
Retro Invaders a space invaders clone by Chris Favreau. Written for the RetroMbuino development board from outrageouscircuits.com for the game programming contest.
input.cpp
00001 #include <math.h> 00002 #include "input.h" 00003 00004 input::input() : left(P0_14, PullUp), right(P0_11, PullUp), down(P0_12, PullUp), up(P0_13, PullUp), square(P0_16, PullUp), circle(P0_1, PullUp), i2c(P0_5, P0_4) 00005 { 00006 // Clear all the button states 00007 for (int i = 0; i < eLastButton; i++) 00008 { 00009 m_iPressedTicks[i] = 0; 00010 m_iHitCount[i] = 0; 00011 } 00012 00013 m_fAccelX = 0.0; 00014 m_fAccelY = 0.0; 00015 m_fAccelZ = 0.0; 00016 00017 i2c.frequency(400); 00018 accelWriteRegister(0x2A, 0x01); 00019 } 00020 00021 void input::dobutton(INPUT_BUTTON button, bool bPressed) 00022 { 00023 // If this button is not being debounced then set the ticks we can debounce the switch 00024 if (bPressed) 00025 { 00026 if (m_iPressedTicks[button] < 1) 00027 { 00028 // This is the first time this button was pressed since it was UP 00029 // Increment the hit count 00030 m_iHitCount[button]++; 00031 } 00032 // Reset the debounce counter 00033 m_iPressedTicks[button] = BUTTON_DEBOUNCE_TICKS; 00034 } 00035 // If this button IS not pressed and we have ticks left... deduct 1 tick 00036 if (!bPressed && (m_iPressedTicks[button] > 0)) 00037 m_iPressedTicks[button]--; 00038 } 00039 00040 void input::tick() 00041 { 00042 // Sample all of the buttons 00043 dobutton(eUp, up.read() == 0); 00044 dobutton(eDown, down.read() == 0); 00045 dobutton(eLeft, left.read() == 0); 00046 dobutton(eRight, right.read() == 0); 00047 dobutton(eCircle, circle.read() == 0); 00048 dobutton(eSquare, square.read() == 0); 00049 00050 // UGHHHHHHH - accelReadRegisters() is BALLS SLOW!!! Disable for NOW! 00051 /* 00052 // Sample the accelerometers 00053 char buffer[6]; 00054 accelReadRegisters(0x01, buffer, 6); 00055 m_fAccelX = accelConvert(buffer); 00056 m_fAccelY = accelConvert(buffer + 2); 00057 m_fAccelZ = accelConvert(buffer + 4); 00058 */ 00059 } 00060 00061 void input::clear() 00062 { 00063 for (int i = 0; i < eLastButton; i++) 00064 { 00065 m_iHitCount[i] = 0; 00066 m_iPressedTicks[i] = 0; 00067 } 00068 } 00069 00070 int input::hit(INPUT_BUTTON button) 00071 { 00072 if (button >= eLastButton) return 0; 00073 // Store the hit count for this button 00074 int hit_count = m_iHitCount[button]; 00075 // Clear the hit count 00076 m_iHitCount[button] = 0; 00077 // Return the stored hit count 00078 return hit_count; 00079 } 00080 00081 bool input::pressed(INPUT_BUTTON button) 00082 { 00083 if (button >= eLastButton) return false; 00084 00085 return (m_iPressedTicks[button] > 0); 00086 } 00087 00088 00089 int input::accel_x() 00090 { 00091 return (int)(m_fAccelX * 100.0); 00092 } 00093 00094 int input::accel_y() 00095 { 00096 return (int)(m_fAccelY * 100.0); 00097 } 00098 00099 int input::accel_z() 00100 { 00101 return (int)(m_fAccelZ * 100.0); 00102 } 00103 00104 int input::accel_roll() 00105 { 00106 // Roll and Pitch are swapped on the Retro... 00107 return (int)((atan(m_fAccelX / sqrt((m_fAccelY * m_fAccelY) + (m_fAccelZ * m_fAccelZ))) * 180.0) / 3.14159); 00108 } 00109 00110 int input::accel_pitch() 00111 { 00112 // Roll and Pitch are swapped on the Retro.. (Pitch is Roll, Roll is Pitch) 00113 return (int)((atan(m_fAccelY / sqrt((m_fAccelX * m_fAccelX) + (m_fAccelZ * m_fAccelZ))) * 180.0) / 3.14159); 00114 } 00115 00116 void input::accelReadRegisters(char address, char* buffer, int len) 00117 { 00118 // BALLS SLOW!!! 00119 i2c.write(I2C_ADDR, &address, 1, true); 00120 i2c.read(I2C_ADDR | 1, buffer, len); 00121 } 00122 00123 int input::accelWriteRegister(char address, char value) 00124 { 00125 char buffer[2] = { address, value }; 00126 return i2c.write(I2C_ADDR, buffer, 2); 00127 } 00128 00129 float input::accelConvert(char* buffer) 00130 { 00131 float val = ((buffer[0] << 2) | (buffer[1] >> 6)); 00132 if (val > 511.0) val -= 1024.0; 00133 return val / 512.0; 00134 }
Generated on Thu Jul 14 2022 20:06:44 by
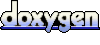