00048 int m_iHitCount[eLastButton]; 00049 void dobutton(INPUT_BUTTON button, bool bPressed); 00050 00051 static const char I2C_ADDR = 0x1C << 1; 00052 I2C i2c; 00053 void accelReadRegisters(char address, char* buffer, int len); 00054 int accelWriteRegister(char address, char value); 00055 float accelConvert(char* buffer); 00056 00057 float m_fAccelX; 00058 float m_fAccelY; 00059 float m_fAccelZ; 00060 };
Generated on Thu Jul 14 2022 20:06:44 by
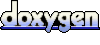