Dallas 1-wire driver with DS18B20 temperature sensor support; custom bus driver to work with https://emir.googlecode.com/svn/emir2/trunk/eagle/emir-shield.sch
Dependents: testing_RTC_OneWire EMIRv2
1wire.h
00001 #ifndef _1WIRE_H 00002 #define _1WIRE_H 00003 00004 /* Dallas 1-wire driver with DS18B20 temperature sensor support 00005 * 00006 * Copyright (c) 2014 Ales Povalac, MIT License 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00009 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00010 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00011 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in all copies or 00015 * substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00018 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00020 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00022 */ 00023 00024 class OneWire 00025 { 00026 public: 00027 OneWire(PinName OwUp, PinName OwDn, PinName OwIn); 00028 00029 void ConvertAll(bool wait); 00030 int ReadTemperature(uint8_t *ROMID, int *result); 00031 00032 int First(uint8_t *ROMID); 00033 int Next(uint8_t *ROMID); 00034 00035 void WriteByte(uint8_t data); 00036 uint8_t ReadByte(void); 00037 void SendCmd(uint8_t *ROMID, uint8_t cmd); 00038 void CRC(uint8_t x, uint8_t *crc); 00039 00040 private: 00041 DigitalOut _OwUp; 00042 DigitalOut _OwDn; 00043 DigitalIn _OwIn; 00044 00045 uint8_t OW_LastDevice; 00046 uint8_t OW_LastDiscrepancy; 00047 uint8_t OW_LastFamilyDiscrepancy; 00048 00049 int Reset(void); 00050 void WriteBit(int bit); 00051 int ReadBit(void); 00052 }; 00053 00054 00055 /* INTERNAL CONSTANTS everything below this line */ 00056 00057 #define ERR_BADCRC 0x8000 00058 #define ERR_BADFAMILY 0x8001 00059 00060 /* Return codes for OWFirst()/OWNext() */ 00061 #define OW_BADWIRE -3 00062 #define OW_BADCRC -2 00063 #define OW_NOPRESENCE -1 00064 #define OW_NOMODULES 0 00065 #define OW_FOUND 1 00066 00067 /* General 1 wire commands */ 00068 #define OW_SEARCH_ROM_CMD 0xF0 00069 #define OW_READ_ROM_CMD 0x33 00070 #define OW_MATCH_ROM_CMD 0x55 00071 #define OW_SKIP_ROM_CMD 0xCC 00072 00073 /* DS1820 commands */ 00074 #define OW_CONVERT_T_CMD 0x44 00075 #define OW_RD_SCR_CMD 0xBE 00076 #define OW_WR_SCR_CMD 0x4E 00077 00078 00079 /* 1-wire delays */ 00080 #define DELAY_A() wait_us(6) 00081 #define DELAY_B() wait_us(64) 00082 #define DELAY_C() wait_us(60) 00083 #define DELAY_D() wait_us(10) 00084 #define DELAY_E() wait_us(9) 00085 #define DELAY_F() wait_us(55) 00086 #define DELAY_G() 00087 #define DELAY_H() wait_us(480) 00088 #define DELAY_I() wait_us(70) 00089 #define DELAY_J() wait_us(410) 00090 00091 /* Other */ 00092 #define CONVERT_T_DELAY 750 00093 00094 #endif
Generated on Tue Jul 12 2022 18:13:10 by
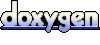