
Very short program to SET the MAC Address of MBED,
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 extern "C" void mbed_mac_address(char *s) 00004 { 00005 char mac[6]; 00006 mac[0] = 0x00; 00007 mac[1] = 0x02; 00008 mac[2] = 0xf7; 00009 mac[3] = 0xf0; 00010 mac[4] = 0x46; 00011 mac[5] = 0x4b; 00012 00013 memcpy(s, mac, 6); 00014 } 00015 00016 00017 // the one on my desk ........ 00 02 f7 f0 55 5e 00018 // the one on the fireplace .. 00 02 f7 f0 46 4b 00019 00020 // 00:02:f7:f0:46:4b .. from tweet program ? 00021 00022 // -> http://mbed.org/forum/mbed/topic/972/?page=1#comment-10318 00023 00024 // Going to write the MAC address from one of my MBED's to annother MBED. 00025 00026 DigitalOut myled(LED1); 00027 Serial pc(USBTX, USBRX); // tx, rx pc.baud(921600); 00028 00029 int main() 00030 { 00031 uint64_t uid = 0; 00032 char mmac[6]; 00033 char mac[6]; 00034 00035 pc.baud(921600); 00036 00037 pc.printf("Trying to overwrite MAC Address ..\r\n"); 00038 00039 mbed_mac_address(mmac); 00040 uid = mmac[0] << 40 | mmac[1] << 32 | 00041 mmac[2] << 24 | mmac[3] << 16 | 00042 mmac[4] << 8 | mmac[5] << 0; 00043 00044 pc.printf ("Here it is .. %02x %02x %02x %02x %02x %02x .. \r\n", mmac[0], mmac[1], mmac[2], mmac[3], mmac[4], mmac[5]); 00045 00046 while (1) 00047 { 00048 myled = 1; 00049 wait(0.2); 00050 myled = 0; 00051 wait(0.2); 00052 } 00053 } 00054 00055 00056
Generated on Tue Jul 19 2022 11:30:54 by
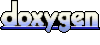