Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
mDot.h
00001 /********************************************************************** 00002 * COPYRIGHT 2015 MULTI-TECH SYSTEMS, INC. 00003 * 00004 * Redistribution and use in source and binary forms, with or without modification, 00005 * are permitted provided that the following conditions are met: 00006 * 1. Redistributions of source code must retain the above copyright notice, 00007 * this list of conditions and the following disclaimer. 00008 * 2. Redistributions in binary form must reproduce the above copyright notice, 00009 * this list of conditions and the following disclaimer in the documentation 00010 * and/or other materials provided with the distribution. 00011 * 3. Neither the name of MULTI-TECH SYSTEMS, INC. nor the names of its contributors 00012 * may be used to endorse or promote products derived from this software 00013 * without specific prior written permission. 00014 * 00015 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00016 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00017 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00018 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00019 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00020 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00021 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00022 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00023 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00024 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00025 * 00026 ****************************************************************************** 00027 */ 00028 00029 #ifndef MDOT_H 00030 #define MDOT_H 00031 00032 #include "mbed.h" 00033 #include "rtos.h" 00034 #include "Mote.h" 00035 #include <vector> 00036 #include <map> 00037 #include <string> 00038 00039 class mDotEvent; 00040 class LoRaConfig; 00041 00042 00043 class mDot { 00044 friend class mDotEvent; 00045 00046 private: 00047 00048 mDot(lora::ChannelPlan* plan); 00049 ~mDot(); 00050 00051 void initLora(); 00052 00053 void setLastError(const std::string& str); 00054 00055 static bool validateBaudRate(const uint32_t& baud); 00056 static bool validateFrequencySubBand(const uint8_t& band); 00057 bool validateDataRate(const uint8_t& dr); 00058 00059 int32_t joinBase(const uint32_t& retries); 00060 int32_t sendBase(const std::vector<uint8_t>& data, const bool& confirmed = false, const bool& blocking = true, const bool& highBw = false); 00061 void waitForPacket(); 00062 void waitForLinkCheck(); 00063 00064 mDot(const mDot&); 00065 mDot& operator=(const mDot&); 00066 00067 uint32_t RTC_ReadBackupRegister(uint32_t RTC_BKP_DR); 00068 void RTC_WriteBackupRegister(uint32_t RTC_BKP_DR, uint32_t Data); 00069 00070 void RTC_DisableWakeupTimer(); 00071 void RTC_EnableWakeupTimer(); 00072 00073 void enterStopMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00074 void enterStandbyMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00075 00076 static mDot* _instance; 00077 00078 lora::Mote* _mote; 00079 LoRaConfig* _config; 00080 lora::Settings _settings; 00081 mDotEvent* _events; 00082 00083 std::string _last_error; 00084 static const uint32_t _baud_rates[]; 00085 00086 uint8_t _linkFailCount; 00087 uint8_t _class; 00088 InterruptIn* _wakeup; 00089 PinName _wakeup_pin; 00090 00091 typedef enum { 00092 OFF, 00093 ON, 00094 BLINK, 00095 } state; 00096 00097 public: 00098 00099 #if defined(TARGET_MTS_MDOT_F411RE) 00100 typedef enum { 00101 FM_APPEND = (1 << 0), 00102 FM_TRUNC = (1 << 1), 00103 FM_CREAT = (1 << 2), 00104 FM_RDONLY = (1 << 3), 00105 FM_WRONLY = (1 << 4), 00106 FM_RDWR = (FM_RDONLY | FM_WRONLY), 00107 FM_DIRECT = (1 << 5) 00108 } FileMode; 00109 #endif /* TARGET_MTS_MDOT_F411RE */ 00110 00111 typedef enum { 00112 MDOT_OK = 0, 00113 MDOT_INVALID_PARAM = -1, 00114 MDOT_TX_ERROR = -2, 00115 MDOT_RX_ERROR = -3, 00116 MDOT_JOIN_ERROR = -4, 00117 MDOT_TIMEOUT = -5, 00118 MDOT_NOT_JOINED = -6, 00119 MDOT_ENCRYPTION_DISABLED = -7, 00120 MDOT_NO_FREE_CHAN = -8, 00121 MDOT_TEST_MODE = -9, 00122 MDOT_NO_ENABLED_CHAN = -10, 00123 MDOT_AGGREGATED_DUTY_CYCLE = -11, 00124 MDOT_MAX_PAYLOAD_EXCEEDED = -12, 00125 MDOT_LBT_CHANNEL_BUSY = -13, 00126 MDOT_NOT_IDLE = -14, 00127 MDOT_ERROR = -1024, 00128 } mdot_ret_code; 00129 00130 enum JoinMode { 00131 MANUAL = 0, 00132 OTA, 00133 AUTO_OTA, 00134 PEER_TO_PEER 00135 }; 00136 00137 enum Mode { 00138 COMMAND_MODE, 00139 SERIAL_MODE 00140 }; 00141 00142 enum RX_Output { 00143 HEXADECIMAL, 00144 BINARY, 00145 EXTENDED, 00146 EXTENDED_HEX 00147 }; 00148 00149 enum DataRates { 00150 DR0, 00151 DR1, 00152 DR2, 00153 DR3, 00154 DR4, 00155 DR5, 00156 DR6, 00157 DR7, 00158 DR8, 00159 DR9, 00160 DR10, 00161 DR11, 00162 DR12, 00163 DR13, 00164 DR14, 00165 DR15 00166 }; 00167 00168 enum FrequencySubBands { 00169 FSB_ALL, 00170 FSB_1, 00171 FSB_2, 00172 FSB_3, 00173 FSB_4, 00174 FSB_5, 00175 FSB_6, 00176 FSB_7, 00177 FSB_8 00178 }; 00179 00180 enum wakeup_mode { 00181 RTC_ALARM, 00182 INTERRUPT, 00183 RTC_ALARM_OR_INTERRUPT 00184 }; 00185 00186 enum UserBackupRegs { 00187 UBR0, 00188 UBR1, 00189 UBR2, 00190 UBR3, 00191 UBR4, 00192 UBR5, 00193 UBR6, 00194 UBR7, 00195 UBR8, 00196 UBR9 00197 #if defined (TARGET_XDOT_L151CC) 00198 ,UBR10, 00199 UBR11, 00200 UBR12, 00201 UBR13, 00202 UBR14, 00203 UBR15, 00204 UBR16, 00205 UBR17, 00206 UBR18, 00207 UBR19, 00208 UBR20, 00209 UBR21 00210 #endif /* TARGET_XDOT_L151CC */ 00211 }; 00212 00213 #if defined(TARGET_MTS_MDOT_F411RE) 00214 typedef struct { 00215 int16_t fd; 00216 char name[33]; 00217 uint32_t size; 00218 } mdot_file; 00219 #endif /* TARGET_MTS_MDOT_F411RE */ 00220 00221 typedef struct { 00222 uint32_t Up; 00223 uint32_t Down; 00224 uint32_t Joins; 00225 uint32_t JoinFails; 00226 uint32_t MissedAcks; 00227 uint32_t CRCErrors; 00228 } mdot_stats; 00229 00230 typedef struct { 00231 int16_t last; 00232 int16_t min; 00233 int16_t max; 00234 int16_t avg; 00235 } rssi_stats; 00236 00237 typedef struct { 00238 int16_t last; 00239 int16_t min; 00240 int16_t max; 00241 int16_t avg; 00242 } snr_stats; 00243 00244 typedef struct { 00245 bool status; 00246 uint8_t dBm; 00247 uint32_t gateways; 00248 std::vector<uint8_t> payload; 00249 } link_check; 00250 00251 typedef struct { 00252 int32_t status; 00253 int16_t rssi; 00254 int16_t snr; 00255 } ping_response; 00256 00257 static std::string JoinModeStr(uint8_t mode); 00258 static std::string ModeStr(uint8_t mode); 00259 static std::string RxOutputStr(uint8_t format); 00260 static std::string DataRateStr(uint8_t rate); 00261 static std::string FrequencyBandStr(uint8_t band); 00262 static std::string FrequencySubBandStr(uint8_t band); 00263 00264 #if defined(TARGET_MTS_MDOT_F411RE) 00265 uint32_t UserRegisters[10]; 00266 #else 00267 uint32_t UserRegisters[22]; 00268 #endif /* TARGET_MTS_MDOT_F411RE */ 00269 00270 /** 00271 * Get a handle to the singleton object 00272 * @param plan the channel plan to use 00273 * @returns pointer to mDot object 00274 */ 00275 static mDot* getInstance(lora::ChannelPlan* plan); 00276 00277 /** 00278 * Can only be used after a dot has 00279 * configured with a plan 00280 * @returns pointer to mDot object 00281 */ 00282 static mDot* getInstance(); 00283 00284 void setEvents(mDotEvent* events); 00285 00286 /** 00287 * 00288 * Get library version information 00289 * @returns string containing library version information 00290 */ 00291 std::string getId(); 00292 00293 /** 00294 * Get MTS LoRa version information 00295 * @returns string containing MTS LoRa version information 00296 */ 00297 std::string getMtsLoraId(); 00298 00299 00300 /** 00301 * MAC version 00302 * 00303 * @return string containing version information of supported LoRaWAN MAC Version 00304 */ 00305 const char* getMACVersion(); 00306 00307 /** 00308 * Perform a soft reset of the system 00309 */ 00310 void resetCpu(); 00311 00312 /** 00313 * Reset config to factory default 00314 */ 00315 void resetConfig(); 00316 00317 /** 00318 * Save config data to non volatile memory 00319 * @returns true if success, false if failure 00320 */ 00321 bool saveConfig(); 00322 00323 /** 00324 * Set the log level for the library 00325 * options are: 00326 * NONE_LEVEL - logging is off at this level 00327 * FATAL_LEVEL - only critical errors will be reported 00328 * ERROR_LEVEL 00329 * WARNING_LEVEL 00330 * INFO_LEVEL 00331 * DEBUG_LEVEL 00332 * TRACE_LEVEL - every detail will be reported 00333 * @param level the level to log at 00334 * @returns MDOT_OK if success 00335 */ 00336 int32_t setLogLevel(const uint8_t& level); 00337 00338 /** 00339 * Get the current log level for the library 00340 * @returns current log level 00341 */ 00342 uint8_t getLogLevel(); 00343 00344 /** 00345 * Seed pseudo RNG in LoRaMac layer, uses random value from radio RSSI reading by default 00346 * @param seed for RNG 00347 */ 00348 void seedRandom(uint32_t seed); 00349 00350 /** 00351 * @returns true if MAC command answers are ready to be sent 00352 */ 00353 bool hasMacCommands(); 00354 00355 00356 uint8_t setChannelPlan(lora::ChannelPlan* plan); 00357 00358 lora::Settings* getSettings(); 00359 00360 /** 00361 * Returns boolean indicative of start-up from standby mode 00362 * @returns true if dot woke from standby 00363 */ 00364 bool getStandbyFlag(); 00365 00366 std::vector<uint16_t> getChannelMask(); 00367 00368 int32_t setChannelMask(uint8_t offset, uint16_t mask); 00369 00370 /** 00371 * Add a channel 00372 * @returns MDOT_OK 00373 */ 00374 int32_t addChannel(uint8_t index, uint32_t frequency, uint8_t datarateRange); 00375 00376 /** 00377 * Add a downlink channel 00378 * @returns MDOT_OK 00379 */ 00380 int32_t addDownlinkChannel(uint8_t index, uint32_t frequency); 00381 00382 /** 00383 * Get list of channel frequencies currently in use 00384 * @returns vector of channels currently in use 00385 */ 00386 std::vector<uint32_t> getChannels(); 00387 00388 /** 00389 * Get list of downlink channel frequencies currently in use 00390 * @returns vector of channels currently in use 00391 */ 00392 std::vector<uint32_t> getDownlinkChannels(); 00393 00394 /** 00395 * Get list of channel datarate ranges currently in use 00396 * @returns vector of datarate ranges currently in use 00397 */ 00398 std::vector<uint8_t> getChannelRanges(); 00399 00400 /** 00401 * Get list of channel frequencies in config file to be used as session defaults 00402 * @returns vector of channels in config file 00403 */ 00404 std::vector<uint32_t> getConfigChannels(); 00405 00406 /** 00407 * Get list of channel datarate ranges in config file to be used as session defaults 00408 * @returns vector of datarate ranges in config file 00409 */ 00410 std::vector<uint8_t> getConfigChannelRanges(); 00411 00412 /** 00413 * Get default frequency band 00414 * @returns frequency band the device was manufactured for 00415 */ 00416 uint8_t getDefaultFrequencyBand(); 00417 00418 /** 00419 * Set frequency sub band 00420 * only applicable if frequency band is set for United States (FB_915) 00421 * sub band 0 will allow the radio to use all 64 channels 00422 * sub band 1 - 8 will allow the radio to use the 8 channels in that sub band 00423 * for use with Conduit gateway and MTAC_LORA, use sub bands 1 - 8, not sub band 0 00424 * @param band the sub band to use (0 - 8) 00425 * @returns MDOT_OK if success 00426 */ 00427 int32_t setFrequencySubBand(const uint8_t& band); 00428 00429 /** 00430 * Get frequency sub band 00431 * @returns frequency sub band currently in use 00432 */ 00433 uint8_t getFrequencySubBand(); 00434 00435 /** 00436 * Get frequency band 00437 * @returns frequency band (channel plan) currently in use 00438 */ 00439 uint8_t getFrequencyBand(); 00440 00441 /** 00442 * Get channel plan name 00443 * @returns name of channel plan currently in use 00444 */ 00445 std::string getChannelPlanName(); 00446 00447 /** 00448 * Get the datarate currently in use within the MAC layer 00449 * returns 0-15 00450 */ 00451 uint8_t getSessionDataRate(); 00452 00453 00454 /** 00455 * Get the current max EIRP used in the channel plan 00456 * May be changed by the network server 00457 * returns 0-36 00458 */ 00459 uint8_t getSessionMaxEIRP(); 00460 00461 /** 00462 * Set the current max EIRP used in the channel plan 00463 * May be changed by the network server 00464 * accepts 0-36 00465 */ 00466 void setSessionMaxEIRP(uint8_t max); 00467 00468 /** 00469 * Get the current downlink dwell time used in the channel plan 00470 * May be changed by the network server 00471 * returns 0-1 00472 */ 00473 uint8_t getSessionDownlinkDwelltime(); 00474 00475 /** 00476 * Set the current downlink dwell time used in the channel plan 00477 * May be changed by the network server 00478 * accepts 0-1 00479 */ 00480 void setSessionDownlinkDwelltime(uint8_t dwell); 00481 00482 /** 00483 * Get the current uplink dwell time used in the channel plan 00484 * May be changed by the network server 00485 * returns 0-1 00486 */ 00487 uint8_t getSessionUplinkDwelltime(); 00488 00489 /** 00490 * Set the current uplink dwell time used in the channel plan 00491 * May be changed by the network server 00492 * accepts 0-1 00493 */ 00494 void setSessionUplinkDwelltime(uint8_t dwell); 00495 00496 /** 00497 * Set the current downlink dwell time used in the channel plan 00498 * May be changed by the network server 00499 * accepts 0-1 00500 */ 00501 uint32_t getListenBeforeTalkTime(uint8_t ms); 00502 00503 /** 00504 * Set the current downlink dwell time used in the channel plan 00505 * May be changed by the network server 00506 * accepts 0-1 00507 */ 00508 void setListenBeforeTalkTime(uint32_t ms); 00509 00510 /** 00511 * Set public network mode 00512 * 0:PRIVATE_MTS, 1:PUBLIC_LORAWAN, 2:PRIVATE_LORAWAN 00513 * PRIVATE_MTS - Sync Word 0x12, US/AU Downlink frequencies per Frequency Sub Band 00514 * PUBLIC_LORAWAN - Sync Word 0x34 00515 * PRIVATE_LORAWAN - Sync Word 0x12 00516 * 00517 * The default Join Delay is 5 seconds 00518 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00519 * The Join Delay must be changed independently of Public Network setting 00520 * 00521 * @see lora::NetworkType 00522 * @returns MDOT_OK if success 00523 */ 00524 int32_t setPublicNetwork(const uint8_t& val); 00525 00526 /** 00527 * Get public network mode 00528 * 00529 * The default Join Delay is 5 seconds 00530 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00531 * The Join Delay must be changed independently of Public Network setting 00532 * 00533 * @see lora:NetworkType 00534 * @returns 0:PRIVATE_MTS, 1:PUBLIC_LORAWAN, 2:PRIVATE_LORAWAN 00535 */ 00536 uint8_t getPublicNetwork(); 00537 00538 /** 00539 * Get the device ID 00540 * @returns vector containing the device ID (size 8) 00541 */ 00542 std::vector<uint8_t> getDeviceId(); 00543 00544 /** 00545 * Get the device port to be used for lora application data (1-223) 00546 * @returns port 00547 */ 00548 uint8_t getAppPort(); 00549 00550 /** 00551 * Set the device port to be used for lora application data (1-223) 00552 * @returns MDOT_OK if success 00553 */ 00554 int32_t setAppPort(uint8_t port); 00555 00556 /** 00557 * Set the device class A, B or C 00558 * @returns MDOT_OK if success 00559 */ 00560 int32_t setClass(std::string newClass); 00561 00562 /** 00563 * Get the device class A, B or C 00564 * @returns MDOT_OK if success 00565 */ 00566 std::string getClass(); 00567 00568 /** 00569 * Get the max packet length with current settings 00570 * @returns max packet length 00571 */ 00572 uint8_t getMaxPacketLength(); 00573 00574 /** 00575 * Set network address 00576 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00577 * @param addr a vector of 4 bytes 00578 * @returns MDOT_OK if success 00579 */ 00580 int32_t setNetworkAddress(const std::vector<uint8_t>& addr); 00581 00582 /** 00583 * Get network address 00584 * @returns vector containing network address (size 4) 00585 */ 00586 std::vector<uint8_t> getNetworkAddress(); 00587 00588 /** 00589 * Set network session key 00590 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00591 * @param key a vector of 16 bytes 00592 * @returns MDOT_OK if success 00593 */ 00594 int32_t setNetworkSessionKey(const std::vector<uint8_t>& key); 00595 00596 /** 00597 * Get network session key 00598 * @returns vector containing network session key (size 16) 00599 */ 00600 std::vector<uint8_t> getNetworkSessionKey(); 00601 00602 /** 00603 * Set data session key 00604 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00605 * @param key a vector of 16 bytes 00606 * @returns MDOT_OK if success 00607 */ 00608 int32_t setDataSessionKey(const std::vector<uint8_t>& key); 00609 00610 /** 00611 * Get data session key 00612 * @returns vector containing data session key (size 16) 00613 */ 00614 std::vector<uint8_t> getDataSessionKey(); 00615 00616 /** 00617 * Set network name 00618 * for use with OTA & AUTO_OTA network join modes 00619 * generates network ID (crc64 of name) automatically 00620 * @param name a string of of at least 8 bytes and no more than 128 bytes 00621 * @return MDOT_OK if success 00622 */ 00623 int32_t setNetworkName(const std::string& name); 00624 00625 /** 00626 * Get network name 00627 * @return string containing network name (size 8 to 128) 00628 */ 00629 std::string getNetworkName(); 00630 00631 /** 00632 * Set network ID 00633 * for use with OTA & AUTO_OTA network join modes 00634 * setting network ID via this function sets network name to empty 00635 * @param id a vector of 8 bytes 00636 * @returns MDOT_OK if success 00637 */ 00638 int32_t setNetworkId(const std::vector<uint8_t>& id); 00639 00640 /** 00641 * Get network ID 00642 * @returns vector containing network ID (size 8) 00643 */ 00644 std::vector<uint8_t> getNetworkId(); 00645 00646 /** 00647 * Set network passphrase 00648 * for use with OTA & AUTO_OTA network join modes 00649 * generates network key (cmac of passphrase) automatically 00650 * @param name a string of of at least 8 bytes and no more than 128 bytes 00651 * @return MDOT_OK if success 00652 */ 00653 int32_t setNetworkPassphrase(const std::string& passphrase); 00654 00655 /** 00656 * Get network passphrase 00657 * @return string containing network passphrase (size 8 to 128) 00658 */ 00659 std::string getNetworkPassphrase(); 00660 00661 /** 00662 * Set network key 00663 * for use with OTA & AUTO_OTA network join modes 00664 * setting network key via this function sets network passphrase to empty 00665 * @param id a vector of 16 bytes 00666 * @returns MDOT_OK if success 00667 */ 00668 int32_t setNetworkKey(const std::vector<uint8_t>& id); 00669 00670 /** 00671 * Get generic app key 00672 * @returns a vector containing key (size 16) 00673 */ 00674 std::vector<uint8_t> getGenAppKey(); 00675 00676 /** 00677 * Set generic app key 00678 * for use with Multicast key exchange 00679 * @param id a vector of 16 bytes 00680 * @returns MDOT_OK if success 00681 */ 00682 int32_t setGenAppKey(const std::vector<uint8_t>& id); 00683 00684 /** 00685 * Get network key 00686 * @returns a vector containing network key (size 16) 00687 */ 00688 std::vector<uint8_t> getNetworkKey(); 00689 00690 /** 00691 * Set lorawan application EUI 00692 * equivalent to setNetworkId 00693 * @param eui application EUI (size 8) 00694 */ 00695 int32_t setAppEUI(const uint8_t* eui); 00696 00697 /** 00698 * Get lorawan application EUI 00699 * equivalent to getNetworkId 00700 * @returns vector containing application EUI (size 8) 00701 */ 00702 const uint8_t* getAppEUI(); 00703 00704 /** 00705 * Set lorawan application key 00706 * equivalent to setNetworkKey 00707 * @param eui application key (size 16) 00708 */ 00709 int32_t setAppKey(const uint8_t* key); 00710 00711 /** 00712 * Set lorawan application key 00713 * equivalent to getNetworkKey 00714 * @returns eui application key (size 16) 00715 */ 00716 const uint8_t* getAppKey(); 00717 00718 /** 00719 * Set device nonce counter incremented with each Join Request 00720 * @returns MDOT_OK if success 00721 */ 00722 int32_t setDevNonce(const uint16_t& nonce); 00723 00724 /** 00725 * Get device nonce counter incremented with each Join Request 00726 * @returns join nonce 00727 */ 00728 uint16_t getDevNonce(); 00729 00730 /** 00731 * Set app nonce counter incremented with each Join Accept 00732 * @returns MDOT_OK if success 00733 */ 00734 int32_t setAppNonce(const uint32_t& nonce); 00735 00736 /** 00737 * Get app nonce counter incremented with each Join Accept 00738 * @returns app nonce 00739 */ 00740 uint32_t getAppNonce(); 00741 00742 /** 00743 * Enable/disable Join Nonce validation for App Nonce in Join Accept 00744 * Default is enabled 00745 */ 00746 int32_t setJoinNonceValidation(bool enable); 00747 bool getJoinNonceValidation(); 00748 00749 /** 00750 * Add a multicast session address and keys 00751 * Downlink counter is set to 0 00752 * Up to 8 MULTICAST_SESSIONS can be set 00753 */ 00754 int32_t setMulticastSession(uint8_t index, uint32_t addr, const uint8_t* nsk, const uint8_t* ask); 00755 00756 /** 00757 * Set multicast session info 00758 * Up to 8 MULTICAST_SESSIONS 00759 */ 00760 int32_t setMulticastAddress(uint8_t index, uint32_t addr); 00761 int32_t setMulticastNetworkSessionKey(uint8_t index, const uint8_t* nsk); 00762 int32_t setMulticastApplicationSessionKey(uint8_t index, const uint8_t* ask); 00763 00764 /** 00765 * Set a multicast session counter 00766 * Up to 8 MULTICAST_SESSIONS 00767 */ 00768 int32_t setMulticastDownlinkCounter(uint8_t index, uint32_t count); 00769 int32_t setMulticastPeriodicity(uint8_t index, int8_t period); 00770 int32_t setMulticastFrequency(uint8_t index, uint32_t freq); 00771 int32_t setMulticastDatarate(uint8_t index, uint8_t dr); 00772 00773 /** 00774 * Get multicast session info 00775 * Up to 8 MULTICAST_SESSIONS 00776 */ 00777 uint32_t getMulticastAddress(uint8_t index); 00778 int32_t getMulticastNetworkSessionKey(uint8_t index, uint8_t* nsk); 00779 int32_t getMulticastApplicationSessionKey(uint8_t index, uint8_t* ask); 00780 uint32_t getMulticastDownlinkCounter(uint8_t index); 00781 int8_t getMulticastPeriodicity(uint8_t index); 00782 uint32_t getMulticastFrequency(uint8_t index); 00783 uint8_t getMulticastDatarate(uint8_t index); 00784 00785 /** 00786 * Attempt to join network 00787 * each attempt will be made with a random datarate up to the configured datarate 00788 * JoinRequest backoff between tries is enforced to 1% for 1st hour, 0.1% for 1-10 hours and 0.01% after 10 hours 00789 * Check getNextTxMs() for time until next join attempt can be made 00790 * @returns MDOT_OK if success 00791 */ 00792 int32_t joinNetwork(); 00793 00794 /** 00795 * Attempts to join network once 00796 * @returns MDOT_OK if success 00797 */ 00798 int32_t joinNetworkOnce(); 00799 00800 /** 00801 * Resets current network session, essentially disconnecting from the network 00802 * has no effect for MANUAL network join mode 00803 */ 00804 void resetNetworkSession(); 00805 00806 /** 00807 * Restore saved network session from flash 00808 * has no effect for MANUAL network join mode 00809 */ 00810 void restoreNetworkSession(); 00811 00812 /** 00813 * Save current network session to flash 00814 * has no effect for MANUAL network join mode 00815 */ 00816 void saveNetworkSession(); 00817 00818 /** 00819 * Set number of times joining will retry each sub-band before changing 00820 * to the next subband in US915 and AU915 00821 * @param retries must be between 0 - 255 00822 * @returns MDOT_OK if success 00823 */ 00824 int32_t setJoinRetries(const uint8_t& retries); 00825 00826 /** 00827 * Get number of times joining will retry each sub-band 00828 * @returns join retries (0 - 255) 00829 */ 00830 uint8_t getJoinRetries(); 00831 00832 /** 00833 * Set network join mode 00834 * MANUAL: set network address and session keys manually 00835 * OTA: User sets network name and passphrase, then attempts to join 00836 * AUTO_OTA: same as OTA, but network sessions can be saved and restored 00837 * @param mode MANUAL, OTA, or AUTO_OTA 00838 * @returns MDOT_OK if success 00839 */ 00840 int32_t setJoinMode(const uint8_t& mode); 00841 00842 /** 00843 * Get network join mode 00844 * @returns MANUAL, OTA, or AUTO_OTA 00845 */ 00846 uint8_t getJoinMode(); 00847 00848 /** 00849 * Get network join status 00850 * @returns true if currently joined to network 00851 */ 00852 bool getNetworkJoinStatus(); 00853 00854 /** 00855 * Do a network link check 00856 * application data may be returned in response to a network link check command 00857 * @returns link_check structure containing success, dBm above noise floor, gateways in range, and packet payload 00858 */ 00859 link_check networkLinkCheck(); 00860 00861 /** 00862 * Set network link check count to perform automatic link checks every count packets 00863 * only applicable if ACKs are disabled 00864 * @param count must be between 0 - 255 00865 * @returns MDOT_OK if success 00866 */ 00867 int32_t setLinkCheckCount(const uint8_t& count); 00868 00869 /** 00870 * Get network link check count 00871 * @returns count (0 - 255) 00872 */ 00873 uint8_t getLinkCheckCount(); 00874 00875 /** 00876 * Set network link check threshold, number of link check failures or missed acks to tolerate 00877 * before considering network connection lost 00878 * @pararm count must be between 0 - 255 00879 * @returns MDOT_OK if success 00880 */ 00881 int32_t setLinkCheckThreshold(const uint8_t& count); 00882 00883 /** 00884 * Get network link check threshold 00885 * @returns threshold (0 - 255) 00886 */ 00887 uint8_t getLinkCheckThreshold(); 00888 00889 /** 00890 * Get/set number of failed link checks in the current session 00891 * @returns count (0 - 255) 00892 */ 00893 uint8_t getLinkFailCount(); 00894 int32_t setLinkFailCount(uint8_t count); 00895 00896 /** 00897 * Set UpLinkCounter number of packets sent to the gateway during this network session (sequence number) 00898 * @returns MDOT_OK 00899 */ 00900 int32_t setUpLinkCounter(uint32_t count); 00901 00902 /** 00903 * Get UpLinkCounter 00904 * @returns number of packets sent to the gateway during this network session (sequence number) 00905 */ 00906 uint32_t getUpLinkCounter(); 00907 00908 /** 00909 * Set UpLinkCounter number of packets sent by the gateway during this network session (sequence number) 00910 * @returns MDOT_OK 00911 */ 00912 int32_t setDownLinkCounter(uint32_t count); 00913 00914 /** 00915 * Get DownLinkCounter 00916 * @returns number of packets sent by the gateway during this network session (sequence number) 00917 */ 00918 uint32_t getDownLinkCounter(); 00919 00920 /** 00921 * Enable/disable AES encryption 00922 * AES encryption must be enabled for use with Conduit gateway and MTAC_LORA card 00923 * @param on true for AES encryption to be enabled 00924 * @returns MDOT_OK if success 00925 */ 00926 int32_t setAesEncryption(const bool& on); 00927 00928 /** 00929 * Get AES encryption 00930 * @returns true if AES encryption is enabled 00931 */ 00932 bool getAesEncryption(); 00933 00934 /** 00935 * Get RSSI stats 00936 * @returns rssi_stats struct containing last, min, max, and avg RSSI in dB 00937 */ 00938 rssi_stats getRssiStats(); 00939 00940 /** 00941 * Get SNR stats 00942 * @returns snr_stats struct containing last, min, max, and avg SNR in cB 00943 */ 00944 snr_stats getSnrStats(); 00945 00946 /** 00947 * Get ms until next free channel 00948 * only applicable for European models, US models return 0 00949 * @returns time (ms) until a channel is free to use for transmitting 00950 */ 00951 uint32_t getNextTxMs(); 00952 00953 /** 00954 * Get available bytes for payload 00955 * @returns bytes 00956 */ 00957 uint8_t getNextTxMaxSize(); 00958 00959 /** 00960 * Get join delay in seconds 00961 * Defaults to 5 seconds 00962 * Must match join delay setting of the network server 00963 * 00964 * The default Join Delay is 5 seconds 00965 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00966 * 00967 * @returns number of seconds before join accept message is expected 00968 */ 00969 uint8_t getJoinDelay(); 00970 00971 /** 00972 * Set join delay in seconds 00973 * Defaults to 5 seconds 00974 * Must match join delay setting of the network server 00975 * 00976 * The default Join Delay is 5 seconds 00977 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00978 * 00979 * @param delay number of seconds before join accept message is expected 00980 * @return MDOT_OK if success 00981 */ 00982 uint32_t setJoinDelay(uint8_t delay); 00983 00984 /** 00985 * Get join Rx1 datarate offset 00986 * defaults to 0 00987 * @returns offset 00988 */ 00989 uint8_t getJoinRx1DataRateOffset(); 00990 00991 /** 00992 * Set join Rx1 datarate offset 00993 * @param offset for datarate 00994 * @return MDOT_OK if success 00995 */ 00996 uint32_t setJoinRx1DataRateOffset(uint8_t offset); 00997 00998 /** 00999 * Get join Rx2 datarate 01000 * defaults to US:DR8, AU:DR8, EU:DR0 01001 * @returns datarate 01002 */ 01003 uint8_t getJoinRx2DataRate(); 01004 01005 /** 01006 * Set join Rx2 datarate 01007 * @param datarate 01008 * @return MDOT_OK if success 01009 */ 01010 uint32_t setJoinRx2DataRate(uint8_t datarate); 01011 01012 /** 01013 * Get join Rx2 frequency 01014 * defaults US:923.3, AU:923.3, EU:869.525 01015 * @returns frequency 01016 */ 01017 uint32_t getJoinRx2Frequency(); 01018 01019 /** 01020 * Set join Rx2 frequency 01021 * @param frequency 01022 * @return MDOT_OK if success 01023 */ 01024 uint32_t setJoinRx2Frequency(uint32_t frequency); 01025 01026 /** 01027 * Get rx delay in seconds 01028 * Defaults to 1 second 01029 * @returns number of seconds before response message is expected 01030 */ 01031 uint8_t getRxDelay(); 01032 01033 /** 01034 * Set rx delay in seconds 01035 * Defaults to 1 second 01036 * @param delay number of seconds before response message is expected 01037 * @return MDOT_OK if success 01038 */ 01039 uint32_t setRxDelay(uint8_t delay); 01040 01041 /** 01042 * Get preserve session to save network session info through reset or power down in AUTO_OTA mode 01043 * Defaults to off 01044 * @returns true if enabled 01045 */ 01046 bool getPreserveSession(); 01047 01048 /** 01049 * Set preserve session to save network session info through reset or power down in AUTO_OTA mode 01050 * Defaults to off 01051 * @param enable 01052 * @return MDOT_OK if success 01053 */ 01054 uint32_t setPreserveSession(bool enable); 01055 01056 /** 01057 * Get data pending 01058 * only valid after sending data to the gateway 01059 * @returns true if server has available packet(s) 01060 */ 01061 bool getDataPending(); 01062 01063 /** 01064 * Get ack requested 01065 * only valid after sending data to the gateway 01066 * @returns true if server has requested ack 01067 */ 01068 bool getAckRequested(); 01069 01070 /** 01071 * Get is transmitting indicator 01072 * @returns true if currently transmitting 01073 */ 01074 bool getIsTransmitting(); 01075 01076 /** 01077 * Get is idle indicator 01078 * @returns true if not currently transmitting, waiting or receiving 01079 */ 01080 bool getIsIdle(); 01081 01082 /** 01083 * Set TX data rate 01084 * data rates affect maximum payload size 01085 * @param dr DR0-DR7 for Europe, DR0-DR4 for United States 01086 * @returns MDOT_OK if success 01087 */ 01088 int32_t setTxDataRate(const uint8_t& dr); 01089 01090 /** 01091 * Get TX data rate 01092 * @returns current TX data rate (DR0-DR15) 01093 */ 01094 uint8_t getTxDataRate(); 01095 01096 /** 01097 * Get a random value from the radio based on RSSI 01098 * @returns randome value 01099 */ 01100 uint32_t getRadioRandom(); 01101 01102 /** 01103 * Get data rate spreading factor and bandwidth 01104 * EU868 Datarates 01105 * --------------- 01106 * DR0 - SF12BW125 01107 * DR1 - SF11BW125 01108 * DR2 - SF10BW125 01109 * DR3 - SF9BW125 01110 * DR4 - SF8BW125 01111 * DR5 - SF7BW125 01112 * DR6 - SF7BW250 01113 * DR7 - FSK 01114 * 01115 * US915 Datarates 01116 * --------------- 01117 * DR0 - SF10BW125 01118 * DR1 - SF9BW125 01119 * DR2 - SF8BW125 01120 * DR3 - SF7BW125 01121 * DR4 - SF8BW500 01122 * 01123 * AU915 Datarates 01124 * --------------- 01125 * DR0 - SF12BW125 01126 * DR1 - SF11BW125 01127 * DR2 - SF10BW125 01128 * DR3 - SF9BW125 01129 * DR4 - SF8BW125 01130 * DR5 - SF7BW125 01131 * DR6 - SF8BW500 01132 * 01133 * @returns spreading factor and bandwidth 01134 */ 01135 std::string getDataRateDetails(uint8_t rate); 01136 01137 /** 01138 * Set TX power output of radio before antenna gain, default: 14 dBm 01139 * actual output power may be limited by local regulations for the chosen frequency 01140 * power affects maximum range 01141 * @param power 2 dBm - 20 dBm 01142 * @returns MDOT_OK if success 01143 */ 01144 int32_t setTxPower(const uint32_t& power); 01145 01146 /** 01147 * Get TX power 01148 * @returns TX power (2 dBm - 20 dBm) 01149 */ 01150 uint32_t getTxPower(); 01151 01152 /** 01153 * Get configured gain of installed antenna, default: +3 dBi 01154 * @returns gain of antenna in dBi 01155 */ 01156 int8_t getAntennaGain(); 01157 01158 /** 01159 * Set configured gain of installed antenna, default: +3 dBi 01160 * @param gain -127 dBi - 128 dBi 01161 * @returns MDOT_OK if success 01162 */ 01163 int32_t setAntennaGain(int8_t gain); 01164 01165 /** 01166 * Enable/disable TX waiting for rx windows 01167 * when enabled, send calls will block until a packet is received or RX timeout 01168 * @param enable set to true if expecting responses to transmitted packets 01169 * @returns MDOT_OK if success 01170 */ 01171 int32_t setTxWait(const bool& enable); 01172 01173 /** 01174 * Get TX wait 01175 * @returns true if TX wait is enabled 01176 */ 01177 bool getTxWait(); 01178 01179 /** 01180 * Cancel pending rx windows 01181 */ 01182 void cancelRxWindow(); 01183 01184 /** 01185 * Get time on air 01186 * @returns the amount of time (in ms) it would take to send bytes bytes based on current configuration 01187 */ 01188 uint32_t getTimeOnAir(uint8_t bytes); 01189 01190 /** 01191 * Get min frequency 01192 * @returns minimum frequency based on current channel plan 01193 */ 01194 uint32_t getMinFrequency(); 01195 01196 /** 01197 * Get max frequency 01198 * @returns maximum frequency based on current channel plan 01199 */ 01200 uint32_t getMaxFrequency(); 01201 01202 /** 01203 * Get min datarate 01204 * @returns minimum datarate based on current channel plan 01205 */ 01206 uint8_t getMinDatarate(); 01207 01208 /** 01209 * Get max datarate 01210 * @returns maximum datarate based on current channel plan 01211 */ 01212 uint8_t getMaxDatarate(); 01213 01214 /** 01215 * Get min datarate offset 01216 * @returns minimum datarate offset based on current channel plan 01217 */ 01218 uint8_t getMinDatarateOffset(); 01219 01220 /** 01221 * Get max datarate offset 01222 * @returns maximum datarate based on current channel plan 01223 */ 01224 uint8_t getMaxDatarateOffset(); 01225 01226 /** 01227 * Get min datarate 01228 * @returns minimum datarate based on current channel plan 01229 */ 01230 uint8_t getMinRx2Datarate(); 01231 01232 /** 01233 * Get max rx2 datarate 01234 * @returns maximum rx2 datarate based on current channel plan 01235 */ 01236 uint8_t getMaxRx2Datarate(); 01237 01238 /** 01239 * Get max tx power 01240 * @returns maximum tx power based on current channel plan 01241 */ 01242 uint8_t getMaxTxPower(); 01243 01244 /** 01245 * Get min tx power 01246 * @returns minimum tx power based on current channel plan 01247 */ 01248 uint8_t getMinTxPower(); 01249 01250 /** 01251 * Set ping slot periodicity 01252 * Specify the the number of ping slots in a given beacon interval 01253 * Note: Must switch back to class A for the change to take effect 01254 * @param exp - number_of_pings = 2^(7 - exp) where 0 <= exp <= 7 01255 * @returns MDOT_OK if success 01256 */ 01257 uint32_t setPingPeriodicity(uint8_t exp); 01258 01259 /** 01260 * Get ping slot periodicity 01261 * @returns exp = 7 - log2(number_of_pings) 01262 */ 01263 uint8_t getPingPeriodicity(); 01264 01265 /** 01266 * 01267 * get/set adaptive data rate 01268 * configure data rates and power levels based on signal to noise of packets received at gateway 01269 * true == adaptive data rate is on 01270 * set function returns MDOT_OK if success 01271 */ 01272 int32_t setAdr(const bool& on); 01273 bool getAdr(); 01274 01275 /** 01276 * Set the ADR ACK Limit 01277 * @param limit - ADR ACK limit 01278 * @returns MDOT_OK if success 01279 */ 01280 int32_t setAdrAckLimit(uint8_t limit); 01281 01282 /** 01283 * Get the ADR ACK Limit 01284 * @returns ADR ACK limit 01285 */ 01286 uint8_t getAdrAckLimit(); 01287 01288 /** 01289 * Set the ADR ACK Delay 01290 * @param delay - ADR ACK delay 01291 * @returns MDOT_OK if success 01292 */ 01293 int32_t setAdrAckDelay(uint8_t delay); 01294 01295 /** 01296 * Get the ADR ACK Delay 01297 * @returns ADR ACK delay 01298 */ 01299 uint8_t getAdrAckDelay(); 01300 01301 /** 01302 * Enable/disable CRC checking of packets 01303 * CRC checking must be enabled for use with Conduit gateway and MTAC_LORA card 01304 * @param on set to true to enable CRC checking 01305 * @returns MDOT_OK if success 01306 */ 01307 int32_t setCrc(const bool& on); 01308 01309 /** 01310 * Get CRC checking 01311 * @returns true if CRC checking is enabled 01312 */ 01313 bool getCrc(); 01314 01315 /** 01316 * Set ack 01317 * @param retries 0 to disable acks, otherwise 1 - 8 01318 * @returns MDOT_OK if success 01319 */ 01320 int32_t setAck(const uint8_t& retries); 01321 01322 /** 01323 * Get ack 01324 * @returns 0 if acks are disabled, otherwise retries (1 - 8) 01325 */ 01326 uint8_t getAck(); 01327 01328 /** 01329 * Set number of packet repeats for unconfirmed frames 01330 * @param repeat 0 or 1 for no repeats, otherwise 2-15 01331 * @returns MDOT_OK if success 01332 */ 01333 int32_t setRepeat(const uint8_t& repeat); 01334 01335 /** 01336 * Get number of packet repeats for unconfirmed frames 01337 * @returns 0 or 1 if no repeats, otherwise 2-15 01338 */ 01339 uint8_t getRepeat(); 01340 01341 /** 01342 * Send data to the gateway 01343 * validates data size (based on spreading factor) 01344 * @param data a vector of up to 242 bytes (may be less based on spreading factor) 01345 * @returns MDOT_OK if packet was sent successfully (ACKs disabled), or if an ACK was received (ACKs enabled) 01346 */ 01347 int32_t send(const std::vector<uint8_t>& data, const bool& blocking = true, const bool& highBw = false); 01348 01349 /** 01350 * Inject mac command 01351 * @param data a vector containing mac commands 01352 * @returns MDOT_OK 01353 */ 01354 int32_t injectMacCommand(const std::vector<uint8_t>& data); 01355 01356 /** 01357 * Clear MAC command buffer to be sent in next uplink 01358 * @returns MDOT_OK 01359 */ 01360 int32_t clearMacCommands(); 01361 01362 /** 01363 * Get MAC command buffer to be sent in next uplink 01364 * @returns command bytes 01365 */ 01366 std::vector<uint8_t> getMacCommands(); 01367 01368 /** 01369 * Fetch data received from the gateway 01370 * this function only checks to see if a packet has been received - it does not open a receive window 01371 * send() must be called before recv() 01372 * @param data a vector to put the received data into 01373 * @returns MDOT_OK if packet was successfully received 01374 */ 01375 int32_t recv(std::vector<uint8_t>& data); 01376 01377 /** 01378 * Ping 01379 * status will be MDOT_OK if ping succeeded 01380 * @returns ping_response struct containing status, RSSI, and SNR 01381 */ 01382 ping_response ping(); 01383 01384 /** 01385 * Get return code string 01386 * @returns string containing a description of the given error code 01387 */ 01388 static std::string getReturnCodeString(const int32_t& code); 01389 01390 /** 01391 * Get last error 01392 * @returns string explaining the last error that occured 01393 */ 01394 std::string getLastError(); 01395 01396 /** 01397 * Go to sleep 01398 * @param interval the number of seconds to sleep before waking up if wakeup_mode == RTC_ALARM or RTC_ALARM_OR_INTERRUPT, else ignored 01399 * @param wakeup_mode RTC_ALARM, INTERRUPT, RTC_ALARM_OR_INTERRUPT 01400 * if RTC_ALARM the real time clock is configured to wake the device up after the specified interval 01401 * if INTERRUPT the device will wake up on the rising edge of the interrupt pin 01402 * if RTC_ALARM_OR_INTERRUPT the device will wake on the first event to occur 01403 * @param deepsleep if true go into deep sleep mode (lowest power, all memory and registers are lost, peripherals turned off) 01404 * else go into sleep mode (low power, memory and registers are maintained, peripherals stay on) 01405 * 01406 * For the MDOT 01407 * in sleep mode, the device can be woken up on an XBEE_DI (2-8) pin or by the RTC alarm 01408 * in deepsleep mode, the device can only be woken up using the WKUP pin (PA0, XBEE_DIO7) or by the RTC alarm 01409 * For the XDOT 01410 * in sleep mode, the device can be woken up on GPIO (0-3), UART1_RX, WAKE or by the RTC alarm 01411 * in deepsleep mode, the device can only be woken up using the WKUP pin (PA0, WAKE) or by the RTC alarm 01412 * @returns MDOT_OK on success 01413 */ 01414 int32_t sleep(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM, const bool& deepsleep = true); 01415 01416 /** 01417 * Set auto sleep mode 01418 * Auto sleep mode will automatically put the MCU to sleep after tx and in between receive windows 01419 * Note: The MCU will go into a stop mode sleep in between rx windows. This means that 01420 * peripherals such as timers will not function during the sleep intervals. 01421 * @param enable - Flag to enable auto sleep mode 01422 */ 01423 void setAutoSleep(bool enable); 01424 01425 /** 01426 * Get auto sleep mode 01427 * @returns 0 if sleep mode is disabled, 1 if it is enabled 01428 */ 01429 uint8_t getAutoSleep(); 01430 01431 /** 01432 * Set wake pin 01433 * @param pin the pin to use to wake the device from sleep mode 01434 * For MDOT, XBEE_DI (2-8) 01435 * For XDOT, GPIO (0-3), UART1_RX, or WAKE 01436 */ 01437 void setWakePin(const PinName& pin); 01438 01439 /** 01440 * Get wake pin 01441 * @returns the pin to use to wake the device from sleep mode 01442 * For MDOT, XBEE_DI (2-8) 01443 * For XDOT, GPIO (0-3), UART1_RX, or WAKE 01444 */ 01445 PinName getWakePin(); 01446 01447 /** 01448 * Write data in a user backup register 01449 * @param register one of UBR0 through UBR9 for MDOT, one of UBR0 through UBR21 for XDOT 01450 * @param data user data to back up 01451 * @returns true if success 01452 */ 01453 bool writeUserBackupRegister(uint32_t reg, uint32_t data); 01454 01455 /** 01456 * Read data in a user backup register 01457 * @param register one of UBR0 through UBR9 for MDOT, one of UBR0 through UBR21 for XDOT 01458 * @param data gets set to content of register 01459 * @returns true if success 01460 */ 01461 bool readUserBackupRegister(uint32_t reg, uint32_t& data); 01462 01463 /** 01464 * Set LBT time in us 01465 * @param ms time in us 01466 * @returns true if success 01467 */ 01468 bool setLbtTimeUs(uint16_t us); 01469 01470 /** 01471 * Get LBT time in us 01472 * @returns LBT time in us 01473 */ 01474 uint16_t getLbtTimeUs(); 01475 01476 /** 01477 * Set LBT threshold in dBm 01478 * @param rssi threshold in dBm 01479 * @returns true if success 01480 */ 01481 bool setLbtThreshold(int8_t rssi); 01482 01483 /** 01484 * Get LBT threshold in dBm 01485 * @returns LBT threshold in dBm 01486 */ 01487 int8_t getLbtThreshold(); 01488 01489 /** 01490 * Get Radio Frequency Offset 01491 * Used for fine calibration of radio frequencies 01492 * @returns frequency offset in MHz 01493 */ 01494 int32_t getFrequencyOffset(); 01495 /** 01496 * Get Radio Frequency Offset 01497 * Used for fine calibration of radio frequencies 01498 * @param offset frequency offset in MHz 01499 */ 01500 void setFrequencyOffset(int32_t offset); 01501 01502 /** 01503 * Get GPS time from network server 01504 * Sends a DeviceTimeReq command to the network server 01505 * @returns time since GPS epoch, 0 on failure 01506 */ 01507 uint64_t getGPSTime(); 01508 01509 /** 01510 * Add a device time request to the MacCommand buffer 01511 */ 01512 void addDeviceTimeRequest(); 01513 01514 #if defined(TARGET_MTS_MDOT_F411RE) 01515 /////////////////////////////////////////////////////////////////// 01516 // Filesystem (Non Volatile Memory) Operation Functions for mDot // 01517 /////////////////////////////////////////////////////////////////// 01518 01519 // Save user file data to flash 01520 // file - name of file max 30 chars 01521 // data - data of file 01522 // size - size of file 01523 // returns true if successful 01524 bool saveUserFile(const char* file, void* data, uint32_t size); 01525 01526 // Append user file data to flash 01527 // file - name of file max 30 chars 01528 // data - data of file 01529 // size - size of file 01530 // returns true if successful 01531 bool appendUserFile(const char* file, void* data, uint32_t size); 01532 01533 // Read user file data from flash 01534 // file - name of file max 30 chars 01535 // data - data of file 01536 // size - size of file 01537 // returns true if successful 01538 bool readUserFile(const char* file, void* data, uint32_t size); 01539 01540 // Move a user file in flash 01541 // file - name of file 01542 // new_name - new name of file 01543 // returns true if successful 01544 bool moveUserFile(const char* file, const char* new_name); 01545 01546 // Delete user file data from flash 01547 // file - name of file max 30 chars 01548 // returns true if successful 01549 bool deleteUserFile(const char* file); 01550 01551 // Open user file in flash, max of 4 files open concurrently 01552 // file - name of file max 30 chars 01553 // mode - combination of FM_APPEND | FM_TRUNC | FM_CREAT | 01554 // FM_RDONLY | FM_WRONLY | FM_RDWR | FM_DIRECT 01555 // returns - mdot_file struct, fd field will be a negative value if file could not be opened 01556 mDot::mdot_file openUserFile(const char* file, int mode); 01557 01558 // Seek an open file 01559 // file - mdot file struct 01560 // offset - offset in bytes 01561 // whence - where offset is based SEEK_SET, SEEK_CUR, SEEK_END 01562 // returns true if successful 01563 bool seekUserFile(mDot::mdot_file& file, size_t offset, int whence); 01564 01565 // Read bytes from open file 01566 // file - mdot file struct 01567 // data - mem location to store data 01568 // length - number of bytes to read 01569 // returns - number of bytes read, negative if error 01570 int readUserFile(mDot::mdot_file& file, void* data, size_t length); 01571 01572 // Write bytes to open file 01573 // file - mdot file struct 01574 // data - data to write 01575 // length - number of bytes to write 01576 // returns - number of bytes written, negative if error 01577 int writeUserFile(mDot::mdot_file& file, void* data, size_t length); 01578 01579 // Close open file 01580 // file - mdot file struct 01581 // returns true if successful 01582 bool closeUserFile(mDot::mdot_file& file); 01583 01584 // List user files stored in flash 01585 std::vector<mDot::mdot_file> listUserFiles(); 01586 01587 // Move file into the firmware upgrade path to be flashed on next boot 01588 // file - name of file 01589 // returns true if successful 01590 bool moveUserFileToFirmwareUpgrade(const char* file); 01591 01592 // Return total size of all files saved in FLASH 01593 // Does not include SPIFFS overhead 01594 uint32_t getUsedSpace(); 01595 01596 bool repairFlashFileSystem(); 01597 #else 01598 /////////////////////////////////////////////////////////////// 01599 // EEPROM (Non Volatile Memory) Operation Functions for xDot // 01600 /////////////////////////////////////////////////////////////// 01601 01602 // Write to EEPROM 01603 // addr - address to write to (0 - 0x17FF) 01604 // data - data to write 01605 // size - size of data 01606 // returns true if successful 01607 bool nvmWrite(uint16_t addr, void* data, uint16_t size); 01608 01609 // Read from EEPROM 01610 // addr - address to read from (0 - 0x17FF) 01611 // data - buffer for data 01612 // size - size of buffer 01613 // returns true if successful 01614 bool nvmRead(uint16_t addr, void* data, uint16_t size); 01615 #endif /* TARGET_MTS_MDOT_F411RE */ 01616 01617 // get current statistics 01618 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01619 mdot_stats getStats(); 01620 01621 // reset statistics 01622 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01623 void resetStats(); 01624 01625 // Convert pin number 2-8 to pin name DIO2-DI8 01626 static PinName pinNum2Name(uint8_t num); 01627 01628 // Convert pin name DIO2-DI8 to pin number 2-8 01629 static uint8_t pinName2Num(PinName name); 01630 01631 // Convert pin name DIO2-DI8 to string 01632 static std::string pinName2Str(PinName name); 01633 01634 uint64_t crc64(uint64_t crc, const unsigned char *s, uint64_t l); 01635 01636 /************************************************************************* 01637 * The following functions are only used by the AT command application and 01638 * should not be used by standard applications consuming the mDot library 01639 ************************************************************************/ 01640 01641 // set/get configured baud rate for command port 01642 // only for use in conjunction with AT interface 01643 // set function returns MDOT_OK if success 01644 int32_t setBaud(const uint32_t& baud); 01645 uint32_t getBaud(); 01646 01647 // set/get baud rate for debug port 01648 // set function returns MDOT_OK if success 01649 int32_t setDebugBaud(const uint32_t& baud); 01650 uint32_t getDebugBaud(); 01651 01652 // set/get command terminal echo 01653 // set function returns MDOT_OK if success 01654 int32_t setEcho(const bool& on); 01655 bool getEcho(); 01656 01657 // set/get command terminal verbose mode 01658 // set function returns MDOT_OK if success 01659 int32_t setVerbose(const bool& on); 01660 bool getVerbose(); 01661 01662 // set/get startup mode 01663 // COMMAND_MODE (default), starts up ready to accept AT commands 01664 // SERIAL_MODE, read serial data and send it as LoRa packets 01665 // set function returns MDOT_OK if success 01666 int32_t setStartUpMode(const uint8_t& mode); 01667 uint8_t getStartUpMode(); 01668 01669 int32_t setRxDataRate(const uint8_t& dr); 01670 uint8_t getRxDataRate(); 01671 01672 // get/set TX/RX frequency 01673 // if set to 0, device will hop frequencies 01674 // set function returns MDOT_OK if success 01675 int32_t setTxFrequency(const uint32_t& freq); 01676 uint32_t getTxFrequency(); 01677 int32_t setRxFrequency(const uint32_t& freq); 01678 uint32_t getRxFrequency(); 01679 01680 // get/set RX output mode 01681 // valid options are HEXADECIMAL, BINARY, and EXTENDED 01682 // set function returns MDOT_OK if success 01683 int32_t setRxOutput(const uint8_t& mode); 01684 uint8_t getRxOutput(); 01685 01686 // get/set serial wake interval 01687 // valid values are 2 s - INT_MAX (2147483647) s 01688 // set function returns MDOT_OK if success 01689 int32_t setWakeInterval(const uint32_t& interval); 01690 uint32_t getWakeInterval(); 01691 01692 // get/set serial wake delay 01693 // valid values are 2 ms - INT_MAX (2147483647) ms 01694 // set function returns MDOT_OK if success 01695 int32_t setWakeDelay(const uint32_t& delay); 01696 uint32_t getWakeDelay(); 01697 01698 // get/set serial receive timeout 01699 // valid values are 0 ms - 65000 ms 01700 // set function returns MDOT_OK if success 01701 int32_t setWakeTimeout(const uint16_t& timeout); 01702 uint16_t getWakeTimeout(); 01703 01704 // get/set serial wake mode 01705 // valid values are INTERRUPT or RTC_ALARM 01706 // set function returns MDOT_OK if success 01707 int32_t setWakeMode(const uint8_t& delay); 01708 uint8_t getWakeMode(); 01709 01710 // get/set serial flow control enabled 01711 // set function returns MDOT_OK if success 01712 int32_t setFlowControl(const bool& on); 01713 bool getFlowControl(); 01714 01715 // get/set serial clear on error 01716 // if enabled the data read from the serial port will be discarded if it cannot be sent or if the send fails 01717 // set function returns MDOT_OK if success 01718 int32_t setSerialClearOnError(const bool& on); 01719 bool getSerialClearOnError(); 01720 01721 // MTS_RADIO_DEBUG_COMMANDS 01722 01723 /** 01724 * Disable Duty cycle 01725 * enables or disables the duty cycle limitations 01726 * **** ONLY TO BE USED FOR TESTINGS PURPOSES **** 01727 * **** ALL DEPLOYABLE CODE MUST ADHERE TO LOCAL REGULATIONS **** 01728 * **** THIS SETTING WILL NOT BE SAVED TO CONFIGURATION ***** 01729 * @param val true to disable duty-cycle (default:false) 01730 */ 01731 int32_t setDisableDutyCycle(bool val); 01732 01733 /** 01734 * Disable Duty cycle 01735 * **** ONLY TO BE USED FOR TESTINGS PURPOSES **** 01736 * **** ALL DEPLOYABLE CODE MUST ADHERE TO LOCAL REGULATIONS **** 01737 * **** THIS SETTING WILL NOT BE SAVED TO CONFIGURATION ***** 01738 * @return true if duty-cycle is disabled (default:false) 01739 */ 01740 uint8_t getDisableDutyCycle(); 01741 01742 /** 01743 * LBT RSSI 01744 * @return the current RSSI on the configured frequency (SetTxFrequency) using configured LBT Time 01745 */ 01746 int16_t lbtRssi(); 01747 01748 void openRxWindow(uint32_t timeout, uint8_t bandwidth = 0); 01749 void closeRxWindow(); 01750 void sendContinuous(bool enable=true, uint32_t timeout=0, uint32_t frequency=0, int8_t txpower=-1); 01751 int32_t setDeviceId(const std::vector<uint8_t>& id); 01752 int32_t setProtectedAppEUI(const std::vector<uint8_t>& appEUI); 01753 int32_t setProtectedAppKey(const std::vector<uint8_t>& appKey); 01754 std::vector<uint8_t> getProtectedAppEUI(); 01755 std::vector<uint8_t> getProtectedAppKey(); 01756 int32_t setProtectedGenAppKey(const std::vector<uint8_t>& appKey); 01757 int32_t setDefaultFrequencyBand(const uint8_t& band); 01758 bool saveProtectedConfig(); 01759 // resets the radio/mac/link 01760 void resetRadio(); 01761 int32_t setRadioMode(const uint8_t& mode); 01762 std::map<uint8_t, uint8_t> dumpRegisters(); 01763 void eraseFlash(); 01764 01765 void setWakeupCallback(void (*function)(void)); 01766 01767 template<typename T> 01768 void setWakeupCallback(T *object, void (T::*member)(void)) { 01769 _wakeup_callback.attach(object, member); 01770 } 01771 01772 lora::ChannelPlan* getChannelPlan(void); 01773 01774 uint32_t setRx2DataRate(uint8_t dr); 01775 uint8_t getRx2DataRate(); 01776 01777 void mcGroupKeys(uint8_t *mcKeyEncrypt, uint32_t addr, uint8_t groupId, uint32_t frame_count); 01778 private: 01779 01780 void sleep_ms(uint32_t interval, 01781 uint8_t wakeup_mode = RTC_ALARM, 01782 bool deepsleep = true); 01783 01784 01785 void wakeup(); 01786 01787 mdot_stats _stats; 01788 01789 Callback<void()> _wakeup_callback; 01790 01791 bool _standbyFlag; 01792 bool _testMode; 01793 uint8_t _savedPort; 01794 void handleTestModePacket(); 01795 lora::ChannelPlan* _plan; 01796 }; 01797 01798 #endif
Generated on Wed Jul 13 2022 04:34:59 by
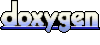