
Retro Invaders a space invaders clone by Chris Favreau. Written for the RetroMbuino development board from outrageouscircuits.com for the game programming contest.
Game.h
00001 #include "mbed.h" 00002 #include "./Display/display.h" // LCD Display Driver 00003 #include "./led/led.h" // LED Driver 00004 #include "./Input/input.h" // INPUT Driver 00005 #include "./Sound/sound.h" // SOUND Driver 00006 00007 #pragma once 00008 00009 enum GAME_MODE 00010 { 00011 eGameTitle, 00012 eGamePlay, 00013 eGameOver, 00014 }; 00015 00016 #define FRAMES_PER_SECOND 20 // unfortunately this is what we get... 00017 #define MS_PER_FRAME 50 00018 00019 class Game 00020 { 00021 int frame_timeout; 00022 int frame_count; 00023 int now; 00024 00025 Timer timer; 00026 display disp; 00027 AnalogIn ain; 00028 led lights; 00029 input inputs; 00030 Sound sound; 00031 00032 GAME_MODE mode; 00033 int game_step; 00034 bool paused; 00035 00036 void initialize(); 00037 bool dotick(); 00038 00039 void DoGameTitle(); 00040 void DoGamePlay(); 00041 void DoGameOver(); 00042 00043 uint8_t star_field_timer; 00044 void SetupStarfield(); 00045 void UpdateStarfield(); 00046 00047 void SetupInvaders(); 00048 void UpdateInvaders(); 00049 bool invaders[40]; 00050 int8_t active_invaders; 00051 int8_t invaders_inc; 00052 uint8_t invaders_timer; 00053 uint8_t invaders_wait_time; 00054 uint8_t invaders_frame; 00055 uint8_t invaders_x, invaders_y; 00056 bool invader_active_shot[4]; 00057 uint8_t invader_shot_interval; 00058 uint8_t invader_shot_x[4]; 00059 uint8_t invader_shot_y[4]; 00060 int8_t invader_shot_timer; 00061 uint8_t invader_march_note; 00062 00063 00064 void SetupPlayer(); 00065 void UpdatePlayer(); 00066 uint8_t player_x, player_y; 00067 bool player_shot_active; 00068 uint8_t player_shot_x, player_shot_y; 00069 00070 void SetupSaucer(); 00071 void UpdateSaucer(); 00072 bool saucer_active; 00073 int saucer_timer; 00074 int8_t saucer_inc; 00075 uint8_t saucer_x, saucer_y; 00076 00077 void SetupShields(); 00078 void UpdateShields(); 00079 uint8_t shield_x, shield_y; 00080 uint8_t shield_points[4]; 00081 00082 void CheckCollisions(); 00083 00084 void IncScore(int points); 00085 void UpdateScore(); 00086 void UpdateLives(); 00087 int hiscore; 00088 int score; 00089 uint8_t lives; 00090 uint8_t level; 00091 00092 uint8_t wait_timer; 00093 00094 void UpdateGameOverInvaders(); 00095 uint8_t game_over_msg; 00096 00097 public: 00098 00099 Game(); 00100 00101 void tick(); 00102 };
Generated on Thu Jul 14 2022 20:06:44 by
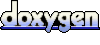